在 Python 中計算兩個列表的點積
-
在 Python 中使用
sum()
和zip()
函式計算點積 -
在 Python 中使用
map()
和mul()
函式計算點積 -
在 Python 中使用
more_itertools.dotproduct
計算點積 - 在 Python 中使用 NumPy 計算點積
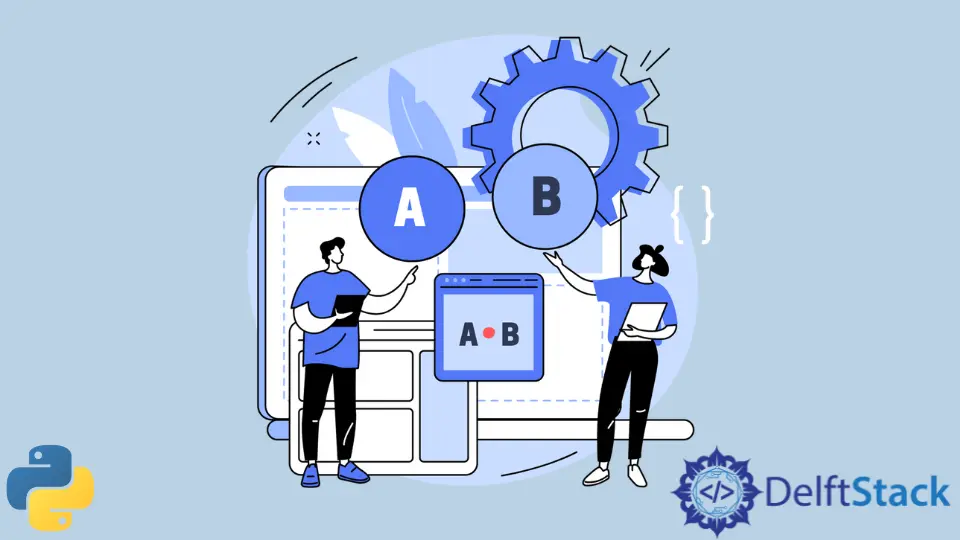
點積是一種數學運算,也稱為標量積。點積是一個代數表示式,它接受兩個相等長度的序列並返回一個數字作為結果。
在 Python 中使用 sum()
和 zip()
函式計算點積
我們可以使用 zip()
函式和 sum()
函式計算等長列表的點積。
zip
函式通過組合來自兩個可迭代物件的元組序列中的元素來返回一個 zip 物件。另一方面,sum
函式返回可迭代物件(例如列表)中專案的總和。
由於點積在數學上涉及數字序列中元素的一系列總和和乘積,我們可以使用這兩者的組合來計算兩個列表的點積。
num1 = [2, 4, 6, 8, 10]
num2 = [10, 20, 30, 40, 50]
print(sum([i * j for (i, j) in zip(num1, num2)]))
輸出:
1100
在 Python 中使用 map()
和 mul()
函式計算點積
Python 中的 operator
模組提供了一組函式來匯入和使用 Python 中的內部運算子來執行各種操作。
這些運算包括邏輯運算、序列運算、數學運算和物件比較。mul()
函式執行物件與一系列數字(例如 DataFrame)的逐元素乘法。
另一方面,map()
函式是一個內建函式,它允許我們將某個函式應用於可迭代物件的所有元素。最後,我們還將使用 sum()
函式計算兩個數值列表的乘積之和,如下面的程式碼所示。
from operator import mul
num1 = [2, 4, 6, 8, 10]
num2 = [10, 20, 30, 40, 50]
print(sum(map(mul, num1, num2)))
輸出:
1100
在 Python 中使用 more_itertools.dotproduct
計算點積
Python more_itertools
是一個 Python 庫,它提供了優雅的函式來處理 Python 中的可迭代物件。more_itertools
庫提供的函式允許我們在視窗、組合和包裝等其他操作中對可迭代物件進行分組和選擇。
more_itertools
庫不僅為複雜的迭代提供解決方案;它也更優雅和記憶體效率更高。使用 more_itertools.product()
函式,我們可以計算列表中數字序列的點積,如下所示。
import more_itertools as mit
num1 = [2, 4, 6, 8, 10]
num2 = [10, 20, 30, 40, 50]
print(mit.dotproduct(num1, num2))
輸出:
1100
在 Python 中使用 NumPy 計算點積
NumPy 是一個科學的 Python 包,它允許我們處理多維物件,例如陣列和矩陣。
NumPy 速度快但效率更高,因為我們可以使用很少的程式碼實現很多目標。我們可以無縫地使用陣列,而無需顯式索引和迴圈遍歷其向量化程式碼。
下面是一個解決方案,它使用 for 迴圈以及乘積和加法運算子的組合來計算兩個列表的點積。
num1 = [2, 4, 6, 8, 10]
num2 = [10, 20, 30, 40, 50]
dot_product = 0
for x, y in zip(num1, num2):
dot_product = dot_product + x * y
print("The dot product of the two lists is: ", dot_product)
輸出:
The dot product of the two lists is: 1100
儘管此解決方案計算點積,但 NumPy 提供了一種更優雅的替代方案,無需編寫任何迴圈。
使用 numpy.dot()
方法,我們可以輕鬆計算兩個列表中數字序列的點積。這個解決方案是精確的,因此不容易出錯,可以在下面的程式碼中實現。
import numpy as np
num1 = [2, 4, 6, 8, 10]
num2 = [10, 20, 30, 40, 50]
dot_product = np.dot(num1, num2)
print("The dot product of the two lists is: ", dot_product)
輸出:
The dot product of the two lists is: 1100
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn