在 C++ 中查找字符串的长度
Jinku Hu
2023年10月12日
-
使用
length
函数在 C++ 中查找字符串的长度 -
使用
size
函数在 C++ 中查找字符串的长度 -
使用
while
循环在 C++ 中查找字符串的长度 -
使用
std::strlen
函数在 C++ 中查找字符串的长度
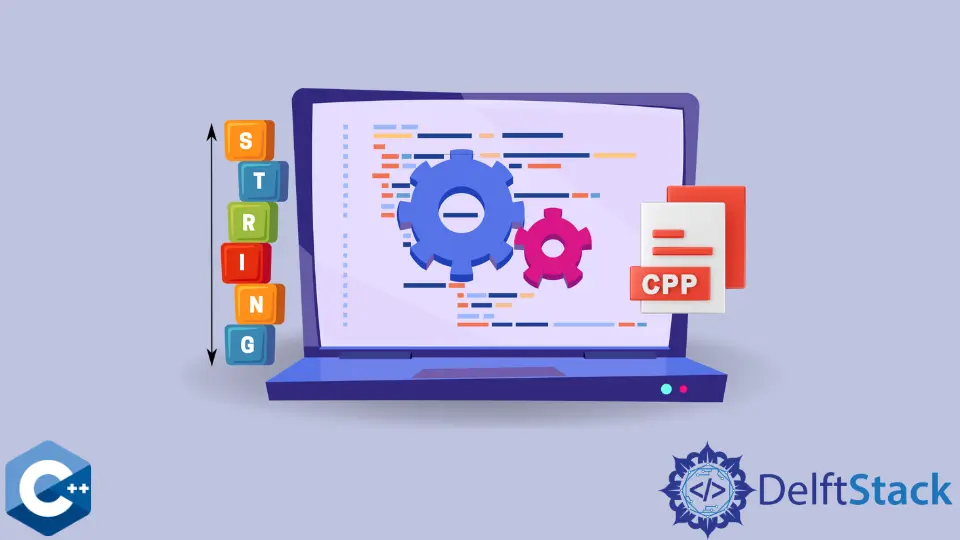
本文将介绍几种如何在 C++ 中查找字符串长度的方法。
使用 length
函数在 C++ 中查找字符串的长度
C++ 标准库提供了 std::basic_string
类,以扩展类似于 char
的序列,并实现了用于存储和处理此类数据的通用结构。虽然,大多数人对 std::string
类型更熟悉,后者本身就是 std::basic_string<char>
的类型别名。std::string
提供了 length
内置函数来检索存储的 char
序列的长度。
#include <cstring>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main(int argc, char *argv[]) {
string str1 = "this is random string oiwaoj";
cout << "string: " << str1 << endl;
cout << "length: " << str1.length() << endl;
exit(EXIT_SUCCESS);
}
输出:
string: this is random string oiwaoj
length: 28
使用 size
函数在 C++ 中查找字符串的长度
std::string
类中包含的另一个内置函数是 size
,其行为与以前的方法相似。它不带参数,并返回字符串对象中 char
元素的数量。
#include <cstring>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main(int argc, char *argv[]) {
string str1 = "this is random string oiwaoj";
cout << "string: " << str1 << endl;
cout << "length: " << str1.size() << endl;
exit(EXIT_SUCCESS);
}
输出:
string: this is random string oiwaoj
length: 28
使用 while
循环在 C++ 中查找字符串的长度
或者,可以实现自己的函数来计算字符串的长度。在这种情况下,我们利用 while
循环将字符串作为 char
序列遍历,并在每次迭代时将计数器加 1。注意,该函数将 char*
作为参数,并调用了 c_str
方法以在主函数中检索此指针。当取消引用的指针值等于 0
时,循环停止,并且以 null 终止的字符串实现保证了这一点。
#include <cstring>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
size_t lengthOfString(const char *s) {
size_t size = 0;
while (*s) {
size += 1;
s += 1;
}
return size;
}
int main(int argc, char *argv[]) {
string str1 = "this is random string oiwaoj";
cout << "string: " << str1 << endl;
cout << "length: " << lengthOfString(str1.c_str()) << endl;
exit(EXIT_SUCCESS);
}
输出:
string: this is random string oiwaoj
length: 28
使用 std::strlen
函数在 C++ 中查找字符串的长度
最后,可以使用老式的 C 字符串库函数 strlen
,该函数将单个 const char*
参数作为我们自定义的函数-lengthOfString
。当在不以空字节结尾的 char
序列上调用时,这后两种方法可能会出错,因为它可能在遍历期间访问超出范围的内存。
#include <cstring>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main(int argc, char *argv[]) {
string str1 = "this is random string oiwaoj";
cout << "string: " << str1 << endl;
cout << "length: " << std::strlen(str1.c_str()) << endl;
exit(EXIT_SUCCESS);
}
输出:
string: this is random string oiwaoj
length: 28
作者: Jinku Hu