C#에서 SQL 데이터베이스에 연결
Fil Zjazel Romaeus Villegas
2023년10월12일
Csharp
Csharp SQL
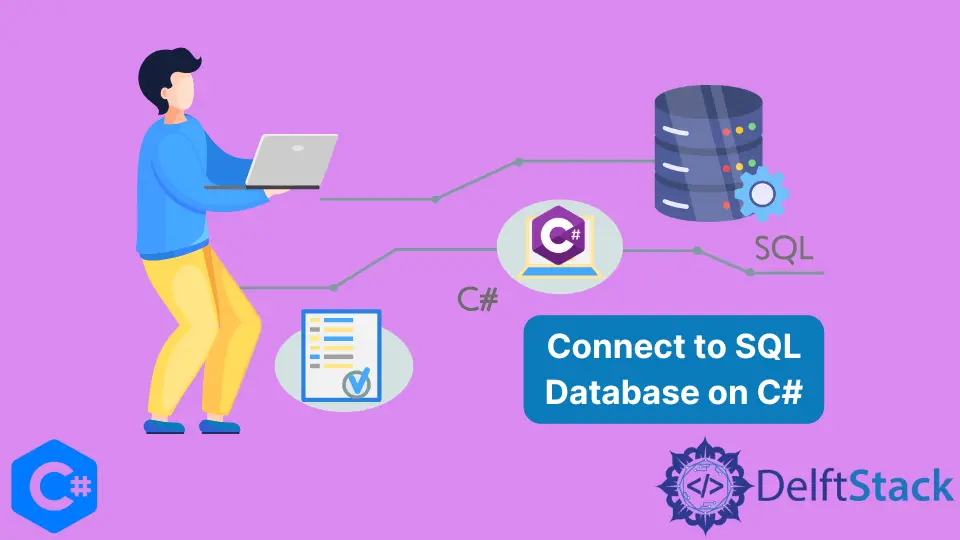
이 자습서에서는 SqlConnection
개체를 사용하여 C#에서 SQL 데이터베이스에 연결하는 방법을 보여줍니다.
SqlConnection
개체를 사용하여 C#
의 SQL 데이터베이스에 연결
SqlConnection
클래스는 전달된 연결 문자열에 지정된 대로 SQL Server 데이터베이스에 대한 연결을 나타내는 개체입니다. 네임스페이스 System.Data.SqlClient
에 포함되어 있습니다.
SqlConnection connection = new SqlConnection(connectionString);
연결 문자열에는 데이터 원본에 대한 정보, 연결 방법 및 연결 구성 세부 정보가 포함됩니다. 연결 문자열에 다양한 매개변수를 포함할 수 있지만 가장 일반적으로 사용되는 매개변수에 대해 설명합니다.
서버
/데이터 소스
: 데이터베이스를 보유하고 있는 서버의 이름입니다.Database
/ Initial Catalog
: 데이터베이스의 이름입니다.신뢰할 수 있는 연결
/통합 보안
: 응용 프로그램이 시스템에서 사용 가능한 보안 패키지를 사용할 수 있는지 여부를 지정합니다. true로 설정하면 사용자 ID 및 암호 매개변수가 필요하지 않습니다.User ID
: 연결을 위한 사용자 이름입니다.비밀번호
: 연결에 사용할 비밀번호입니다.
연결 문자열을 SqlConnection
개체에 전달하면 해당 메서드를 사용하여 연결을 관리할 수 있습니다.
Open()
: 연결을 엽니다.Close()
: 연결을 닫습니다.Dispose()
: 연결에서 사용하는 리소스를 해제합니다.ChangeDatabase()
: 열려 있는SqlConnection
에 대한 현재 데이터베이스를 변경합니다.
예시:
using System;
using System.Data.SqlClient;
namespace SQLConnection_Sample {
class Program {
static void Main(string[] args) {
// The server's name that holds the database
string DataSource = "MSI\\SQLEXPRESS";
// The name of the database
string InitialCatalog = "SampleDB";
// Sets if the connection should use integrated security.
// If this value is set to "SSPI", the user's Windows Authentication will be used
string IntegratedSecurity = "SSPI";
// Should the database require a specific log in
string UserID = "";
string Password = "";
string connectionString = "Data Source =" + DataSource +
"; Initial Catalog =" + InitialCatalog +
"; Integrated Security=" + IntegratedSecurity
//+ "; User ID=" + UserID
//+ "; Password=" + Password
;
try {
SqlConnection connection = new SqlConnection(connectionString);
connection.Open();
Console.WriteLine("The database has been opened!");
Console.WriteLine("Connection State: " + connection.State.ToString());
connection.Close();
Console.WriteLine("The database has been closed!");
connection.Dispose();
Console.WriteLine("The database connection has been disposed!");
Console.WriteLine("Connection State: " + connection.State.ToString());
} catch (Exception ex) {
Console.WriteLine("There's an error connecting to the database!\n" + ex.Message);
}
Console.ReadLine();
}
}
}
위의 예에서는 먼저 서버, 데이터베이스 이름, 통합 보안 매개변수를 입력하여 연결 문자열을 생성했습니다. SqlConnection
개체에 전달한 후 연결을 열고 닫고 최종적으로 삭제하여 다양한 상태를 시연했습니다.
이 모든 것이 콘솔에 인쇄됩니다.
출력:
The database has been opened!
Connection State: Open
The database has been closed!
The database connection has been disposed!
Connection State: Closed
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다