如何在 Python 中從列表中獲取唯一值
Hassan Saeed
2023年1月30日
Python
Python List
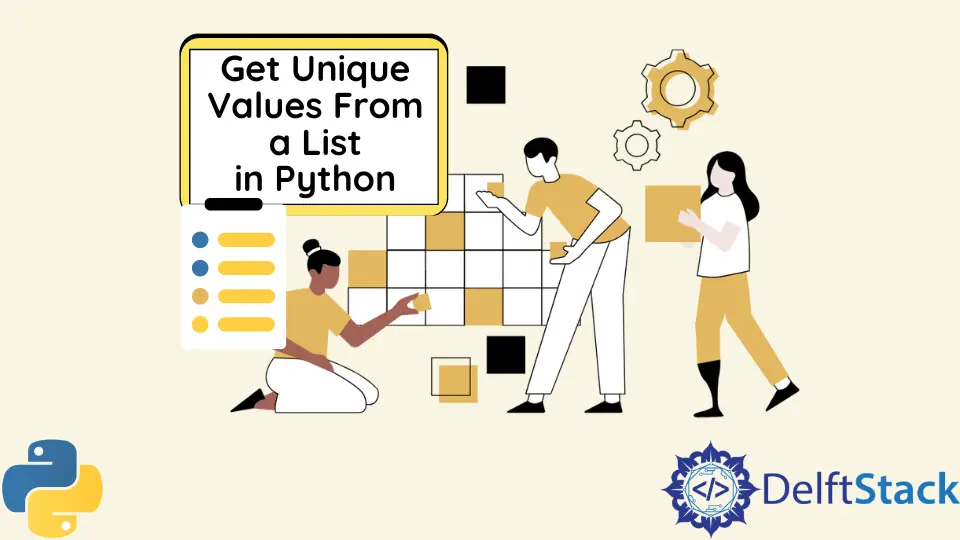
本教程討論了在 Python 中從一個列表中獲取唯一值的方法。
使用 dict.fromkeys
從 Python 中的列表中獲取唯一值
我們可以使用 dict
類的 dict.fromkeys
方法從一個 Python 列表中獲取唯一的值。這個方法保留了元素的原始順序,並且只保留重複的第一個元素。下面的例子說明了這一點。
inp_list = [2, 2, 3, 1, 4, 2, 5]
unique_list = list(dict.fromkeys(inp_list))
print(unique_list)
輸出:
[2, 3, 1, 4, 5]
在 Python 中使用一個新的列表來獲取原始列表中的唯一值
在 Python 中從一個列表中獲取唯一值的另一種方法是建立一個新的列表,並從原列表中只新增唯一的元素。這種方法保留了元素的原始順序,只保留重複的第一個元素。下面的例子說明了這一點。
inp_list = [2, 2, 3, 1, 4, 2, 5]
unique_list = []
[unique_list.append(x) for x in inp_list if x not in unique_list]
unique_list
輸出:
[2, 3, 1, 4, 5]
在 Python 中使用 set()
從列表中獲取唯一值
在 Python 中,set
只儲存唯一的值。我們可以將列表中的值插入到 set
中以獲得唯一的值。然而,這種方法並不保留元素的順序。下面的例子說明了這一點。
inp_list = [2, 2, 3, 1, 4, 2, 5]
unique_list = list(set(inp_list))
print(unique_list)
輸出:
[1, 2, 3, 4, 5]
請注意,元素的順序並沒有像在原始列表中那樣被保留下來。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe