PHP 中的 this 和 self
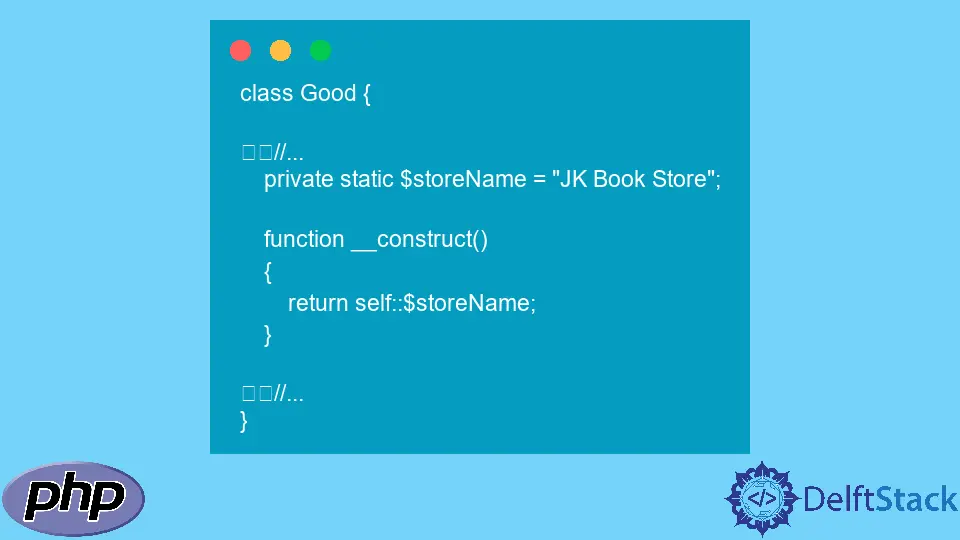
this
和 self
是物件導向程式設計 (OOP) 的元件屬性。OOP 是 PHP 的一個元件特性,它是一種程式設計過程,而不是程序式程式設計,我們在其中編寫執行資料操作的函式。
使用 OOP,我們可以建立同時具有資料和函式(方法)的物件。
不過,OOP 提供了一種更快、更全面的方式來使用任何支援它的語言(包括 PHP)進行編碼。某些特性或屬性可能很複雜,例如 this
和 self
,並且會使使用 OOP 變得不好玩。
本文將討論 this
和 self
有何不同以及如何在 PHP 中使用它們。
PHP 中的 OOP
OOP 為你的 PHP 程式提供了清晰的結構,並允許你遵循不要重複自己
的流行原則。
類和方法是 PHP 中 OOP 的重要組成部分,可以使用以下程式碼片段輕鬆建立。
<?php
class Good {
public $propertyOne;
public $propertyTwo;
private $propertyThree;
function methodOne($propertyOne) {
//
}
}
?>
$propertyOne
、$propertyTwo
、$propertyThree
是類 Good
的屬性,methodOne()
是一個方法。
我們可以使用此程式碼片段建立物件、OOP 的整個目標以及類和方法的原因。
$goodOne = new Good();
PHP 中的 this
和 self
為了擴充套件當前程式碼,我們可以建立一個方法來設定類 Good
的 $propertyOne
。
class Good {
//...
function setGoodName($propertyOne) {
$this->propertyOne = $propertyOne;
}
function showGoodName() {
return $this->propertyOne;
}
}
$this
關鍵字指的是當前物件,並且僅在類中的方法內部可用。因此,如果我們想在 PHP 程式碼中使用 $this
,它必須在我們類的方法中。
在程式碼片段的情況下,$this
關鍵字指向當前物件,以允許我們在 Good
類中呼叫 $propertyOne
。
讓我們利用我們建立的方法。
$book = new Good();
$book->setGoodName("PHP for Dummies");
echo $book->showGoodName();
程式碼片段的輸出如下。
PHP for Dummies
讓我們進一步擴充套件我們的 PHP 程式碼,併為類 Good
新增一個關於靜態儲存 Name
的屬性,將其設為私有,並將該屬性返回給 constructor。
class Good {
//...
private static $storeName = "JK Book Store";
function __construct()
{
return self::$storeName;
}
//...
}
self
關鍵字指的是當前類,並允許我們訪問類和靜態變數,如上面的程式碼片段所示。self
關鍵字使用範圍解析運算子::
訪問或引用靜態類成員。
因此,self
和 $this
之間的一個很大區別是 self
訪問靜態或類變數或方法,而 $this
訪問非靜態和物件變數和方法。
因此,在使用 OOP 時,要知道我們在物件(類的例項)內部使用 $this
,而對於類和靜態屬性使用 self
。
完整原始碼:
<?php
class Good {
// properties
public $propertyOne;
public $propertyTwo;
private $propertyThree;
private static $storeName = "JK Book Store";
// methods
function __construct()
{
return self::$storeName;
}
function setGoodName($propertyOne) {
$this->propertyOne = $propertyOne;
}
function showGoodName() {
return $this->propertyOne;
}
}
// creating a object
$book = new Good();
$book->setGoodName("PHP for Dummies");
echo $book->showGoodName();
?>
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn