Python 中的 __eq__ 方法
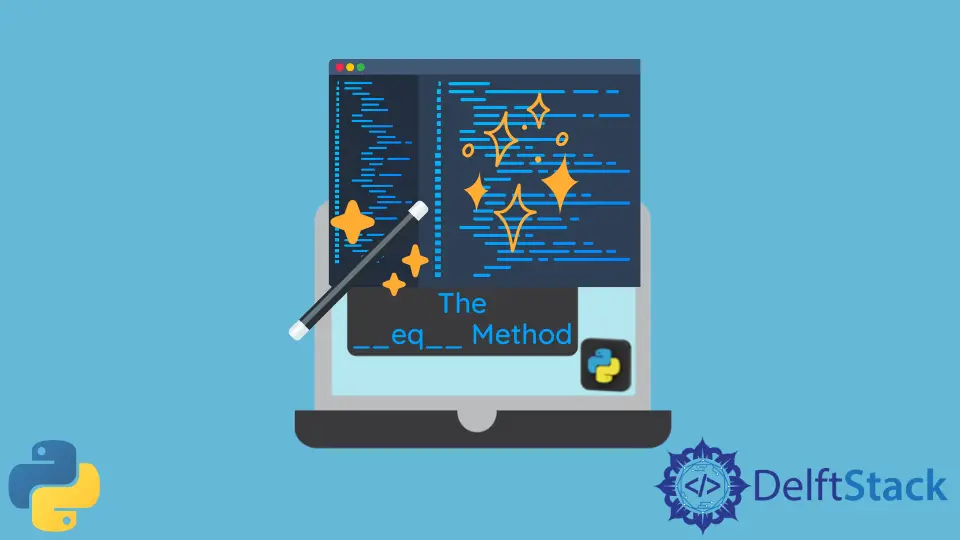
本指南將展示我們如何在 Python 中處理 __eq__()
方法。我們將組合不同的物件並學習這種特定方法的功能。讓我們深入瞭解它。
Python 中的 __eq__()
首先,__eq__()
是一個 dunder/magic 方法,如你所見,它前面和後面都有雙下劃線。每當你使用 ==
運算子時,都會呼叫此方法來比較兩個類例項。如果你沒有為 __eq__()
方法提供特定實現,Python 將使用 is
運算子進行比較。但是你如何指定這個方法呢?答案在於覆蓋這個函式。看看下面的程式碼。
# __eq__ this is called dunder/magic method in python
# class of student has constructor with two arguments
class Student:
def __init__(self, name, roll):
self.name = name
self.roll = roll
# object one of class student
s1 = Student("David", 293)
# object two of class student
s2 = Student("David", 293)
# Applying equality function two objects
print(s1 == s2)
輸出:
False
如你所見,我們建立了一個名為 Student
的類,並將其兩個引數設定為 name
和 roll
。後來,你建立了兩個具有相同 Student
類資料的例項。正如我們在上面的程式碼中所做的那樣比較這兩個例項將給出一個 false
輸出。
儘管這些例項具有相同的資料,但它們在比較後並不相等。這是因為 ==
運算子比較了 case 的地址,這是不同的。你可以通過執行以下程式碼和上面的程式碼來看到這一點。
# Both objects have different ids thats why the s1==s2 is false
print(id(s1))
print(id(s2))
輸出:
1991016021344 //(it will vary)
1991021345808 //(it will vary)
但是,如果我們要建立一個例項,然後在另一個示例中儲存該例項,如下所示,它們的地址將是相同的。看一看。
# object one of class student
s1 = Student("Ahsan", 293)
s3 = s1
# In this case we have same id for s1 and s3
print(s1 == s3)
print(id(s1))
print(id(s3))
輸出:
True
1913894976432 //(it will vary)
1913894976432 //(it will vary)
它會給你 true
作為輸出。那麼,我們如何根據資料比較兩個例項呢?我們需要實現 dunder 方法並像下面這樣覆蓋它。
# class of student has constructor with two arguments and a modified dunder method of __eq__
class Student:
def __init__(self, name, roll):
self.name = name
self.roll = roll
# implementing the dunder method
def __eq__(self, obj):
# checking both objects of same class
if isinstance(obj, Student):
if self.roll == obj.roll:
return True
else:
return False
# object of class student
s4 = Student("David", 293)
# other object of class student
s5 = Student("David", 293)
# Applying equality function two objects
print(s4 == s5)
# Both objects have different ids, but the s4==s5 is true because we override the __eq__ function it will give true when the roll number is some
print(id(s4))
print(id(s5))
# object of class student
s6 = Student("David", 293)
# other object of class student
s7 = Student("David", 178)
# Applying equality function two objects will give us False because the roll is different
print(s6 == s7)
輸出:
True
1935568125136 //(it will vary)
1935568124656 //(it will vary)
False
現在,我們通過在裡面放置一個檢查 roll
是否相同的 if
條件來覆蓋 __eq__()
方法。這就是你在 Python 中覆蓋 __eq__()
方法的方式。
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn