在 Angular 中上传文件
- 在 Angular 中导入库
- 在 Angular 中导出组件和声明变量
-
在 Angular 中使用
basicUpload()
上传多个文件 -
在 Angular 中使用
basicUploadSingle()
上传单个文件 -
在 Angular 中使用
uploadAndProgress()
上传多个文件 -
在 Angular 中使用
UploadAndProgressSingle()
上传单个文件 - Angular 调用上传函数
- 概括
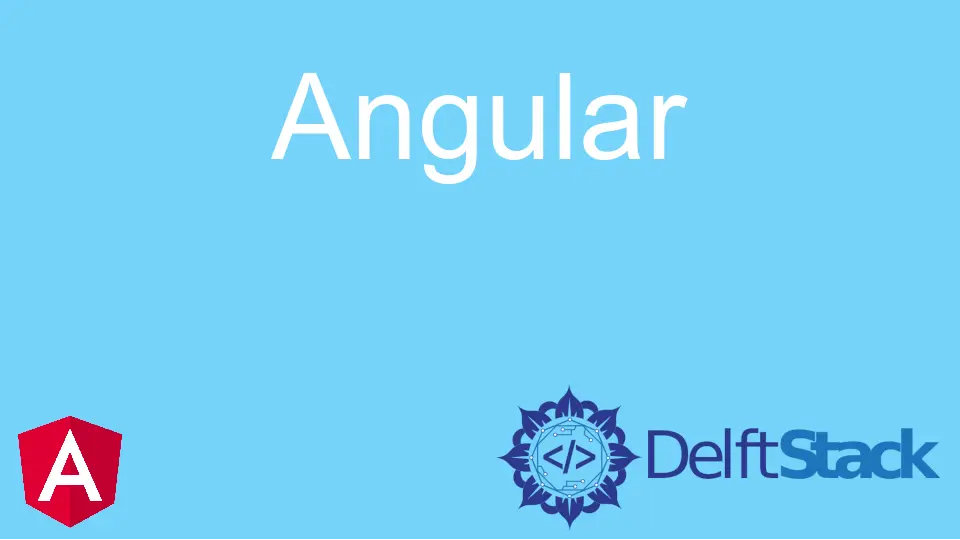
我们将介绍如何使用 Angular 上传文件的四种不同方法。
我们还将介绍如何在文件上传时显示进度条,并在上传完成时显示文件上传完成消息。
在 Angular 中导入库
首先,我们将导入库并为文件上传器设置组件。
# angular
import { Component, VERSION } from '@angular/core';
import {HttpClientModule, HttpClient, HttpRequest, HttpResponse, HttpEventType} from '@angular/common/http';
@Component({
selector: 'my-app',
template: `
Version = {{version.full}} <br/>
<input type="file" (change)="upload($event.target.files)" />
Upload Percent: {{percentDone}}% <br />
<ng-container *ngIf="uploadSuccess">
Upload Successful
</ng-container>
`,
})
在 Angular 中导出组件和声明变量
现在,我们将导出我们的 AppComponent
并将变量 percentDone
声明为 number
并将 UploadSuccess
声明为布尔值。我们还将声明一个调用 HttpClient
的构造函数。
# angular
export class AppComponent {
percentDone: number;
uploadSuccess: boolean;
constructor(
private http: HttpClient,
) { }
version = VERSION
在 Angular 中使用 basicUpload()
上传多个文件
Angular 有四种不同风格的文件上传,多个文件的 basicUpload()
就是其中之一。可以以这种方式一次上传多个文件,而无需显示任何文件进度条。
basicUpload(files: File[]){
var formData = new FormData();
Array.from(files).forEach(f => formData.append('file', f))
this.http.post('https://file.io', formData)
.subscribe(event => {
console.log('done')
})
}
我们正在向 https://file.io
发送上传请求,可以使用 后端服务器 URL
进行更改。后端服务器将接收文件的上传数据,它将向上传的文件发送一个 URL。
在 Angular 中使用 basicUploadSingle()
上传单个文件
在 Angular 中上传文件的第二种样式是单个文件的 basicUploadSingle()
。在这种风格中,用户一次只能上传一个文件。此样式也不会在文件上传时显示进度。
basicUploadSingle(file: File){
this.http.post('https://file.io', file)
.subscribe(event => {
console.log('done')
})
}
在 Angular 中使用 uploadAndProgress()
上传多个文件
在 Angular 中上传文件的第三种样式是用于多个文件的 uploadAndProgress()
。用户可以以这种方式同时上传多个文件,进度条会显示上传文件的百分比。
uploadAndProgress(files: File[]){
console.log(files)
var formData = new FormData();
Array.from(files).forEach(f => formData.append('file',f))
this.http.post('https://file.io', formData, {reportProgress: true, observe: 'events'})
.subscribe(event => {
if (event.type === HttpEventType.UploadProgress) {
this.percentDone = Math.round(100 * event.loaded / event.total);
} else if (event instanceof HttpResponse) {
this.uploadSuccess = true;
}
});
}
在 Angular 中使用 UploadAndProgressSingle()
上传单个文件
在 Angular 中上传文件的第四种风格是单个文件的 uploadAndProgressSingle()
