Tkinter Photoimage
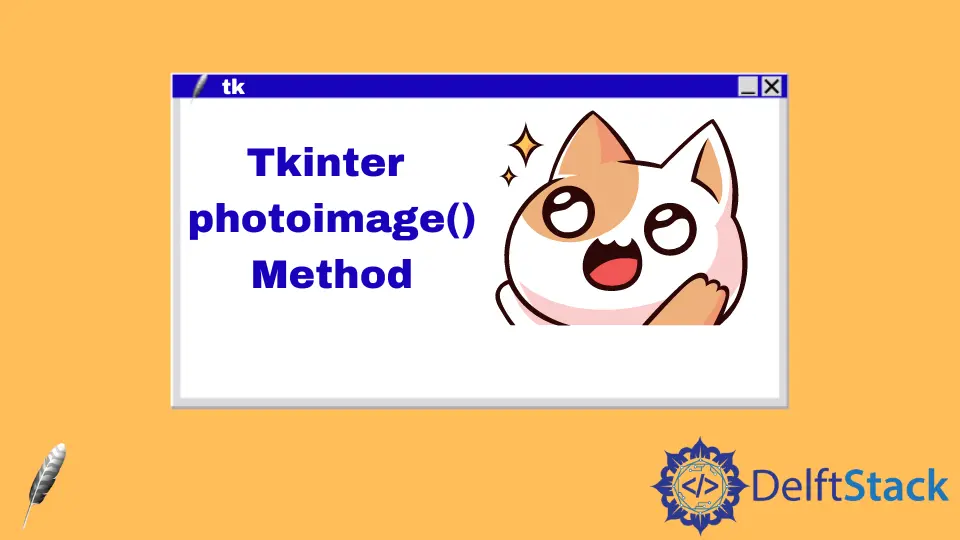
在本文中,你將瞭解 photoimage()
方法以及如何在 Tkinter 中使用它。Tkinter 在 Python 程式設計師中非常流行,因為它可以讓你用 Python 做一些有趣的事情。
你可以使用此方法將圖片新增到按鈕、標籤或畫布。它使應用程式更具互動性、美觀性和實用性。
在 Tkinter 中使用 photoimage()
方法顯示影象
PhotoImage
只能顯示元件支援的影象。如果它們不能顯示在元件上,它將返回錯誤。
顯示影象的一般元件是標籤、按鈕和畫布。
我們需要安裝 Pillow
模組以使用 PhotoImage()
方法,因此使用此命令安裝此模組。
pip install Pillow
from tkinter import *
from PIL import ImageTk, Image
# CreateTK object
gui = Tk()
# Create canvas object
cnv = Canvas(gui, width=400, height=400)
cnv.pack()
# create an object of PhotoImage
photo = ImageTk.PhotoImage(Image.open("lion.png"))
cnv.create_image(30, 30, anchor=NW, image=photo)
gui.mainloop()
我們將 ImageTk
和 Image
類匯入此程式碼。ImageTk
類有幾個方法;一種是幫助顯示影象的 PhotoImage()
方法。
我們使用了 Image
類中的 open()
方法。open()
方法包含你要顯示的檔案路徑,並接受多種格式,如 jpg
、png
、gif
等。
在這種情況下,canvas
元件用於顯示影象;在下一個示例中,我們將使用 Label
元件來顯示影象。
在 Tkinter 中使用 resize()
方法調整影象大小
有時我們需要在 GUI 中調整影象的大小;幸運的是,Tkinter 提供了幫助調整影象大小的 resize()
方法。
from tkinter import *
from PIL import Image, ImageTk
gui = Tk()
width = 100
height = 100
photo = Image.open("lion.png")
photo = photo.resize((width, height), Image.ANTIALIAS)
# create an object of PhotoImage
photoImg = ImageTk.PhotoImage(photo)
photo_label = Label(gui, image=photoImg)
photo_label.pack()
gui.mainloop()
當我們使用 resize()
方法時,我們不能直接在 PhotoImage()
中傳遞影象,因為在 PhotoImage()
方法中傳遞影象後我們將無法使用 resize()
方法. resize()
方法有兩個引數,寬度和高度。
調整大小的影象已通過此程式碼的 PhotoImage()
方法。Label
元件使用影象屬性顯示影象。
在 Tkinter 中放大照片
在此程式碼中,你將學習如何使用 Tkinter 中的縮放功能。
ZoomIn()
函式有助於放大影象。zoom_in
變數限制建立影象物件,直到第二次執行。
img1 = img1._PhotoImage__photo.zoom(max_num)
由於我們使用的是全域性影象物件,因此我們通過 zoom_in
變數設定 if
條件。_PhotoImage__photo.zoom()
方法有助於放大影象;此方法需要一個整數。
我們在這個方法中傳遞了 max_num
變數;當 ZoomIn()
函式呼叫時,此變數將加 1。
讓我們演示一下 ZoomOut()
函式,該函式的工作原理與 ZoomIn()
函式相同。
img2 = img2._PhotoImage__photo.subsample(min_num)
_PhotoImage__photo.subsample()
方法使其不同於 ZoomIn()
函式。
這種方法有助於縮小影象;該方法採用整數。簡而言之,當 ZoomOut()
函式呼叫影象時,它會被縮小。
from tkinter import *
from PIL import Image as PIL_image, ImageTk as PIL_imagetk
window = Tk()
zoom_in = 1
zoom_out = 1
max_num = 1
min_num = 1
def ZoomIn():
global img1
global img2
global zoom_in
global max_num
# A condition which will let create image object in second execution
if zoom_in > 1:
img1 = PIL_imagetk.PhotoImage(file="lion.png")
max_num += 1
if max_num < 3:
img1 = img1._PhotoImage__photo.zoom(max_num)
label.config(image=img1)
else:
img1 = img1._PhotoImage__photo.zoom(3)
label.config(image=img1)
max_num = 1
img2 = PIL_imagetk.PhotoImage(file="lion.png")
zoom_in += 1
def ZoomOut():
global img2
global img1
global zoom_out
global min_num
# A condition which will let create image object in second execution
if zoom_out > 1:
img2 = PIL_imagetk.PhotoImage(file="lion.png")
min_num += 1
if min_num < 3:
img2 = img2._PhotoImage__photo.subsample(min_num)
label.config(image=img2)
else:
img2 = img2._PhotoImage__photo.subsample(3)
label.config(image=img2)
min_num = 1
img1 = PIL_imagetk.PhotoImage(file="lion.png")
zoom_out += 1
img1 = PIL_imagetk.PhotoImage(file="lion.png")
img2 = PIL_imagetk.PhotoImage(file="lion.png")
label = Label(window, image=img1)
label.pack()
btn = Button(window, text="zoom in", command=ZoomIn)
btn.pack()
btn2 = Button(window, text="zoom out", command=ZoomOut)
btn2.pack()
window.mainloop()
輸出:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn