使用 Python 實現高通濾波器
Vaibhav Vaibhav
2022年5月17日
Python
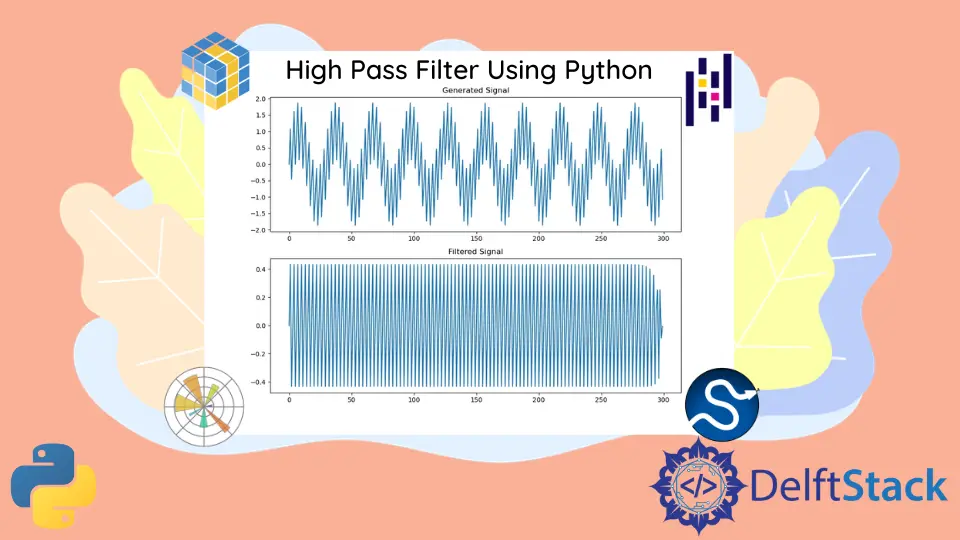
高通濾波器是限制低於預定義閾值頻率或截止頻率的訊號移動的濾波器。頻率大於或等於閾值的訊號暢通無阻地通過濾波器。此動作會衰減低頻訊號。高通濾波器過濾掉不需要的噪音,例如聲音。
在本文中,我們將學習如何使用 Python 實現高通濾波器。
使用 Python 實現高通濾波器
高通濾波器的實現使用了 4
個 Python 模組,即 numpy
、pandas
、scipy
和 matplotlib
。
numpy
模組是一個強大的 Python 模組,具有豐富的實用程式,可用於處理大型多維矩陣和陣列。這些實用程式有助於無縫地對矩陣執行復雜和直接的數學運算。這些實用程式針對輸入進行了優化和高度動態化。
要安裝最新版本的 numpy
模組,請使用以下 pip
命令之一。
pip install numpy
pip3 install numpy
pandas
模組是一個功能強大且靈活的開源資料分析和資料操作模組,用 Python 編寫。
要安裝 pandas
模組,請使用以下 pip
命令之一。
pip install pandas
pip3 install pandas
scipy
模組是用於科學和技術計算的開源 Python 模組。
要安裝 scipy
模組,請使用以下 pip
命令之一。
pip install scipy
pip3 install scipy
而且,matplotlib
模組是一個全面且優化的 Python 庫,用於資料的圖形視覺化。
要安裝最新版本的 matplotlib
模組,請使用以下 pip
命令之一。
pip install matplotlib
pip3 install matplotlib
現在我們已經完成了關於庫的簡要介紹,讓我們看看高通濾波器的 Python 程式碼。
import numpy as np
import pandas as pd
from scipy import signal
import matplotlib.pyplot as plt
def sine_generator(fs, sinefreq, duration):
T = duration
n = fs * T
w = 2.0 * np.pi * sinefreq
t_sine = np.linspace(0, T, n, endpoint=False)
y_sine = np.sin(w * t_sine)
result = pd.DataFrame({"data": y_sine}, index=t_sine)
return result
def butter_highpass(cutoff, fs, order=5):
nyq = 0.5 * fs
normal_cutoff = cutoff / nyq
b, a = signal.butter(order, normal_cutoff, btype="high", analog=False)
return b, a
def butter_highpass_filter(data, cutoff, fs, order=5):
b, a = butter_highpass(cutoff, fs, order=order)
y = signal.filtfilt(b, a, data)
return y
fps = 30
sine_fq = 10
duration = 10
sine_5Hz = sine_generator(fps, sine_fq, duration)
sine_fq = 1
duration = 10
sine_1Hz = sine_generator(fps, sine_fq, duration)
sine = sine_5Hz + sine_1Hz
filtered_sine = butter_highpass_filter(sine.data, 10, fps)
plt.figure(figsize=(20, 10))
plt.subplot(211)
plt.plot(range(len(sine)), sine)
plt.title("Generated Signal")
plt.subplot(212)
plt.plot(range(len(filtered_sine)), filtered_sine)
plt.title("Filtered Signal")
plt.show()
在上面的程式碼中,正弦頻率或 sine_fq
值應以赫茲或 Hz
為單位,持續時間或 duration
應以秒或 sec
為單位。生成的訊號是原始訊號,濾波後的訊號是高通濾波器形成的訊號。生成的圖表顯示了兩者之間的差異。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Vaibhav Vaibhav