。在这种风格中,用户可以同时上传单个文件,进度条显示上传文件的百分比。
uploadAndProgressSingle(file: File){
this.http.post('https://file.io', file, {reportProgress: true, observe: 'events'})
.subscribe(event => {
if (event.type === HttpEventType.UploadProgress) {
this.percentDone = Math.round(100 * event.loaded / event.total);
} else if (event instanceof HttpResponse) {
this.uploadSuccess = true;
}
});
}
Angular 调用上传函数
现在我们已经在 angular 中添加了四种样式的上传函数,我们可以使用所需的样式调用我们的上传函数。
upload(files: File[]){
//pick from one of the 4 styles of file uploads below
this.uploadAndProgress(files);
}
输出:
概括
我们讨论了四种不同风格的文件上传方式,以及如何在我们的 upload
函数中调用它们。完整代码如下。
import { Component, VERSION } from '@angular/core';
import {HttpClientModule, HttpClient, HttpRequest, HttpResponse, HttpEventType} from '@angular/common/http';
@Component({
selector: 'my-app',
template: `
Version = {{version.full}} <br/>
<input type="file" (change)="upload($event.target.files)" />
Upload Percent: {{percentDone}}% <br />
<ng-container *ngIf="uploadSuccess">
Upload Successful
</ng-container>
`,
})
export class AppComponent {
percentDone: number;
uploadSuccess: boolean;
constructor(
private http: HttpClient,
) { }
version = VERSION
upload(files: File[]){
this.uploadAndProgress(files);
}
basicUpload(files: File[]){
var formData = new FormData();
Array.from(files).forEach(f => formData.append('file', f))
this.http.post('https://file.io', formData)
.subscribe(event => {
console.log('done')
})
}
basicUploadSingle(file: File){
this.http.post('https://file.io', file)
.subscribe(event => {
console.log('done')
})
}
uploadAndProgress(files: File[]){
console.log(files)
var formData = new FormData();
Array.from(files).forEach(f => formData.append('file',f))
this.http.post('https://file.io', formData, {reportProgress: true, observe: 'events'})
.subscribe(event => {
if (event.type === HttpEventType.UploadProgress) {
this.percentDone = Math.round(100 * event.loaded / event.total);
} else if (event instanceof HttpResponse) {
this.uploadSuccess = true;
}
});
}
uploadAndProgressSingle(file: File){
this.http.post('https://file.io', file, {reportProgress: true, observe: 'events'})
.subscribe(event => {
if (event.type === HttpEventType.UploadProgress) {
this.percentDone = Math.round(100 * event.loaded / event.total);
} else if (event instanceof HttpResponse) {
this.uploadSuccess = true;
}
});
}
}
输出:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn