Angular로 파일 업로드
- Angular에서 라이브러리 가져오기
- Angular에서 구성 요소 내보내기 및 변수 선언
-
basicUpload()
를 사용하여 Angular로 여러 파일 업로드 -
basicUploadSingle()
를 사용하여 Angular로 단일 파일 업로드 -
uploadAndProgress()
를 사용하여 Angular로 여러 파일 업로드 -
UploadAndProgressSingle()
를 사용하여 Angular에서 단일 파일 업로드 - Angular에서 업로드 함수 호출하기
- 요약
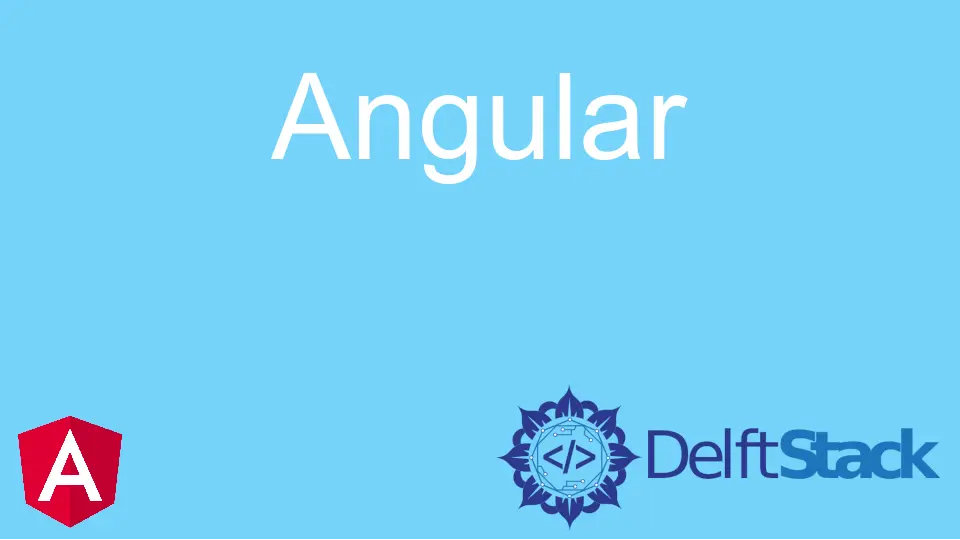
Angular를 사용하여 4가지 스타일로 파일을 업로드하는 방법을 소개합니다.
또한 파일 업로드 시 진행률 표시줄을 표시하는 방법과 업로드가 완료되면 파일 업로드 완료 메시지를 표시하는 방법도 소개합니다.
Angular에서 라이브러리 가져오기
먼저 라이브러리를 가져오고 파일 업로더에 대한 구성 요소를 설정합니다.
# angular
import { Component, VERSION } from '@angular/core';
import {HttpClientModule, HttpClient, HttpRequest, HttpResponse, HttpEventType} from '@angular/common/http';
@Component({
selector: 'my-app',
template: `
Version = {{version.full}} <br/>
<input type="file" (change)="upload($event.target.files)" />
Upload Percent: {{percentDone}}% <br />
<ng-container *ngIf="uploadSuccess">
Upload Successful
</ng-container>
`,
})
Angular에서 구성 요소 내보내기 및 변수 선언
이제 AppComponent
를 내보내고 percentDone
변수를 number
로 선언하고 UploadSuccess
를 부울로 선언합니다. 또한 HttpClient
를 호출하는 생성자를 선언합니다.
# angular
export class AppComponent {
percentDone: number;
uploadSuccess: boolean;
constructor(
private http: HttpClient,
) { }
version = VERSION
basicUpload()
를 사용하여 Angular로 여러 파일 업로드
Angular에는 4가지 다른 스타일의 파일 업로드가 있으며 여러 파일에 대한 basicUpload()
가 그 중 하나입니다. 파일의 진행률 표시줄을 표시하지 않고 이 스타일로 여러 파일을 한 번에 업로드할 수 있습니다.
basicUpload(files: File[]){
var formData = new FormData();
Array.from(files).forEach(f => formData.append('file', f))
this.http.post('https://file.io', formData)
.subscribe(event => {
console.log('done')
})
}
백엔드 서버 URL
로 변경할 수 있는 https://file.io
로 업로드 요청을 보내고 있습니다. 백엔드 서버는 업로드된 파일에 대한 URL을 보낼 파일의 업로드 데이터를 수신합니다.
