在 Python 中建立管道
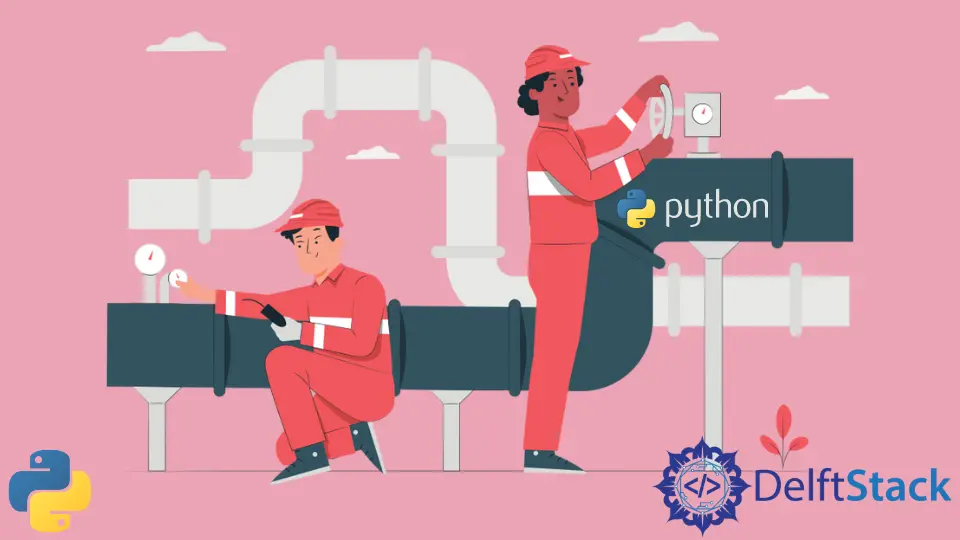
本文將演示為 sklearn 資料集和自定義資料集建立用於機器學習的 Python 管道。
在 Python 中為自定義資料集建立管道
我們需要兩個匯入包來建立 Python 管道,Pandas 用於生成 DataFrame,sklearn 用於管道。除此之外,我們還部署了另外兩個子包,Pipeline 和 Linear Regression。
以下是使用的所有軟體包的列表。
import pandas as pd
from sklearn.pipeline import Pipeline
from sklearn.linear_model import LinearRegression
形成具有方程值的資料集
該程式旨在建立一個管道,當有足夠的後續值訓練模型時,該管道將預測方程的結果值。
這裡使用的等式是:
c = a + 3*\sqrt[3]{b}
我們使用線性方程的值建立一個 Pandas 資料集。
df = pd.DataFrame(columns=["col1", "col2", "col3"], val=[[15, 8, 21], [16, 27, 25]])
將資料拆分為訓練集和測試集
每個機器學習模型都需要將資料分成不等的兩半。分離後,我們使用這兩組來訓練和測試模型。
比較顯著的部分用於訓練,另一部分用於測試模型。
在下面的程式碼片段中,前 8 個值用於訓練模型,其餘用於測試。
learn = df.iloc[:8]
evaluate = df.iloc[8:]
scikit-learn 管道通過將值輸入管道然後給出結果來工作。值通過兩個輸入變數 - X 和 y 提供。
在所使用的等式中,c 是 a 和 b 的函式。因此,為了使管道適合線性迴歸模型中的值,我們將 a、b 值轉換為 X 值和 y 中的 c 值。
重要的是要注意 X 和 y 是學習和評估變數。因此,我們將變數 a 和 b 傳遞給訓練函式,並將變數 c 分配給測試函式。
learn_X = learn.drop("col3", axis=1)
learn_y = learn.col3
evaluate_X = evaluate.drop("col3", axis=1)
evaluate_y = evaluate.col3
在上面的程式碼中,當值被輸入到 learn_X
變數中時,Pandas drop()
函式會刪除列 c 的值。在 learn_y
變數中,傳輸 c 列的值。
axis = 1
代表列,而 0 值代表行。
建立 Python 管道並在其中擬合值
我們使用 Pipeline 函式在 Python 中建立一個管道。我們必須在使用前將其儲存在變數中。
在這裡,為此目的宣告瞭一個名為 rock
的變數。
在管道內部,我們必須給出它的名稱和要使用的模型 - ('Model for Linear Regression', LinearRegression())
。
rock = Pipeline(steps=[("Model for Linear Regression", LinearRegression())])
完成在 Python 中建立管道的步驟後,需要為其擬合學習值,以便線性模型可以使用提供的值訓練管道。
rock.fit(learn_X, learn_y)
管道訓練完成後,變數 evaluate_X
通過 pipe1.predict()
函式預測以下值。
預測值儲存在一個新變數 evalve
中並列印出來。
evalve = rock.predict(evaluate_X)
print(f"\n{evalve}")
讓我們把所有東西放在一起來觀察管道是如何建立的以及它的效能。
import pandas as pd
# import warnings
# warnings.filterwarnings('ignore')
from sklearn.pipeline import Pipeline
from sklearn.linear_model import LinearRegression
df = pd.DataFrame(
columns=["col1", "col2", "col3"],
data=[
[15, 8, 21],
[16, 27, 25],
[17, 64, 29],
[18, 125, 33],
[19, 216, 37],
[20, 343, 41],
[21, 512, 45],
[22, 729, 49],
[23, 1000, 53],
[24, 1331, 57],
[25, 1728, 61],
[26, 2197, 65],
],
)
learn = df.iloc[:8]
evaluate = df.iloc[8:]
learn_X = learn.drop("col3", axis=1)
learn_y = learn.col3
evaluate_X = evaluate.drop("col3", axis=1)
evaluate_y = evaluate.col3
print("\n step: Here, the pipeline is formed")
rock = Pipeline(steps=[("Model for Linear Regression", LinearRegression())])
print("\n Step: Fitting the data inside")
rock.fit(learn_X, learn_y)
print("\n Searching for outcomes after evaluation")
evalve = rock.predict(evaluate_X)
print(f"\n{evalve}")
輸出:
"C:/Users/Win 10/pipe.py"
step: Here, the pipeline is formed
Step: Fitting the data inside
Searching for outcomes after evaluation
[53. 57. 61. 65.]
