在 VBA 中檢查一個字串是否包含一個子字串
Glen Alfaro
2023年1月30日
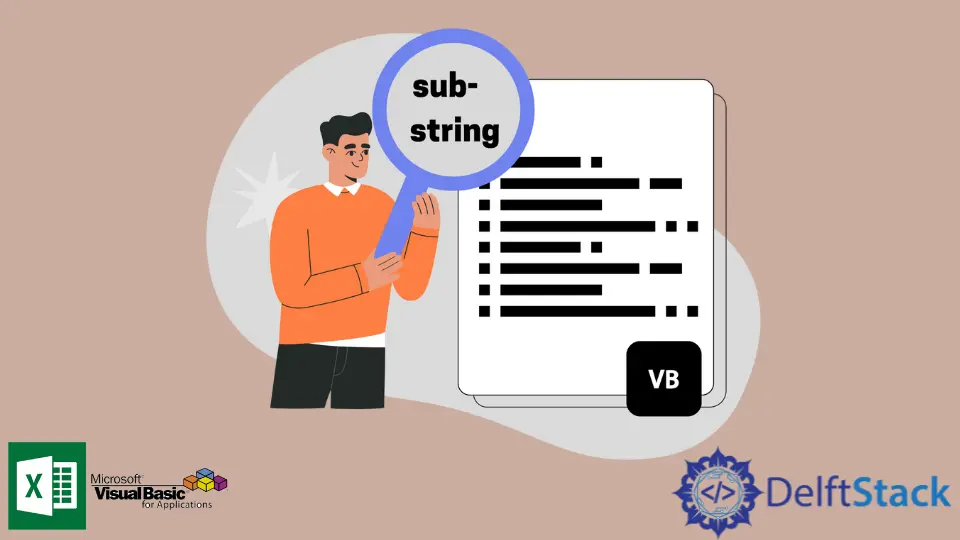
本文將演示使用 Instr()
函式、InstrRev()
函式和 Like
函式來檢查主字串是否包含子字串。
使用 Instr()
函式檢查主字串是否包含子字串
Instr()
函式語法:
InStr([ start ], string1, string2, [ compare ])
返回型別:整數
引數:
[ start ] |
可選的。搜尋將開始的數值。 對於 [ start ] 引數,以下是相應的值:1 - [預設] 搜尋將從主字串的開頭開始< br/> n - 搜尋將從 n 位置開始。 |
string1 |
強制的。要搜尋的字串(主字串) |
string2 |
強制的。要查詢的字串。 |
[ compare ] |
可選的。指示將使用哪種字串比較方法。 對於 [ compare ] 引數,以下是相應的值:0 - [預設] 二進位制比較方法(區分大小寫)1 - 文字比較方法(不區分大小寫) |
下面的程式碼塊將使用 Instr()
函式檢查子字串是否在 VBA 的主字串中。
Function IsSubstring(pos as Integer, mainStr as String, subStr as String,compTyp as Integer) as boolean
'if `Instr()` function returned 0 then the substring is not present in the main string.
'If `Instr()` function returned a value greater than `0`, would mean that the substring is in the main string.
If Instr(pos,mainStr,subStr,compTyp) >0 Then
IsSubstring = true
Else: IsSubstring = false
End if
End Function
Sub test1()
Debug.print IsSubstring(1,"ABCDE","C",1)
End Sub
Sub test2()
Debug.print IsSubstring(1,"ABCDE","F",1)
End Sub
Sub test3()
Debug.print IsSubstring(1,"ABCDE","c",0)
End Sub
輸出 test1
:
True
輸出 test2
:
False
輸出 test3
:
False
下面的程式碼塊將使用 Instr()
函式從主字串返回子字串的位置。
Function GetPosition(pos as Integer, mainStr as String, subStr as String,compTyp as Integer)
'Check first if the substring is in the main string.
If InStr(pos, mainStr, subStr, compTyp) > 0 Then
'if substring is in the main string then get the position of the substring in the main string.
GetPosition = InStr(1, mainStr, subStr, 1)
Else: GetPosition = ("Subtring is not in the main string.")
End If
End Function
Sub test1()
'Check if `C` is in `ABCDE` starting at the first letter (A), case insensitive.
Debug.Print GetPosition(1,"ABCDE", "C",1)
End Sub
Sub test2()
'Check if `c` is in `ABCDE` starting at the first letter of the main string, case sensitive.
Debug.Print GetPosition(1,"ABCDE", "c",0)
End Sub
Sub test3()
'Check if `c` is in `ABCDE` starting at the fourth letter of the main string, case sensitive.
Debug.Print GetPosition(4,"ABCDE", "c",0)
End Sub
輸出 test1
:
3
輸出 test2
:
Subtring is not in the main string.
輸出 test3
:
Subtring is not in the main string.
使用 InstrRev()
函式檢查主字串是否包含子字串
InstrRev()
函式語法:
InStrRev(string1, string2,[ start ], [ compare ])
返回型別:整數
引數:
string1 |
強制的。要搜尋的字串(主字串) |
string2 |
強制的。要查詢的字串。 |
下面的程式碼塊將使用 InstrRev()
函式檢查子字串是否在 VBA 的主字串中。
Function IsSubstring(mainStr As String, subStr As String) As Boolean
'if `InstrRev()` function returned 0 then the substring is not present in the main string.
'If `InstrRev()` function returned a value greater than `0`, would mean that the substring is in the main string.
If InStrRev(mainStr, subStr) > 0 Then
IsSubstring = True
Else: IsSubstring = False
End If
End Function
Sub test1()
Debug.Print IsSubstring("ABCDE", "C")
End Sub
Sub test2()
Debug.Print IsSubstring("ABCDE", "F")
End Sub
輸出 test1
:
True
輸出 test2
:
False
使用 Like
運算子檢查主字串是否包含子字串
Like
運算子語法:
res = string Like pattern
返回型別:布林值
引數:
res |
強制的。布林返回值 |
string |
強制的。要檢視的字串 |
pattern |
強制的。要查詢的字串。更多詳情見備註 |
備註:
? |
任何單個字元 |
* |
任意 0 到多個字元 |
# |
任何單個數字(0 到 9) |
下面的程式碼塊將使用 Like
運算子檢查子字串是否在 VBA 的主字串中
Function IsSubString(mainStr as String,subStr as String) as Boolean
'Check if subStr is in the main string by using *
If mainStr Like "*" & subStr & "*" Then
IsSubString = True
Else: IsSubstring= False
End If
End Function
Sub test1()
Debug.print (IsSubString("ABCDE","C"))
End Sub
Sub test2()
Debug.print (IsSubString("ABCDE","c"))
End Sub
Sub test3()
Debug.print (IsSubString("ABCDE","F"))
End Sub
輸出 test1
:
True
輸出 test2
:
False
輸出 test3
:
False