從 Python 列表中刪除標點符號
-
Python 中的
string.punctuation
常量 -
在 Python 中使用
for
迴圈從列表中刪除標點符號 - 使用 Python 中的列表推導從列表中刪除標點符號
-
使用 Python 中的
str.translate()
函式從列表中刪除標點符號
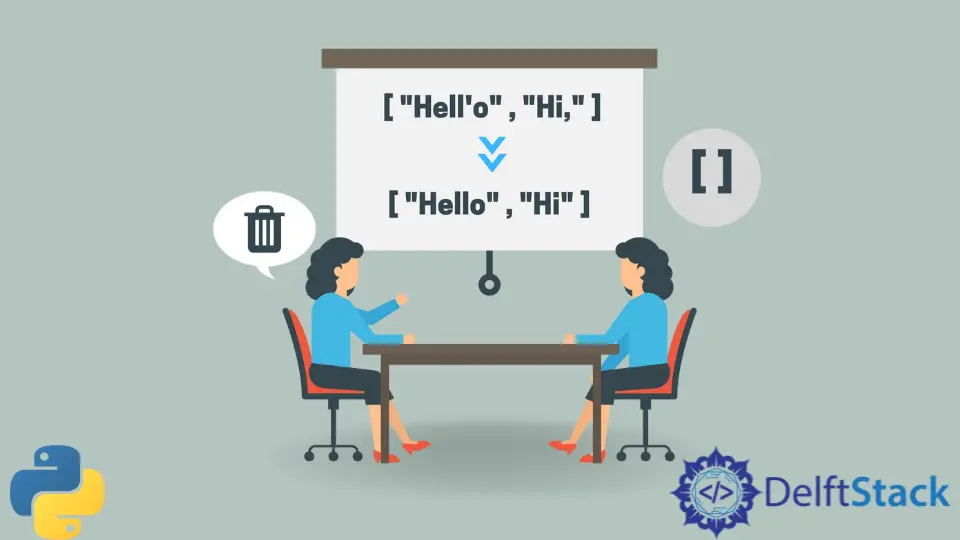
本教程將介紹字串常量 string.punctuation
,並討論在 Python 中從字串列表中刪除標點符號的一些方法。
Python 中的 string.punctuation
常量
string.punctuation
是 Python 中包含所有標點符號的預初始化字串。要使用這個字串,我們必須匯入 string
模組。string.punctuation
常量顯示在以下編碼示例中。
import string
print(string.punctuation)
輸出:
!"#$%&'()*+,-./:;<=>?@[\]^_`{|}~
我們匯入了 string
模組並顯示了 string.punctuation
常量的值。輸出顯示了所有可能的英語標點符號。
在 Python 中使用 for
迴圈從列表中刪除標點符號
我們可以通過在 Python 中使用 string.punctuation
和 for
迴圈從字串列表中刪除所有標點符號。下面的程式碼示例演示了這種現象。
import string
words = ["hell'o", "Hi,", "bye bye", "good bye", ""]
new_words = []
for word in words:
for letter in word:
if letter in string.punctuation:
word = word.replace(letter, "")
new_words.append(word)
print(new_words)
輸出:
['hello', 'Hi', 'bye bye', 'good bye', '']
我們初始化了一個包含標點符號的字串 words
列表。然後我們建立了一個巢狀迴圈,它遍歷 words
列表的每個字串中的每個字元。外層 for
迴圈遍歷列表中的每個字串,內層 for
迴圈遍歷該字串的每個 letter
。然後我們使用 if
語句檢查該 letter
是否在 string.punctuation
常量內。如果字母出現在 string.punctuation
常量中,我們通過用空字串替換它來刪除它。從字串中刪除所有標點符號後,我們將該字串附加到我們的 new_words
列表中。最後,我們列印了 new_words
列表。
此實現的唯一問題是它允許空字串保留在最終列表中。根據我們的要求,我們還可以通過在迴圈中放置額外的檢查來從原始列表中刪除空字串。以下程式碼片段也顯示瞭如何從列表中刪除空字串。
import string
words = ["hell'o", "Hi,", "bye bye", "good bye", ""]
new_words = []
for word in words:
if word == "":
words.remove(word)
else:
for letter in word:
if letter in string.punctuation:
word = word.replace(letter, "")
new_words.append(word)
print(new_words)
輸出:
['hello', 'Hi', 'bye bye', 'good bye']
這一次,我們的程式碼還從原始字串中刪除了所有空字串。
使用 Python 中的列表推導從列表中刪除標點符號
前一種方法的唯一問題是它需要太多程式碼來完成從字串列表中刪除標點符號的簡單任務。列表推導式是一種對列表元素執行不同計算操作的方法。我們可以在列表推導式中使用 for
迴圈和 if
語句。使用列表推導式的主要優點是它們需要的程式碼更少,而且通常比簡單的 for
迴圈更快。我們可以使用帶有 string.punctuation
字串常量的列表推導來從 Python 中的字串列表中刪除標點符號。下面的程式碼示例向我們展示瞭如何使用列表推導從列表中刪除標點符號。
import string
words = ["hell'o", "Hi,", "bye bye", "good bye", ""]
words = [
"".join(letter for letter in word if letter not in string.punctuation)
for word in words
]
print(words)
輸出:
['hello', 'Hi', 'bye bye', 'good bye', '']
老實說,理解上面的程式碼有點困難,但它並不複雜。它只是使用巢狀列表推導。程式碼的內部部分檢查單個單詞中的每個字母是否出現在 string.punctuation
常量中,並且只返回那些不在 string.punctuation
中的字母。包含這部分程式碼的 str.join()
函式將所有返回的字母與一個空字串連線起來,併為我們提供一個沒有任何標點符號的完整單詞。外部部分為我們的 words
列表中的每個單詞執行這個內部列表推導。我們將外部列表推導返回的單詞儲存到 words
列表中。最後,我們顯示 words
列表的所有元素。
使用列表推導式的另一個優點是我們節省了 RAM 上的空間,即在我們的程式碼中,我們更新了原始列表,而不是建立一個新列表來儲存結果。我們還可以通過在外部列表推導式中放置一個額外的 if
語句來從原始列表中刪除空字串。
import string
words = ["hell'o", "Hi,", "bye bye", "good bye", ""]
words = [
"".join(letter for letter in word if letter not in string.punctuation)
for word in words
if word
]
print(words)
輸出:
['hello', 'Hi', 'bye bye', 'good bye']
這一次,當單詞中沒有元素時,我們的外部列表推導不會執行內部列表推導。使用這種方法,我們不會在結果字串列表中得到空字串。
使用 Python 中的 str.translate()
函式從列表中刪除標點符號
我們之前的實現很好,因為它需要更少的程式碼並且比使用傳統迴圈更快,但它可以更好。雖然程式碼較少,但是程式碼有點複雜。從 Python 中的字串列表中刪除標點符號的最快和最有效的方法是 str.translate()
函式。與列表推導式相比,它需要的程式碼更少,而且速度要快得多。str.translate()
函式 根據翻譯表對映字串中的每個字元。在我們的例子中,它將把 string.punctuation
中的所有字母對映到一個空字串。下面的程式碼示例向我們展示瞭如何使用 str.translate()
函式從列表中刪除標點符號。
import string
words = ["hell'o", "Hi,", "bye bye", "good bye", ""]
words = [word.translate(string.punctuation) for word in words]
print(words)
輸出:
["hell'o", 'Hi,', 'bye bye', 'good bye', '']
我們使用帶有 string.punctuation
常量和列表推導式的 str.translate()
函式來從我們的 words
列表中刪除標點符號。word.translate(string.punctuation)
將 string.punctuation
常量中的每個字母對映到一個空字串,列表推導對 words
列表中的每個字串執行此程式碼並返回結果。我們將所有返回的字串分配給 words
列表並顯示輸出。
輸出在結果中顯示一個空字串。為了進一步刪除這個空字串,我們必須在我們的列表推導中放置一個額外的條件。
import string
words = ["hell'o", "Hi,", "bye bye", "good bye", ""]
words = [word.translate(string.punctuation) for word in words if word]
print(words)
輸出:
["hell'o", 'Hi,', 'bye bye', 'good bye']
我們從前一個結果中刪除了空字串,只增加了一個條件。
string.punctuation
是一個預定義的常量字串,包含所有可能的標點符號。多種方法使用這個字串常量從字串列表中刪除標點符號,但最容易編寫、最快和最有效的實現是使用帶有列表推導式的 str.translate()
函式。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn相關文章 - Python List
- 在 Python 中將字典轉換為列表
- 從 Python 列表中刪除某元素的所有出現
- 在 Python 中從列表中刪除重複項
- 如何在 Python 中獲取一個列表的平均值
- Python 列表方法 append 和 extend 之間有什麼區別
- 如何在 Python 中將列表轉換為字串