Process finished with exit code 0
正如我們所看到的,管道預測了確切的值。
在 Python 中為 Scikit-Learn 資料集建立管道
此示例演示如何在 Python 中為 Scikit 學習資料集建立管道。在大型資料集上執行管道操作與小型資料集略有不同。
管道在處理大型資料集時需要使用額外的模型來清理和過濾資料。
下面是我們需要的匯入包。
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.preprocessing import StandardScaler
from sklearn.pipeline import make_pipeline
from sklearn import datasets
使用了來自 sklearn 的資料集。它有多個列和值,但我們將專門使用兩列 - 資料和目標。
載入資料集並將其拆分為訓練集和測試集
我們將把資料集載入到變數 bc
中,並將各個列的值儲存在變數 X 和 y 中。
bc = datasets.load_breast_cancer()
X = bc.data
y = bc.target
載入資料集後,我們定義學習和評估變數。資料集必須分成訓練集和測試集。
a_learn, a_evaluate, b_learn, b_evaluate = train_test_split(
X, y, test_size=0.40, random_state=1, stratify=y
)
我們將資料集分配到 4 個主要變數 - X_learn
、X_evaluate
、y_learn
和 y_evaluate
。與之前的程式不同,這裡的分配是通過 train_test_split()
函式完成的。
test_size=0.4
指示函式保留 40% 的資料集用於測試,剩下的一半用於訓練。
random_state=1
確保對資料集進行統一拆分,以便每次執行函式時預測都給出相同的輸出。每次執行函式時,random_state=0
都會提供不同的結果。
stratify=y
確保在樣本大小中使用相同的資料大小,如為引數分層提供的那樣。如果有 15% 的 1 和 85% 的 0,stratify
將確保系統在每次隨機拆分中都有 15% 的 1 和 85% 的 0。
建立 Python 管道並在其中擬合值
pipeline = make_pipeline(StandardScaler(),
RandomForestClassifier (n_estimators=10, max_features=5, max_depth=2, random_state=1))
其中,
make_pipeline()
是一個用於建立管道的 Scikit-learn 函式。Standard scaler()
從平均值中刪除值並將它們分配到其單位值。RandomForestClassifier()
是一個決策模型,它從資料集中獲取一些樣本值,用每個樣本值建立一個決策樹,然後預測每個決策樹的結果。然後,模型對預測結果的準確性進行投票,投票最多的結果被選為最終預測。n_estimators
表示在投票前要建立的決策樹的數量。max_features
決定執行節點分裂時將形成多少個隨機狀態。max_depth
表示樹的節點有多深。
建立管道後,對值進行擬合,並預測結果。
pipeline.fit(a_learn, b_learn)
y_pred = pipeline.predict(a_evaluate)
讓我們看看完整的程式。
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.ensemble import RandomForestClassifier
from sklearn.pipeline import make_pipeline
from sklearn import datasets
bc = datasets.load_breast_cancer()
X = bc.data
y = bc.target
a_learn, a_evaluate, b_learn, b_evaluate = train_test_split(
X, y, test_size=0.40, random_state=1, stratify=y
)
# Create the pipeline
pipeline = make_pipeline(
StandardScaler(),
RandomForestClassifier(
n_estimators=10, max_features=5, max_depth=2, random_state=1
),
)
pipeline.fit(a_learn, b_learn)
y_pred = pipeline.predict(a_evaluate)
print(y_pred)
輸出:
"C:/Users/Win 10/test_cleaned.py"
[0 0 0 1 1 1 1 0 0 1 1 0 1 0 0 1 0 0 1 0 1 1 0 0 0 1 0 0 0 1 1 1 1 1 1 1 1
1 1 0 1 1 1 1 0 1 1 0 0 0 1 1 1 0 1 1 1 0 1 1 1 1 1 1 0 0 1 1 1 1 0 1 1 1
1 1 0 1 1 0 1 0 1 1 1 1 0 0 0 1 0 0 1 0 1 1 1 0 1 1 0 1 0 1 1 1 1 0 1 1 1
1 1 0 0 1 1 0 0 1 0 0 0 1 0 1 0 0 1 0 1 0 1 0 1 0 1 1 0 0 1 1 1 1 1 0 1 1
0 0 1 0 1 0 0 1 1 0 1 0 0 0 1 0 1 1 1 0 0 1 0 1 1 1 1 0 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 0 1 1 1 1 1 1 0 1 1 1 1 1 0 1 0 0 1 1 1 0 1 0 0 1 1 0 1 1
1 1 1 1 1 0]
Process finished with exit code 0