C 语言中函数的隐式声明
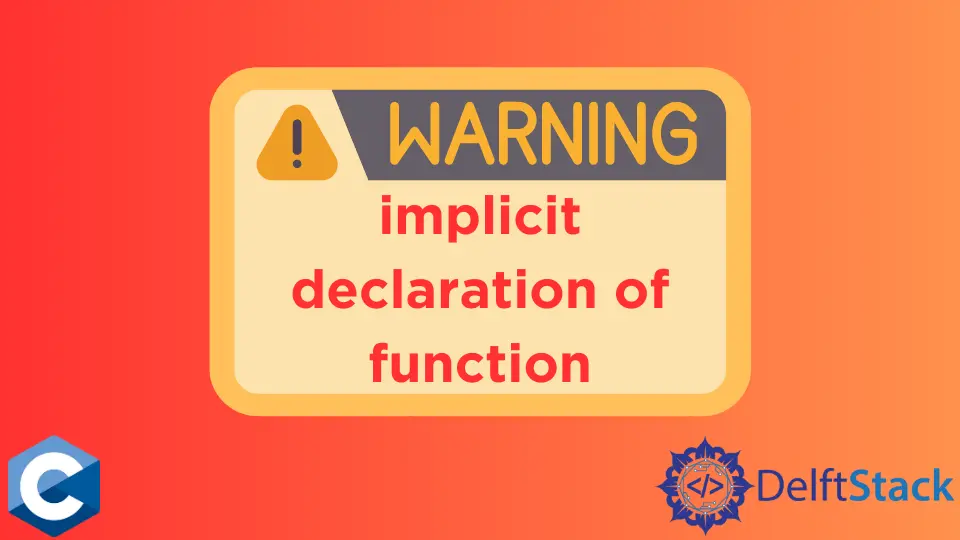
本教程讨论通过在 C 语言中声明 main 函数上方的函数来消除隐式函数声明的警告。
C 语言中函数的隐式声明
有时,编译器会在 C 语言中显示函数隐式声明的警告,这意味着该函数没有在 main()
函数之上声明,或者它的头文件不包括在内。
例如,printf()
函数属于 stdio.h
头文件,如果我们在 C 源文件中使用它之前没有包含它,编译器将显示函数声明是隐式的警告.
在这种情况下,我们必须包含头文件,其中包含函数声明或在 main()
函数之上声明函数。例如,让我们使用 printf()
函数而不包括其头文件。
请参阅下面的代码。
int main() {
int a = 10;
printf("a = %d", a);
return 0;
}
输出:
main.c: In function 'main':
main.c:5:5: warning: implicit declaration of function 'printf' [-Wimplicit-function-declaration]
5 | printf("a = %d",a);
| ^~~~~~
main.c:5:5: warning: incompatible implicit declaration of built-in function 'printf'
main.c:1:1: note: include '<stdio.h>' or provide a declaration of 'printf'
+++ |+#include <stdio.h>
1 |
a = 10
在上面的输出中,我们可以看到编译器给出了一个警告,说 printf()
函数的声明是隐式的,我们必须包含 <stdio.h>
文件,或者我们必须提供一个声明源文件中的 printf()
函数。
我们还可以看到 a
的值被打印出来,这意味着 printf()
函数可以完美运行,即使我们没有包含它的头文件。
现在,让我们包含头文件并重复上面的示例。请参阅下面的代码。
#include <stdio.h>
int main() {
int a = 10;
printf("a = %d", a);
return 0;
}
输出:
a = 10
我们可以在上面看到这次没有显示隐式函数声明的警告,因为我们已经包含了 printf()
函数的头文件。
如果我们在源文件中创建了一个函数但没有在 main()
函数上方声明它,也会显示隐式函数声明警告。
当我们尝试调用函数时,编译器会警告函数声明是隐式的。例如,让我们创建一个函数并从 main()
函数调用它,而不在 main()
函数之上声明它。
#include <stdio.h>
int main() {
int a = fun(2, 3);
printf("a = %d", a);
return 0;
}
int fun(int x, int p) {
int a = x + p;
return a;
}
输出:
main.c: In function 'main':
main.c:4:13: warning: implicit declaration of function 'fun' [-Wimplicit-function-declaration]
4 | int a = fun(2, 3);
| ^~~
a = 5
在上面的输出中,我们可以看到我们创建的函数显示了警告。我们必须在 main()
函数之上声明函数来解决这个问题。
例如,让我们在 main()
函数上方声明该函数并重复上面的示例。请参阅下面的代码。
#include <stdio.h>
int fun(int x, int p);
int main() {
int a = fun(2, 3);
printf("a = %d", a);
return 0;
}
int fun(int x, int p) {
int a = x + p;
return a;
}
输出:
a = 5
我们可以看到警告现在消失了。我们也可以在头文件中声明函数,然后将头文件包含在源文件中,这在函数很多的情况下很有用,因为它会简化代码。