在 C# 中將字串轉換為雙精度
-
在 C# 中使用
Convert.ToDouble()
函式將字串轉換為雙精度數 -
在 C# 中使用
Double.Parse()
方法將字串轉換為雙精度數 -
在 C# 中使用
Double.TryParse()
方法測試字串並將其轉換為 Double
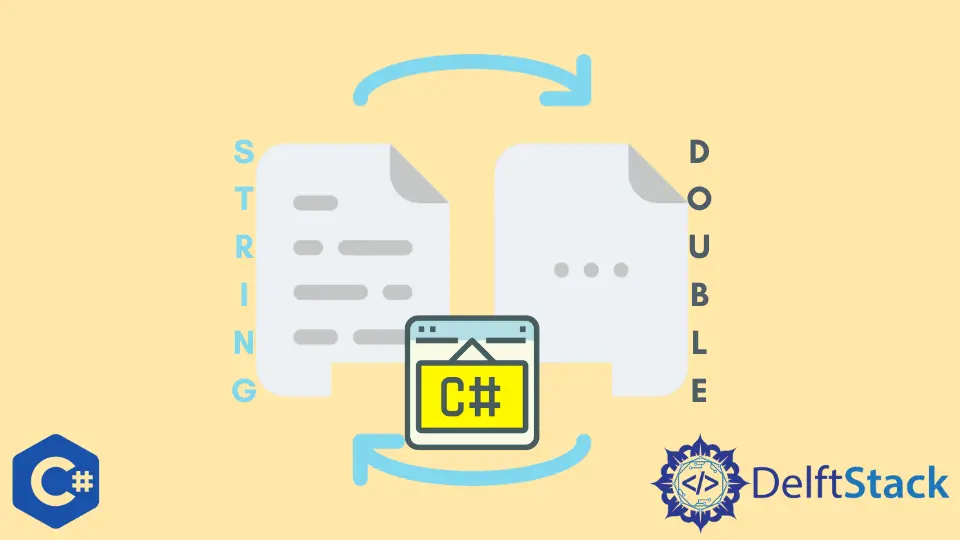
字串用於儲存文字,在 C# 中,字串不僅僅是文字片段。它們是可以具有多種不同操作的物件。
Double 是具有 15 位十進位制值的浮點數。它在 Float(另一種浮點資料型別)之後使用,但僅限於 7 個十進位制數字。
通常,字串可能包含文字中的數字,可能需要用作數字資料型別,例如: Int、Float、Double 等,用於算術運算。
本文將演示如何將字串轉換為雙精度數。
在 C# 中使用 Convert.ToDouble()
函式將字串轉換為雙精度數
我們在這裡宣告一個 String 變數,然後從 Console 類中呼叫 ToDouble()
函式將 String 型別轉換為 Double
。
String word = "123.987324234234";
Console.WriteLine(Convert.ToDouble(word));
如果上述轉換在極少數情況下產生錯誤,你可以嘗試將字串中的 .
改為 ,
。
例如,123.98732
將轉換為 123,98732
。但是,發生這種情況的機會很少,如果出現錯誤,你最好嘗試下面給出的不同選項。
在 C# 中使用 Double.Parse()
方法將字串轉換為雙精度數
該方法繼承自類 Double
,並作為 Console
類提供的方法的絕佳替代品。它解析字串中的數字並提供 Double 資料型別結果。
String word = "123.9873242342342342342342342341";
Double number = Double.Parse(word);
Console.WriteLine(number);
在 C# 中使用 Double.TryParse()
方法測試字串並將其轉換為 Double
Parse()
方法附帶一個類似的函式,稱為 TryParse()
。一種方法,它還允許檢查字串是否為有效的 Double,以及返回已解析的 Double 資料型別。
TryParse()
是一個布林方法,如果字串是有效的 Double 則返回 True
,否則返回 False
。它還接受通過引用傳遞的第二個引數,以使用從字串中解析的 Double 修改其值。
String word = "123.9873242342342342342342342341";
Double get_number;
if (Double.TryParse(word, out get_number)) {
Console.WriteLine("Valid Double. Number is : " + get_number);
} else {
Console.WriteLine("Invalid Double");
}
請記住始終使用 out
關鍵字並傳遞第二個引數。為什麼?
C# 中的 out
關鍵字通過引用而不是值傳遞引數,允許函式修改其值並測試其有效性。
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub