在 Python 中遍歷目錄中的檔案
-
在 Python 中使用
os.listdir()
方法遍歷目錄中的檔案 -
在 Python 中使用
pathlib.path().glob()
方法遍歷目錄中的檔案 -
在 Python 中使用
os.walk()
方法遍歷目錄中的檔案 -
在 Python 中使用
iglob()
方法遍歷目錄中的檔案
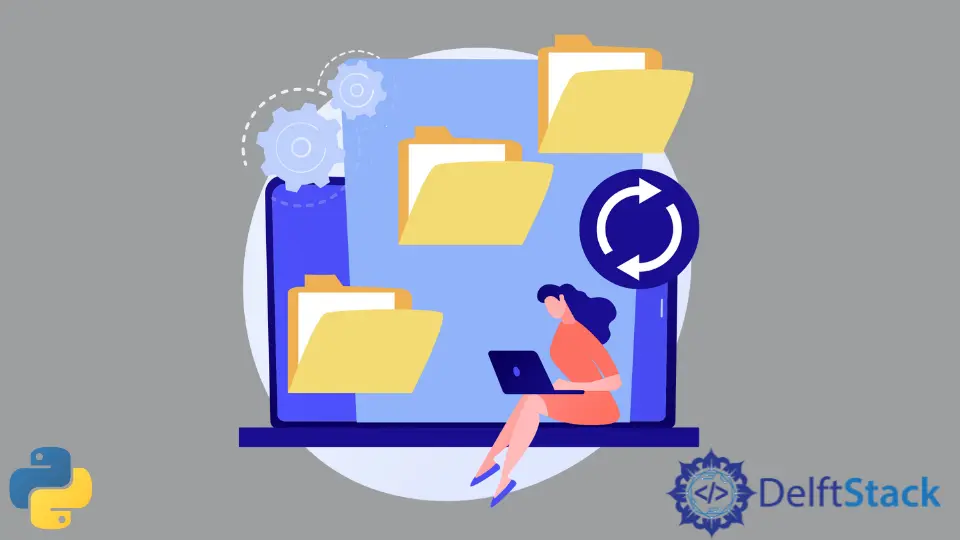
本教程將講解在 Python 中遍歷目錄中的檔案的不同方法。我們需要在目錄中尋找一個檔案來實現檔案共享、檔案檢視或檔案載入等功能,以對儲存在其中的資料執行一些操作。
假設我們有一個檔名,需要從這個檔案中讀取資料,我們需要一些方法來遍歷目錄中的檔案,以找到我們需要的檔案。下面解釋了 Python 中用於遍歷目錄中的檔案的各種方法。
在 Python 中使用 os.listdir()
方法遍歷目錄中的檔案
os
模組的 listdir()
方法將目錄路徑作為輸入,並返回該目錄中所有檔案的列表。由於我們要找到該目錄中的具體檔案,所以我們要通過檔名的迴圈來找到所需的檔案。下面的程式碼示例演示瞭如何使用 Python 中的 listdir()
方法,通過迭代找到一個特定的檔案。
import os
files = os.listdir("Desktop/myFolder")
myfile = "filename.txt"
for filename in files:
if filename == myfile:
continue
在 Python 中使用 pathlib.path().glob()
方法遍歷目錄中的檔案
pathlib
模組的 path()
方法將目錄路徑字串作為輸入,並返回該目錄和子目錄中所有檔案的路徑列表。
假設我們要查詢的檔案是 .txt
檔案,我們可以通過 path().glob()
方法得到所有 .txt
檔案的路徑。下面的程式碼示例演示瞭如何使用 Python 中的 path
方法遍歷一個目錄中所有的 .txt
檔案。
from pathlib import Path
pathlist = Path("Desktop/myFolder").glob("**/*.txt")
myfile = "filename.txt"
for path in pathlist:
if path.name == myfile:
continue
模式**/*.txt
返回當前資料夾及其子資料夾中所有副檔名為 txt
的檔案。path.name
只返回檔名而不是完整的路徑。
在 Python 中使用 os.walk()
方法遍歷目錄中的檔案
os
模組的 walk()
方法也是將目錄路徑字串作為輸入,並將根目錄的路徑作為字串返回,子目錄的列表,以及當前目錄及其子目錄中所有檔案的列表。
要找到名稱為 filename.txt
的檔案,我們可以先得到該目錄中的所有檔案,然後再通過迴圈得到所需的檔案。下面的程式碼示例演示瞭如何通過遍歷一個目錄中的檔案來查詢檔案。
import os
myfile = "filename.txt"
for root, dirs, files in os.walk("Desktop/myFolder"):
for file in files:
if file == myfile:
print(file)
在 Python 中使用 iglob()
方法遍歷目錄中的檔案
glob()
模組的 iglob()
方法將所需檔案的目錄路徑和副檔名作為輸入,並返回所有具有相同副檔名的檔案路徑。下面的程式碼示例演示瞭如何在 Python 中使用 iglob()
方法在目錄中迴圈查詢所需檔案。
import glob
for filepath in glob.iglob("drive/test/*.txt"):
if filepath.endswith("/filename.txt"):
print(filepath)