從 Python 中的列表中刪除列表
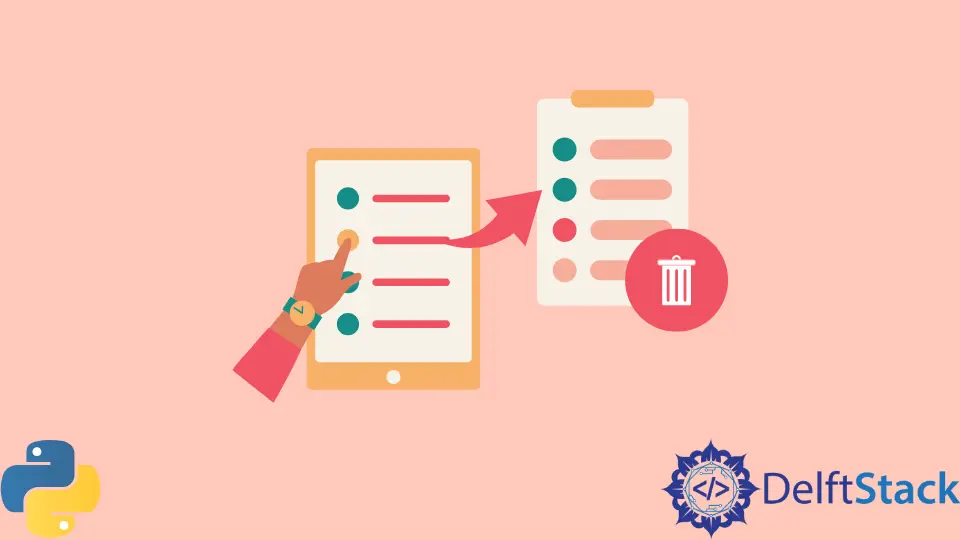
Python 中的列表是一種資料結構,其中包含專案的順序序列。我們可以對列表執行許多操作。假設我們要從列表 A 中刪除列表 B。這僅意味著我們要從列表 A 中刪除也存在於列表 B 中的專案。
例如,我們有一個列表 A,其中包含專案 ["Blue", "Pink", "Purple", "Red"]
,列表 B 包含專案 ["Silver", "Red", "Golden", "Pink"]
。現在,如果我們從列表 A 中刪除列表 B,在輸出中,我們將得到列表 A 作為 ["Blue", "Purple"]
,因為這些專案也存在於列表 B 中。我們可以通過使用來完成此任務帶有列表的 remove()
函式或使用 set
資料結構可用的 difference()
函式。
使用 Python 中的 remove()
方法從列表 a 中刪除列表 B
在這個例子中,我們將使用列表 A 上的 remove()
方法刪除列表 A 和列表 B 中相似的專案。我們使用列表 A 的 remove()
方法,以便從列表中刪除專案列表 A,但列表 B 將與以前相同。在這段程式碼中,我們遍歷列表 A 的專案並檢查該專案是否也存在於列表 B 中;該專案將從列表 A 中刪除。
示例程式碼:
# Python 3.x
list_A = ["Blue", "Pink", "Purple", "Red"]
list_B = ["Silver", "Red", "Golden", "Pink"]
print("List A before:", list_A)
print("List B before:", list_B)
for item in list_A:
if item in list_B:
list_A.remove(item)
print("List A now:", list_A)
print("List B now:", list_B)
輸出:
List A before: ['Blue', 'Pink', 'Purple', 'Red']
List B before: ['Silver', 'Red', 'Golden', 'Pink']
List A now: ['Blue', 'Purple']
List B now: ['Silver', 'Red', 'Golden', 'Pink']
使用 Python 中的 difference()
方法從列表 a 中刪除列表 B
從列表 A 中刪除相似專案的另一種方法是從列表 B 中減去它們。使用 set
資料結構,有一個方法 difference()
將返回存在於集合 A 中但不存在於集合 B 中的專案。它僅返回集合 A 的不同項,它們在兩個集合之間是唯一的。但由於此方法可用於 set
。
因此,在我們的程式碼中,我們首先將兩個列表強制轉換為 set,然後應用 set_A.difference(set_B)
函式,我們將通過將結果強制轉換為列表資料型別再次將結果儲存在 list_A 中。
示例程式碼:
# Python 3.x
list_A = ["Blue", "Pink", "Purple", "Red"]
list_B = ["Silver", "Red", "Golden", "Pink"]
print("List A before:", list_A)
print("List B before:", list_B)
setA = set(list_A)
setB = set(list_B)
list_A = list(setA.difference(list_B))
print("List A now:", list_A)
print("List B now:", list_B)
輸出:
List A before: ['Blue', 'Pink', 'Purple', 'Red']
List B before: ['Silver', 'Red', 'Golden', 'Pink']
List A now: ['Purple', 'Blue']
List B now: ['Silver', 'Red', 'Golden', 'Pink']
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn