在 C# 上连接到 SQL 数据库
Fil Zjazel Romaeus Villegas
2023年10月12日
Csharp
Csharp SQL
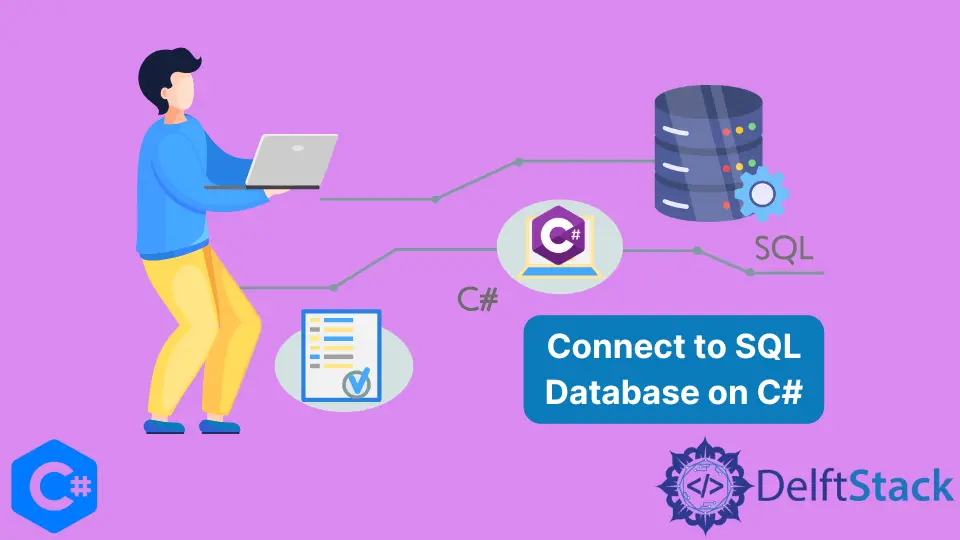
本教程将演示如何使用 SqlConnection
对象连接到 C# 上的 SQL 数据库。
使用 SqlConnection
对象连接到 C#
上的 SQL 数据库
SqlConnection
类是一个对象,表示与传递的连接字符串指定的 SQL Server 数据库的连接。它包含在命名空间 System.Data.SqlClient
中。
SqlConnection connection = new SqlConnection(connectionString);
连接字符串包含有关数据源、如何连接到它以及连接配置详细信息的信息。你可以在连接字符串中包含许多不同的参数,但我们将讨论一些最常用的参数。
Server
/Data Source
:保存数据库的服务器名称。Database
/Initial Catalog
:这是数据库的名称。Trusted Connection
/Integrated Security
:指定应用程序是否可以使用系统上任何可用的安全包。如果设置为 true,则不需要用户 ID 和密码参数。用户 ID
:连接的用户名。密码
:用于连接的密码。
将连接字符串传递给 SqlConnection
对象后,你可以使用其方法管理连接。
Open()
:打开连接。Close()
:关闭连接。Dispose()
:释放连接使用的资源。ChangeDatabase()
:为打开的SqlConnection
更改当前数据库。
例子:
using System;
using System.Data.SqlClient;
namespace SQLConnection_Sample {
class Program {
static void Main(string[] args) {
// The server's name that holds the database
string DataSource = "MSI\\SQLEXPRESS";
// The name of the database
string InitialCatalog = "SampleDB";
// Sets if the connection should use integrated security.
// If this value is set to "SSPI", the user's Windows Authentication will be used
string IntegratedSecurity = "SSPI";
// Should the database require a specific log in
string UserID = "";
string Password = "";
string connectionString = "Data Source =" + DataSource +
"; Initial Catalog =" + InitialCatalog +
"; Integrated Security=" + IntegratedSecurity
//+ "; User ID=" + UserID
//+ "; Password=" + Password
;
try {
SqlConnection connection = new SqlConnection(connectionString);
connection.Open();
Console.WriteLine("The database has been opened!");
Console.WriteLine("Connection State: " + connection.State.ToString());
connection.Close();
Console.WriteLine("The database has been closed!");
connection.Dispose();
Console.WriteLine("The database connection has been disposed!");
Console.WriteLine("Connection State: " + connection.State.ToString());
} catch (Exception ex) {
Console.WriteLine("There's an error connecting to the database!\n" + ex.Message);
}
Console.ReadLine();
}
}
}
在上面的示例中,我们首先通过输入服务器、数据库名称和集成的安全参数来创建连接字符串。将其传递给 SqlConnection
对象后,我们通过打开、关闭和最后释放连接来演示不同的状态。
所有这些都打印在控制台中。
输出:
The database has been opened!
Connection State: Open
The database has been closed!
The database connection has been disposed!
Connection State: Closed
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe