C 語言中函式的隱式宣告
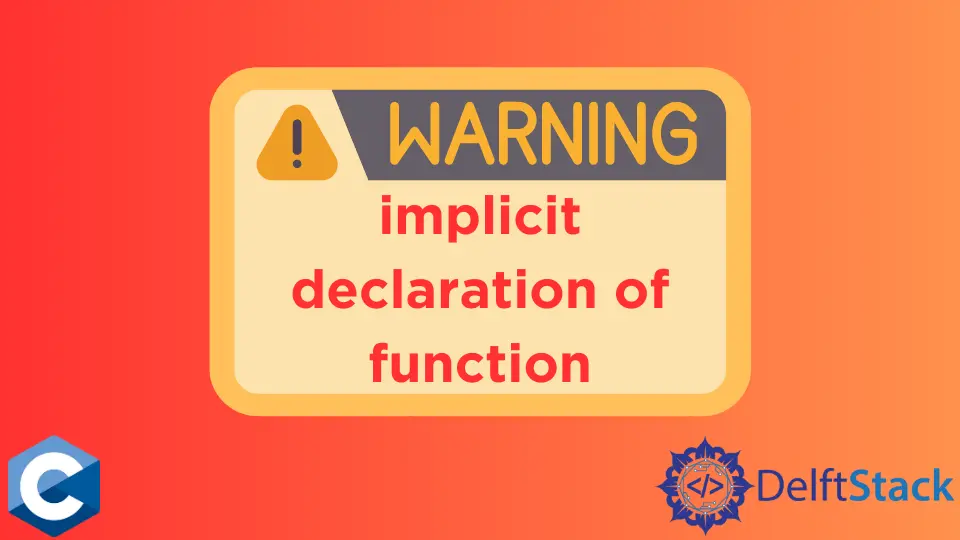
本教程討論通過在 C 語言中宣告 main 函式上方的函式來消除隱式函式宣告的警告。
C 語言中函式的隱式宣告
有時,編譯器會在 C 語言中顯示函式隱式宣告的警告,這意味著該函式沒有在 main()
函式之上宣告,或者它的標頭檔案不包括在內。
例如,printf()
函式屬於 stdio.h
標頭檔案,如果我們在 C 原始檔中使用它之前沒有包含它,編譯器將顯示函式宣告是隱式的警告.
在這種情況下,我們必須包含標頭檔案,其中包含函式宣告或在 main()
函式之上宣告函式。例如,讓我們使用 printf()
函式而不包括其標頭檔案。
請參閱下面的程式碼。
int main() {
int a = 10;
printf("a = %d", a);
return 0;
}
輸出:
main.c: In function 'main':
main.c:5:5: warning: implicit declaration of function 'printf' [-Wimplicit-function-declaration]
5 | printf("a = %d",a);
| ^~~~~~
main.c:5:5: warning: incompatible implicit declaration of built-in function 'printf'
main.c:1:1: note: include '<stdio.h>' or provide a declaration of 'printf'
+++ |+#include <stdio.h>
1 |
a = 10
在上面的輸出中,我們可以看到編譯器給出了一個警告,說 printf()
函式的宣告是隱式的,我們必須包含 <stdio.h>
檔案,或者我們必須提供一個宣告原始檔中的 printf()
函式。
我們還可以看到 a
的值被列印出來,這意味著 printf()
函式可以完美執行,即使我們沒有包含它的標頭檔案。
現在,讓我們包含標頭檔案並重覆上面的示例。請參閱下面的程式碼。
#include <stdio.h>
int main() {
int a = 10;
printf("a = %d", a);
return 0;
}
輸出:
a = 10
我們可以在上面看到這次沒有顯示隱式函式宣告的警告,因為我們已經包含了 printf()
函式的標頭檔案。
如果我們在原始檔中建立了一個函式但沒有在 main()
函式上方宣告它,也會顯示隱式函式宣告警告。
當我們嘗試呼叫函式時,編譯器會警告函式宣告是隱式的。例如,讓我們建立一個函式並從 main()
函式呼叫它,而不在 main()
函式之上宣告它。
#include <stdio.h>
int main() {
int a = fun(2, 3);
printf("a = %d", a);
return 0;
}
int fun(int x, int p) {
int a = x + p;
return a;
}
輸出:
main.c: In function 'main':
main.c:4:13: warning: implicit declaration of function 'fun' [-Wimplicit-function-declaration]
4 | int a = fun(2, 3);
| ^~~
a = 5
在上面的輸出中,我們可以看到我們建立的函式顯示了警告。我們必須在 main()
函式之上宣告函式來解決這個問題。
例如,讓我們在 main()
函式上方宣告該函式並重覆上面的示例。請參閱下面的程式碼。
#include <stdio.h>
int fun(int x, int p);
int main() {
int a = fun(2, 3);
printf("a = %d", a);
return 0;
}
int fun(int x, int p) {
int a = x + p;
return a;
}
輸出:
a = 5
我們可以看到警告現在消失了。我們也可以在標頭檔案中宣告函式,然後將標頭檔案包含在原始檔中,這在函式很多的情況下很有用,因為它會簡化程式碼。