basicUploadSingle()
를 사용하여 Angular로 단일 파일 업로드
Angular에서 파일을 업로드하는 두 번째 스타일은 단일 파일에 대한 basicUploadSingle()
입니다. 이 스타일에서 사용자는 한 번에 하나의 파일만 업로드할 수 있습니다. 이 스타일은 또한 파일이 업로드되는 동안 진행 상황을 표시하지 않습니다.
basicUploadSingle(file: File){
this.http.post('https://file.io', file)
.subscribe(event => {
console.log('done')
})
}
uploadAndProgress()
를 사용하여 Angular로 여러 파일 업로드
Angular에서 파일을 업로드하는 세 번째 스타일은 여러 파일에 대한 uploadAndProgress()
입니다. 사용자는 업로드된 파일의 백분율을 표시하는 진행률 표시줄과 함께 이 스타일로 여러 파일을 동시에 업로드할 수 있습니다.
uploadAndProgress(files: File[]){
console.log(files)
var formData = new FormData();
Array.from(files).forEach(f => formData.append('file',f))
this.http.post('https://file.io', formData, {reportProgress: true, observe: 'events'})
.subscribe(event => {
if (event.type === HttpEventType.UploadProgress) {
this.percentDone = Math.round(100 * event.loaded / event.total);
} else if (event instanceof HttpResponse) {
this.uploadSuccess = true;
}
});
}
UploadAndProgressSingle()
를 사용하여 Angular에서 단일 파일 업로드
Angular에서 파일을 업로드하는 네 번째 스타일은 단일 파일에 대한 uploadAndProgressSingle()
입니다. 이 스타일에서 사용자는 업로드된 파일의 백분율을 표시하는 진행률 표시줄과 함께 단일 파일을 동시에 업로드할 수 있습니다.
uploadAndProgressSingle(file: File){
this.http.post('https://file.io', file, {reportProgress: true, observe: 'events'})
.subscribe(event => {
if (event.type === HttpEventType.UploadProgress) {
this.percentDone = Math.round(100 * event.loaded / event.total);
} else if (event instanceof HttpResponse) {
this.uploadSuccess = true;
}
});
}
Angular에서 업로드 함수 호출하기
Angular에 네 가지 스타일의 업로드 기능을 추가했으므로 원하는 스타일로 업로드 기능을 호출할 수 있습니다.
upload(files: File[]){
//pick from one of the 4 styles of file uploads below
this.uploadAndProgress(files);
}
출력:
요약
Angular에서 4가지 다른 스타일의 파일 업로드와 upload
기능에서 이를 호출하는 방법에 대해 논의했습니다. 전체 코드는 아래에 나와 있습니다.
import { Component, VERSION } from '@angular/core';
import {HttpClientModule, HttpClient, HttpRequest, HttpResponse, HttpEventType} from '@angular/common/http';
@Component({
selector: 'my-app',
template: `
Version = {{version.full}} <br/>
<input type="file" (change)="upload($event.target.files)" />
Upload Percent: {{percentDone}}% <br />
<ng-container *ngIf="uploadSuccess">
Upload Successful
</ng-container>
`,
})
export class AppComponent {
percentDone: number;
uploadSuccess: boolean;
constructor(
private http: HttpClient,
) { }
version = VERSION
upload(files: File[]){
this.uploadAndProgress(files);
}
basicUpload(files: File[]){
var formData = new FormData();
Array.from(files).forEach(f => formData.append('file', f))
this.http.post('https://file.io', formData)
.subscribe(event => {
console.log('done')
})
}
basicUploadSingle(file: File){
this.http.post('https://file.io', file)
.subscribe(event => {
console.log('done')
})
}
uploadAndProgress(files: File[]){
console.log(files)
var formData = new FormData();
Array.from(files).forEach(f => formData.append('file',f))
this.http.post('https://file.io', formData, {reportProgress: true, observe: 'events'})
.subscribe(event => {
if (event.type === HttpEventType.UploadProgress) {
this.percentDone = Math.round(100 * event.loaded / event.total);
} else if (event instanceof HttpResponse) {
this.uploadSuccess = true;
}
});
}
uploadAndProgressSingle(file: File){
this.http.post('https://file.io', file, {reportProgress: true, observe: 'events'})
.subscribe(event => {
if (event.type === HttpEventType.UploadProgress) {
this.percentDone = Math.round(100 * event.loaded / event.total);
} else if (event instanceof HttpResponse) {
this.uploadSuccess = true;
}
});
}
}
출력:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn