Escribir datos en un archivo de texto usando Excel VBA
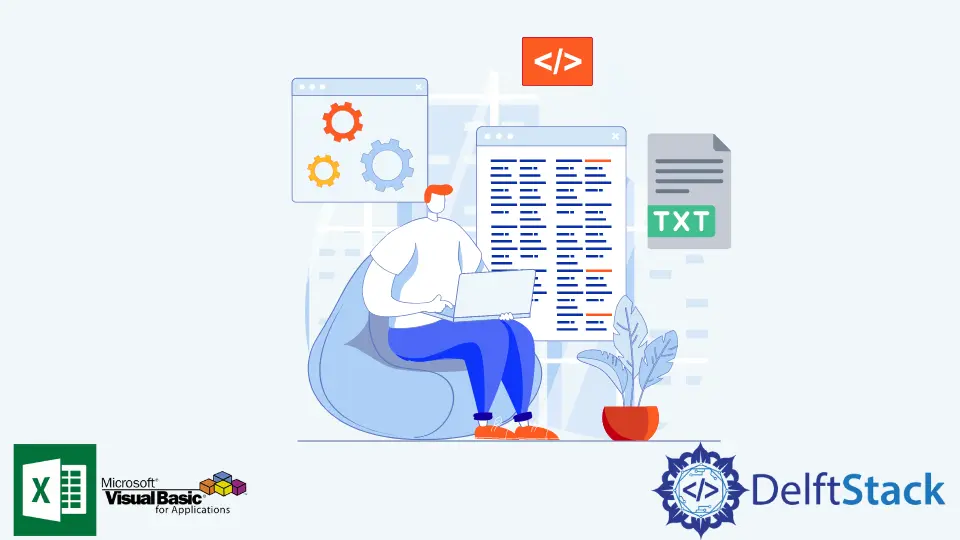
Excel VBA es una solución popular cuando se trata de tareas de procesamiento de datos y limpieza. Además, puede automatizar tareas repetitivas que llevarían mucho tiempo.
Una de las capacidades clave de VBA es su capacidad para generar y editar archivos de texto sin necesidad de abrir, nombrar y guardar cambios manualmente.
Este artículo demostrará cómo generar archivos de texto (.txt)
con la ayuda de VBA.
En este artículo, intentamos generar archivos de texto usando Excel VBA. Antes de profundizar en el código, debemos permitir que Excel VBA interactúe con FileSystemObject
, que maneja archivos y carpetas.
Pasos para habilitar FileSystemObject
:
-
Abrir archivo de Excel.
-
Desde la pestaña
Desarrollador
, abre el EditorVisual Basic
. -
Desde la barra de herramientas
Tools
, haga clic enReferences
. -
Marque la casilla de verificación
Microsoft Scripting Runtime
.
Ya está todo listo.
El bloque de código a continuación demostrará la creación de un archivo de texto usando VBA.
La subrutina SaveTextToFile
requiere dos parámetros, el fileName
y el fileContent
. La variable fileName
será el nombre de archivo deseado del archivo de texto a generar. La variable fileContent
será el contenido real del archivo de texto. El filePath
debe editarse para que encaje con la ubicación correcta del archivo de salida.
Se recomienda declarar el FileSystemObject
como fso
para permitir que funcione el autocompletado de Intellisense
, lo que podría evitar errores tipográficos y también ayudaría a descubrir otro método dentro de la biblioteca FileSystemObject
.
Es importante incluir fso = Nothing
y Set fileStream = Nothing
antes de que finalice la ejecución del código, ya que se producirán errores durante el tiempo de ejecución si estos dos no están cerrados.
Option Explicit
Public Sub SaveTextToFile(fileName As String, fileContent As String)
Dim filePath, fileAttributes As String
filePath = "C:\Users\temp\Desktop\Destination"
fileAttributes = filePath & "\" & fileName & ".txt"
Dim fso As FileSystemObject
Set fso = New FileSystemObject
Dim fileStream As TextStream
Set fileStream = fso.CreateTextFile(fileAttributes)
fileStream.WriteLine fileContent
fileStream.Close
If fso.FileExists(fileAttributes) Then
Debug.Print "File Created Successfully."
End If
Set fileStream = Nothing
Set fso = Nothing
End Sub
Sub testSub()
Call SaveTextToFile("sample1", "Here is the content.")
End Sub
El bloque de código anterior en realidad no interactúa con Excel y genera un solo archivo.
El bloque de código a continuación demostrará la creación de archivos de texto según los datos del archivo de Excel. Consulte la Sheet1
para ver los valores de muestra.
Sheet1
:
| A | B | C |
1| File Name | Content | GenerateTextFile? |
2| sample1 | This is the content of sample1| Yes |
2| sample2 | This is the content of sample2| No |
2| sample3 | This is the content of sample3| Yes |
2| sample4 | This is the content of sample4| No |
2| sample5 | This is the content of sample5| Yes |
2| sample6 | This is the content of sample6| No |
En el ejemplo siguiente, reutilizaremos la subrutina SaveTextToFile
para la creación del archivo de texto. La subrutina testSub
llamará a SaveTextToFile
en un bucle dependiendo de los datos de Sheet1
.
Option Explicit
Public Sub SaveTextToFile(fileName As String, fileContent As String)
Dim filePath, fileAttributes As String
filePath = "C:\Users\temp\Desktop\Destination"
fileAttributes = filePath & "\" & fileName & ".txt"
Dim fso As FileSystemObject
Set fso = New FileSystemObject
Dim fileStream As TextStream
Set fileStream = fso.CreateTextFile(fileAttributes)
fileStream.WriteLine fileContent
fileStream.Close
If fso.FileExists(fileAttributes) Then
Debug.Print fileName & " Created Successfully."
End If
Set fileStream = Nothing
Set fso = Nothing
End Sub
Sub testSub()
Dim wb As Workbook
Dim s1 As Worksheet
Set wb = ThisWorkbook
Set s1 = wb.Sheets("Sheet1")
Dim i As Integer
i = 2
Do Until s1.Cells(i, 1) = ""
If s1.Cells(i, 3) = "Yes" Then
Call SaveTextToFile(s1.Cells(i, 1), s1.Cells(i, 2))
End If
i = i + 1
Loop
End Sub
Salida testSub
:
sample1 Created Successfully.
sample3 Created Successfully.
sample5 Created Successfully.
Sample1
, Sample3
y Sample5
son los archivos de texto creados porque tienen Sí
en la columna GenerateTextFile
. Sample2
y Sample4
no se crean porque son No
en la columna GenerateTextFile
.