使用 Excel VBA 將資料寫入文字檔案
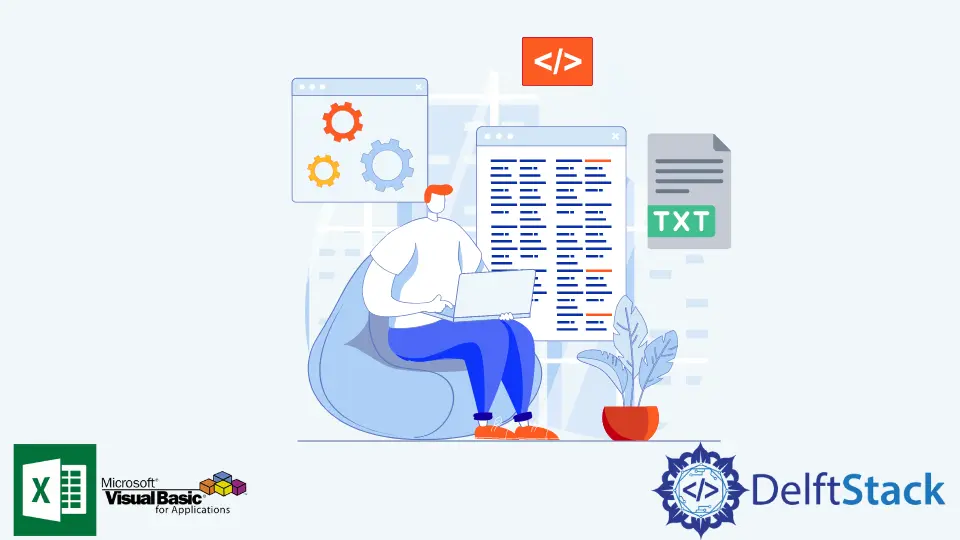
Excel VBA 在處理資料處理任務和清理時是一種流行的解決方案。此外,它可以自動執行需要花費大量時間才能完成的重複性任務。
VBA 的關鍵功能之一是它能夠生成和編輯文字檔案,而無需手動開啟、命名和儲存更改。
本文將演示如何在 VBA 的幫助下生成文字 (.txt)
檔案。
在本文中,我們嘗試使用 Excel VBA 生成文字檔案。在深入研究程式碼之前,我們需要啟用 Excel VBA 與處理檔案和資料夾的 FileSystemObject
進行互動。
啟用 FileSystemObject
的步驟:
-
開啟 Excel 檔案。
-
從
開發人員
選項卡,開啟Visual Basic
編輯器。 -
從
工具
工具欄中,單擊參考
。 -
勾選
Microsoft Scripting Runtime
核取方塊。
現在一切就緒。
下面的程式碼塊將演示使用 VBA 建立文字檔案。
SaveTextToFile
子程式需要兩個引數,fileName
和 fileContent
。fileName
變數將是要生成的文字檔案的所需檔名。fileContent
變數將是文字檔案的實際內容。應該編輯 filePath
,使其適合輸出檔案的正確位置。
建議將 FileSystemObject
宣告為 fso
以啟用 Intellisense
的自動完成功能,這可以避免印刷錯誤,還有助於發現 FileSystemObject
庫中的其他方法。
在程式碼執行結束之前包含 fso = Nothing
和 Set fileStream = Nothing
很重要,因為如果這兩個未關閉,執行時將發生錯誤。
Option Explicit
Public Sub SaveTextToFile(fileName As String, fileContent As String)
Dim filePath, fileAttributes As String
filePath = "C:\Users\temp\Desktop\Destination"
fileAttributes = filePath & "\" & fileName & ".txt"
Dim fso As FileSystemObject
Set fso = New FileSystemObject
Dim fileStream As TextStream
Set fileStream = fso.CreateTextFile(fileAttributes)
fileStream.WriteLine fileContent
fileStream.Close
If fso.FileExists(fileAttributes) Then
Debug.Print "File Created Successfully."
End If
Set fileStream = Nothing
Set fso = Nothing
End Sub
Sub testSub()
Call SaveTextToFile("sample1", "Here is the content.")
End Sub
上面的程式碼塊實際上並不與 Excel 互動,只生成一個檔案。
下面的程式碼塊將演示根據 Excel 檔案中的資料建立文字檔案。有關示例值,請參閱 Sheet1
。
工作表 1
:
| A | B | C |
1| File Name | Content | GenerateTextFile? |
2| sample1 | This is the content of sample1| Yes |
2| sample2 | This is the content of sample2| No |
2| sample3 | This is the content of sample3| Yes |
2| sample4 | This is the content of sample4| No |
2| sample5 | This is the content of sample5| Yes |
2| sample6 | This is the content of sample6| No |
在下面的示例中,我們將重用 SaveTextToFile
子例程來建立文字檔案。testSub
子程式將根據 Sheet1
的資料迴圈呼叫 SaveTextToFile
。
Option Explicit
Public Sub SaveTextToFile(fileName As String, fileContent As String)
Dim filePath, fileAttributes As String
filePath = "C:\Users\temp\Desktop\Destination"
fileAttributes = filePath & "\" & fileName & ".txt"
Dim fso As FileSystemObject
Set fso = New FileSystemObject
Dim fileStream As TextStream
Set fileStream = fso.CreateTextFile(fileAttributes)
fileStream.WriteLine fileContent
fileStream.Close
If fso.FileExists(fileAttributes) Then
Debug.Print fileName & " Created Successfully."
End If
Set fileStream = Nothing
Set fso = Nothing
End Sub
Sub testSub()
Dim wb As Workbook
Dim s1 As Worksheet
Set wb = ThisWorkbook
Set s1 = wb.Sheets("Sheet1")
Dim i As Integer
i = 2
Do Until s1.Cells(i, 1) = ""
If s1.Cells(i, 3) = "Yes" Then
Call SaveTextToFile(s1.Cells(i, 1), s1.Cells(i, 2))
End If
i = i + 1
Loop
End Sub
testSub
輸出:
sample1 Created Successfully.
sample3 Created Successfully.
sample5 Created Successfully.
Sample1
、Sample3
和 Sample5
是建立的文字檔案,因為它們在 GenerateTextFile
列中有 Yes
。Sample2
和 Sample4
未建立,因為它們在 GenerateTextFile
列中為 No
。