Método __eq__ en Python
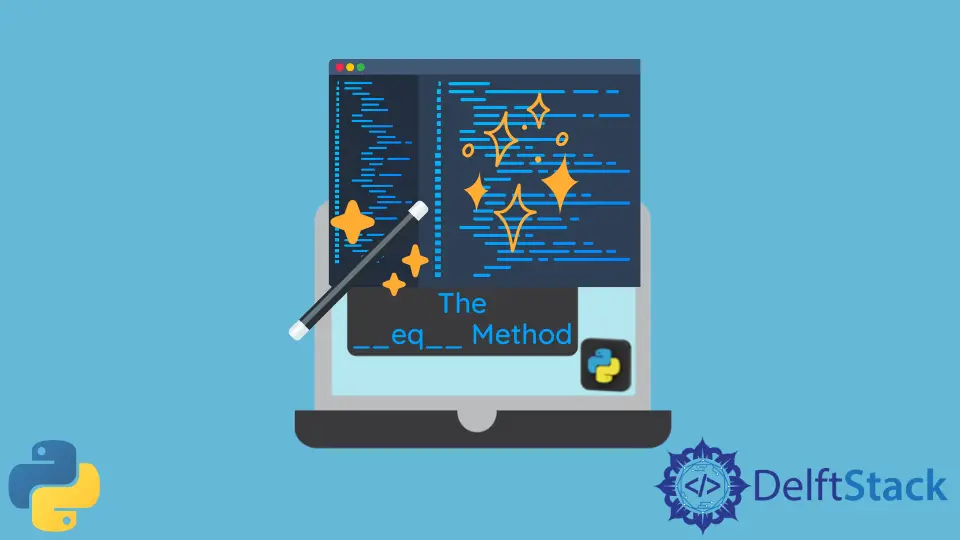
Esta guía mostrará cómo manejamos el método __eq__()
en Python. Complejaremos diferentes objetos y aprenderemos la funcionalidad de este método en particular. Vamos a sumergirnos en eso.
__eq__()
en Python
Primero, __eq__()
es un método dunder/magic, como puede ver, está precedido y seguido por guiones bajos dobles. Cada vez que usa el operador ==
, este método ya se llama para comparar dos instancias de clase. Python usará el operador is
para comparar si no proporciona una implementación específica para el método __eq__()
. Pero, ¿cómo se especifica este método? La respuesta a eso radica en anular esta función. Echa un vistazo al siguiente código.
# __eq__ this is called dunder/magic method in python
# class of student has constructor with two arguments
class Student:
def __init__(self, name, roll):
self.name = name
self.roll = roll
# object one of class student
s1 = Student("David", 293)
# object two of class student
s2 = Student("David", 293)
# Applying equality function two objects
print(s1 == s2)
Producción :
False
Como puede ver, creamos una clase llamada Student
e hicimos dos de sus argumentos como name
y roll
. Más tarde, creó dos instancias con los mismos datos de la clase Student
. Comparar estas dos instancias como lo hemos hecho en el código anterior dará un resultado false
.
A pesar de que estas instancias tienen los mismos datos, no son iguales una vez comparadas. Es porque el operador ==
compara la dirección de los casos, que es diferente. Puede verlo ejecutando el siguiente código y el código anterior.
# Both objects have different ids thats why the s1==s2 is false
print(id(s1))
print(id(s2))
Producción :
1991016021344 //(it will vary)
1991021345808 //(it will vary)
Pero si tuviéramos que crear una instancia y luego guardar esa instancia en otro ejemplo como el siguiente, sus direcciones serían las mismas. Echar un vistazo.
# object one of class student
s1 = Student("Ahsan", 293)
s3 = s1
# In this case we have same id for s1 and s3
print(s1 == s3)
print(id(s1))
print(id(s3))
Producción :
True
1913894976432 //(it will vary)
1913894976432 //(it will vary)
Le daría true
como salida. Entonces, ¿cómo podemos comparar las dos instancias según los datos? Necesitamos implementar el método dunder y anularlo de la siguiente manera.
# class of student has constructor with two arguments and a modified dunder method of __eq__
class Student:
def __init__(self, name, roll):
self.name = name
self.roll = roll
# implementing the dunder method
def __eq__(self, obj):
# checking both objects of same class
if isinstance(obj, Student):
if self.roll == obj.roll:
return True
else:
return False
# object of class student
s4 = Student("David", 293)
# other object of class student
s5 = Student("David", 293)
# Applying equality function two objects
print(s4 == s5)
# Both objects have different ids, but the s4==s5 is true because we override the __eq__ function it will give true when the roll number is some
print(id(s4))
print(id(s5))
# object of class student
s6 = Student("David", 293)
# other object of class student
s7 = Student("David", 178)
# Applying equality function two objects will give us False because the roll is different
print(s6 == s7)
Producción :
True
1935568125136 //(it will vary)
1935568124656 //(it will vary)
False
Ahora, estamos anulando el método __eq__()
poniendo una condición if
dentro que verifica si el roll
es el mismo o no. Así es como anula un método __eq__()
en Python.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn