在 Node.js 中解析 Json
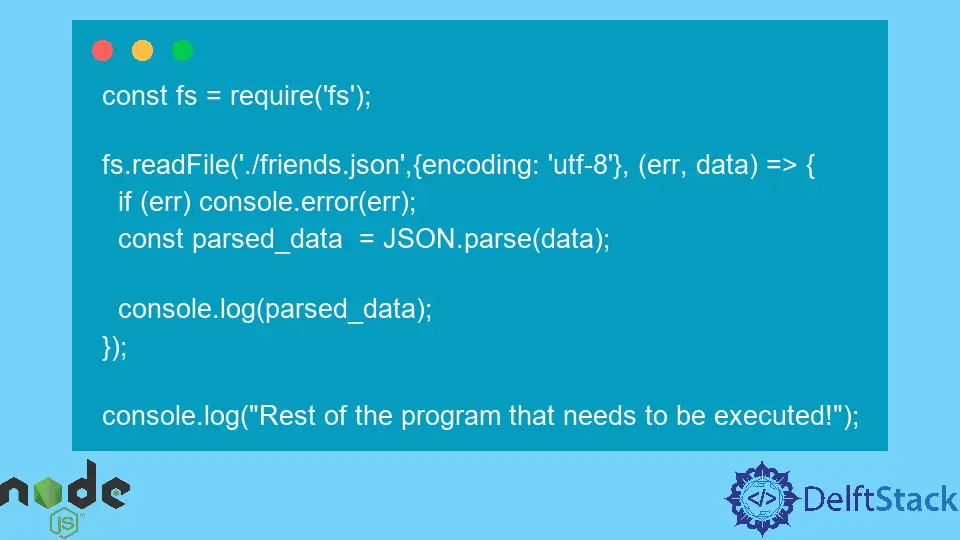
在本節中,我們將學習在 Node js 中同步解析 JSON。解析 JSON 資料可能被稱為以你當前可能使用的特定語言解釋 JSON 資料的過程。
JSON 資料通常儲存在鍵值對中,作為用引號括起來的字串值,同時仍然遵循 JSON 規範。從伺服器接收或由 API 返回的 JSON 資料通常採用字串格式。在這種原始格式中,JSON 資料不易讀取和訪問,因此需要轉換為更使用者友好的形式。
我們可以使用三種主要方法來解析 Node js 中的 JSON 檔案。對於本地檔案,即那些駐留在本地計算機儲存中的檔案,我們可以使用 require()
函式將模組載入到 Node js 環境中。但是,此方法是同步的,這意味著該程式在當前檔案完全解析之前不會執行任何其他操作。
[
{
"id": 0,
"name": "Maribel Andrews"
},
{
"id": 1,
"name": "Gilliam Mcdonald"
},
{
"id": 2,
"name": "Lisa Wright"
}
]
const friends = require("./friends.json");
console.log(friends);
樣本輸出:
[
{ id: 0, name: 'Maribel Andrews' },
{ id: 1, name: 'Gilliam Mcdonald' },
{ id: 2, name: 'Lisa Wright' }
]
該程式不適用於動態程式,但適用於靜態檔案。一旦進行了任何更改,可能會更改資料的程式將要求我們重新解析資料。這不是解析 JSON 檔案的最佳方式。
或者,我們可以使用 fs
模組和 fs.readFileSync()
載入 JSON 檔案,而不是將其作為模組載入。fs 模組是執行檔案操作(例如讀取檔案、重新命名和刪除)的一種更有效的方式。fs.readFileSync()
是一個允許我們同步讀取檔案及其內容的介面。
[
{
"id": 0,
"name": "Maribel Andrews"
},
{
"id": 1,
"name": "Gilliam Mcdonald"
},
{
"id": 2,
"name": "Lisa Wright"
}
]
const fs = require("fs");
const friends = fs.readFileSync("./friends.json");
console.log(friends);
樣本輸出:
<Buffer 5b 0d 0a 20 20 20 20 7b 0d 0a 20 20 20 20 20 20 22 69 64 22 3a 20 30 2c 0d 0a 20 20 20 20 20 20 22 6e 61 6d 65 22 3a 20 22 4d 61 72 69 62 65 6c 20 41 ... 144 more bytes>
雖然我們已成功載入 JSON 檔案,但我們尚未指定編碼格式。readFileSync()
方法在未指定編碼引數時(即為 null)返回原始緩衝區。文字檔案的編碼格式通常是 utf-8
。
const fs = require("fs");
const friends = fs.readFileSync("./friends.json", {encoding: 'utf-8'});
console.log(friends);
樣本輸出:
[
{
"id": 0,
"name": "Maribel Andrews"
},
{
"id": 1,
"name": "Gilliam Mcdonald"
},
{
"id": 2,
"name": "Lisa Wright"
}
]
現在我們已經成功讀取了 JSON 檔案,我們可以將 JSON 字串解析為 JSON 物件。我們可以使用 JSON.parse()
函式來做到這一點。這是一個 javascript 函式,我們可以使用它來將 JSON 字串轉換為物件。
此函式接受兩個引數:要解析的 JSON 字串和稱為 reviver 的可選引數。根據 mozilla.org,如果 reviver 引數是一個函式,它指定如何轉換最初解析的值。
const fs = require("fs");
const friends = fs.readFileSync("./friends.json", {encoding: 'utf-8'});
const parsed_result = JSON.parse(friends);
console.log(parsed_result);
輸出:
[
{ id: 0, name: 'Maribel Andrews' },
{ id: 1, name: 'Gilliam Mcdonald' },
{ id: 2, name: 'Lisa Wright' }
]
在本節中,我們將看到非同步解析 JSON 字串的方法。在前面的示例中,我們已經瞭解瞭如何同步解析 JSON 字串資料。但是,在 Node js 中,編寫同步函式被認為是不好的,尤其是在資料很大的情況下。
我們可以使用 fs.readFile()
方法將 JSON 字串資料解析為 JSON 物件並回撥函式。
它將保證程式的其餘部分將在後臺獲取資料的同時執行,從而減少載入資料的不必要等待時間。
const fs = require('fs');
fs.readFile('./friends.json',{encoding: 'utf-8'}, (err, data) => {
if (err) console.error(err);
const parsed_data = JSON.parse(data);
console.log(parsed_data);
});
console.log("Rest of the program that needs to be executed!");
樣本輸出:
Rest of the program that needs to be executed!
[
{ id: 0, name: 'Maribel Andrews' },
{ id: 1, name: 'Gilliam Mcdonald' },
{ id: 2, name: 'Lisa Wright' }
]
雖然在處理大量資料時,這種方法似乎比前面討論的同步方法更好,但事實並非如此。當我們有來自外部源的資料時,我們經常需要在進行新的更改時再次重新載入資料;這與使用同步方法時的情況相同。在處理大量外部資料時,推薦使用本機 Node js 流 APIS。
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn