Pandas 中如何從文字檔案載入資料
Asad Riaz
2023年1月30日
-
使用
read_csv()
方法從文字檔案載入資料 -
使用
read_fwf()
方法將寬度格式的文字檔案載入到 Pandas dataframe 中 -
使用
read_table()
方法將文字檔案載入到 Pandas dataframe 中
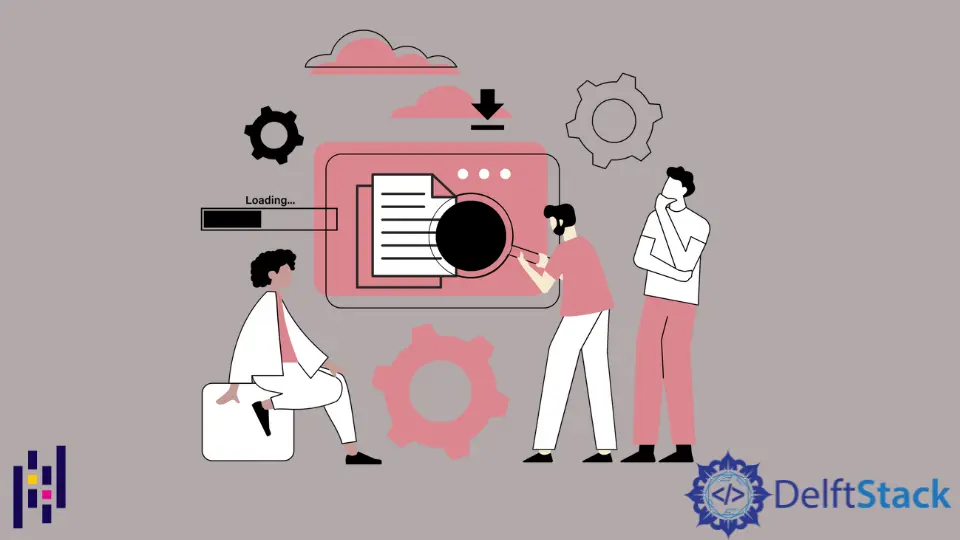
我們將介紹 Pandas Dataframe 從 txt 檔案中載入資料的方法。我們還將介紹可用的選項。
首先,我們將建立一個名為 sample.txt
的簡單文字檔案,並在檔案中新增以下幾行:
45 apple orange banana mango
12 orange kiwi onion tomato
我們需要將其儲存到執行 Python 指令碼的目錄中。
使用 read_csv()
方法從文字檔案載入資料
read_csv()
是將文字檔案轉換為 Pandas Dataframe 的最佳方法。我們需要設定 header=None
,因為上面建立的檔案中沒有任何 header
。如果我們希望將空值替換為 NaN
,我們還可以在方法內部設定 keep_default_na=False
。
示例程式碼:
# python 3.x
import pandas as pd
df = pd.read_csv("sample.txt", sep=" ", header=None)
print(df)
輸出:
0 1 2 3 4
0 45 apple orange banana mango
1 12 orange kiwi onion tomato
我們將設定 sep =" "
因為值之間用一個空格隔開。同樣,如果我們從逗號分隔的檔案中讀取資料,則可以設定 sep =","
。將 sample.txt
中的空格替換為 ,
,然後將 sep =" "
替換為 sep =","
,然後執行程式碼。
Sample.txt
45,apple,orange,banana,mango
12,orange,kiwi,,tomato
程式碼:
# python 3.x
import pandas as pd
df = pd.read_csv("sample.txt", sep=",", header=None)
print(df)
輸出:
0 1 2 3 4
0 45 apple orange banana mango
1 12 orange kiwi NaN tomato
使用 read_fwf()
方法將寬度格式的文字檔案載入到 Pandas dataframe 中
當我們有一個寬度格式化
文字檔案時,read_fwf()
將非常有用。我們不能使用 sep
,因為不同的值可能具有不同的分隔符。考慮以下文字檔案:
Sample.txt
45 apple orange banana mango
12 orange kiwi onion tomato
如果我們看一下 Sample.text
,我們將看到分隔符 delimiter
對於每個值都不相同。所以 read_fwf()
將能達到我們正確讀取這個檔案資料的目的。
程式碼:
# python 3.x
import pandas as pd
df = pd.read_fwf("sample.txt", header=None)
print(df)
輸出:
0 1 2 3 4
0 45 apple orange banana mango
1 12 orange kiwi onion tomato
使用 read_table()
方法將文字檔案載入到 Pandas dataframe 中
read_table()
是將資料從文字檔案載入到 Pandas DataFrame 的另一種方法。
Sample.txt:
45 apple orange banana mango
12 orange kiwi onion tomato
程式碼:
# python 3.x
import pandas as pd
df = pd.read_table("sample.txt", header=None, sep=" ")
print(df)
輸出:
0 1 2 3 4
0 45 apple orange banana mango
1 12 orange kiwi onion tomato