在 Python 中查詢列表中的字串
Manav Narula
2023年1月30日
Python
Python List
-
在 Python 中使用
for
迴圈從列表中查詢包含特定子字串的元素 -
使用
filter()
函式從 Python 列表中查詢包含特定子字串的元素 - 使用正規表示式從 Python 列表中查詢包含特定子字串的元素
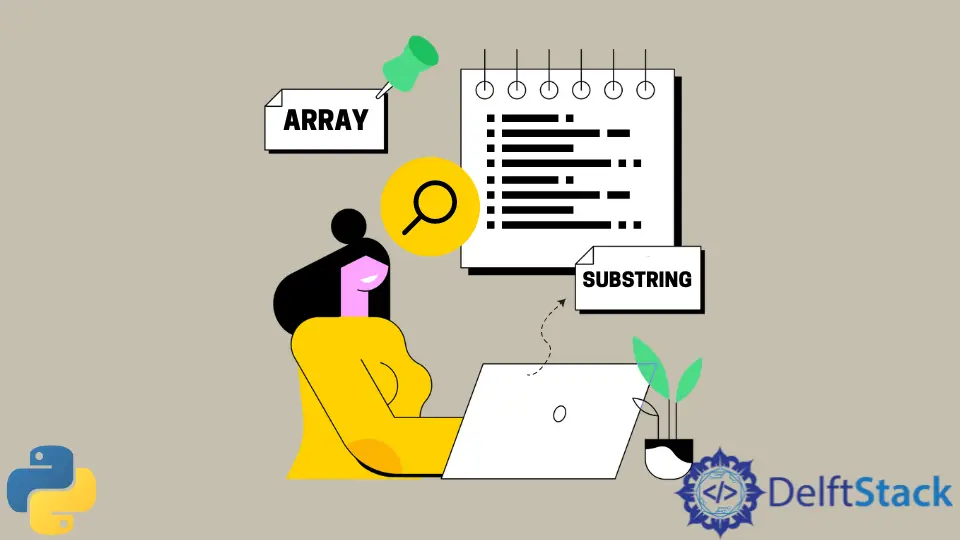
本教程將介紹如何從一個 Python 列表中查詢其中有特定子字串的元素。
我們將使用下面的列表並提取其中有 ack
的字串。
my_list = ["Jack", "Mack", "Jay", "Mark"]
在 Python 中使用 for
迴圈從列表中查詢包含特定子字串的元素
在這個方法中,我們遍歷列表並檢查子字串是否存在於某個元素中。如果子字串存在於該元素中,那麼我們就將其儲存在字串中。下面的程式碼顯示瞭如何操作。
str_match = [s for s in my_list if "ack" in s]
print(str_match)
輸出:
['Jack', 'Mack']
in
關鍵字檢查給定的字串,即本例中的"ack"
,是否存在於字串中。也可以用 __contains__
方法代替,它是字串類的一個神奇方法。例如:
str_match = [s for s in my_list if s.__contains__("ack")]
print(str_match)
輸出:
['Jack', 'Mack']
使用 filter()
函式從 Python 列表中查詢包含特定子字串的元素
filter()
函式借助函式從給定物件中檢索資料的子集。該方法將使用 lambda
關鍵字來定義過濾資料的條件。lambda
關鍵字在 Python 中建立了一個單行的 lambda
函式。請看下面的程式碼片段。
str_match = list(filter(lambda x: "ack" in x, my_list))
print(str_match)
輸出:
['Jack', 'Mack']
使用正規表示式從 Python 列表中查詢包含特定子字串的元素
正規表示式是一個字元序列,可以作為匹配模式來搜尋元素。要使用正規表示式,我們必須匯入 re
模組。在這個方法中,我們將使用 for
迴圈和 re.search()
方法,該方法用於返回與特定模式匹配的元素。下面的程式碼將解釋如何操作。
import re
pattern = re.compile(r"ack")
str_match = [x for x in my_list if re.search("ack", x)]
print(str_match)
輸出:
['Jack', 'Mack']
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Manav Narula
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn