使用 Python 查詢檔案
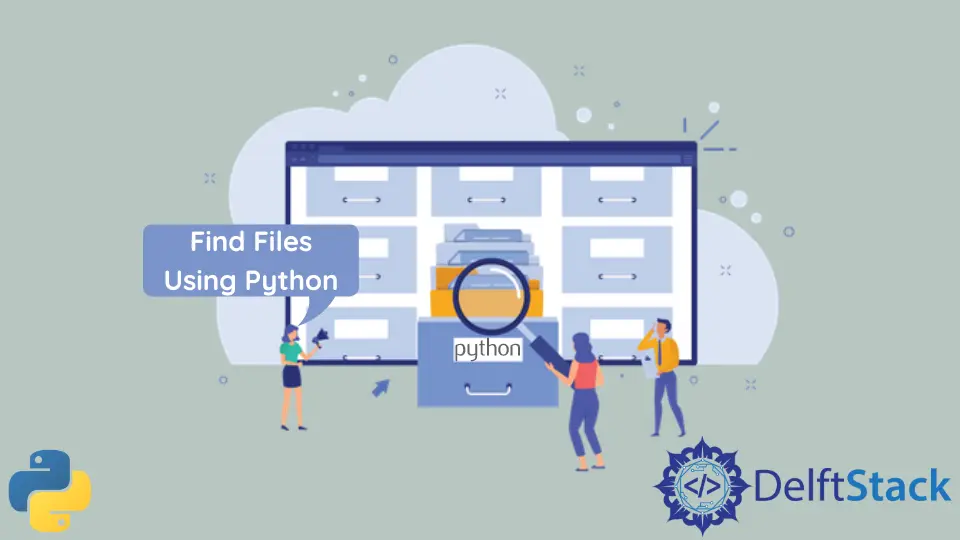
本教程將討論在 Python 中查詢檔案的方法。
在 Python 中使用 os.walk()
函式查詢檔案
如果我們想使用 python 在我們的機器上找到特定檔案的路徑,我們可以使用 os
模組。os
模組為我們的程式碼提供了許多與作業系統相關的功能。os.walk()
函式 將 path
字串作為輸入引數,併為我們提供目錄路徑、目錄名稱和 path
中每個檔案的檔名。下面的示例程式碼向我們展示瞭如何使用 os.walk()
函式在 Python 中查詢檔案。
import os
def findfile(name, path):
for dirpath, dirname, filename in os.walk(path):
if name in filename:
return os.path.join(dirpath, name)
filepath = findfile("file2.txt", "/")
print(filepath)
輸出:
/Users\maisa\Documents\PythonProjects\file2.txt
在上面的程式碼中,我們宣告瞭 findfile()
函式,該函式使用 os.walk()
函式來查詢我們的檔案。findfile()
函式將檔名和根路徑作為輸入引數,並返回我們指定檔案的路徑。這種方法為我們提供了檔案的絕對路徑。
在 Python 中使用 glob.glob()
函式查詢檔案
我們還可以使用 glob.glob()
函式 來解決我們當前的問題。glob.glob()
函式將路徑名作為輸入引數,並返回與輸入引數匹配的所有檔案路徑的列表。我們可以指定一個正規表示式作為只匹配我們檔案的輸入引數。下面的示例程式碼向我們展示瞭如何使用 glob.glob()
函式在 Python 中查詢檔案。
import glob
filepath = glob.glob("**/file.txt", recursive=True)
print(filepath)
輸出:
['Find File\\file.txt']
我們將檔名作為輸入引數傳遞給 glob.glob()
函式,它返回我們檔案的相對路徑。此方法可以為我們提供檔案的相對路徑以及絕對路徑。
在 Python 中使用 Path.glob()
函式查詢檔案
另一種方法是使用 pathlib
模組。這個 Python 模組提供了代表不同作業系統的檔案系統路徑的類。我們可以使用 pathlib
模組中的 Path.glob()
函式 來解決我們的特定問題。這個函式類似於 glob
模組中的 glob()
函式。Path.glob()
函式將模式作為輸入引數,並返回與輸入引數匹配的路徑物件列表。示例程式碼片段向我們展示瞭如何使用 pathlib
模組在 Python 中查詢檔案。
import pathlib
filepath = sorted(pathlib.Path(".").glob("**/file2.txt"))
print(filepath)
輸出:
[WindowsPath('file2.txt')]
我們將與我們的檔案匹配的模式字串傳遞給 Path.glob()
函式。Path.glob()
函式返回一個與模式匹配的 WindowsPath
物件列表。使用這種方法,我們可以獲得特定於我們作業系統的路徑物件。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn