jQuery 中的 $.Ajax 資料型別
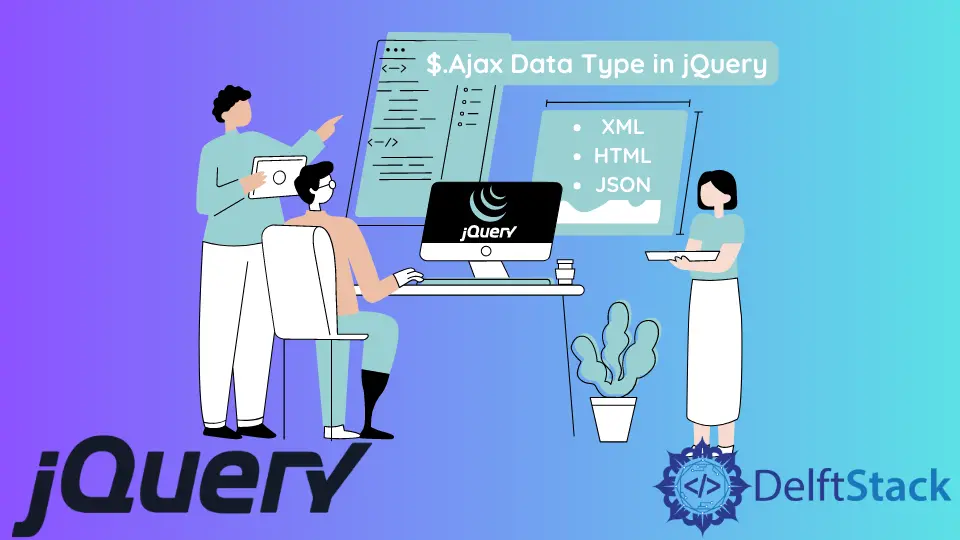
jQuery ajax 請求中的資料型別是我們期望從伺服器獲得的資料型別。本教程描述了 jQuery ajax 中資料型別的使用。
jQuery 中的 Ajax 資料型別
ajax 請求中的資料型別是指我們期望從伺服器獲得的資料型別。如果沒有指定資料,jQuery 將根據響應的 MIME
型別進行設定。
通常,資料是純文字、HTML 或 JSON。下面給出了一個帶有資料型別的簡單 ajax 請求。
$.ajax({
type : "POST",
url : user,
datatype : "application/json",
contentType: "text/plain",
success : function(employee_data) {
//some code here
},
error : function(error) {
//some error error here
},
在上述請求中,我們期望伺服器提供的資料型別是 JSON。資料型別的型別始終是字串。
ajax 請求的可用資料型別有:
-
XML
將返回一個可由 jQuery 處理的文件的 XML 檔案。 -
HTML
將返回 HTML 作為純文字,其中指令碼標籤在插入 DOM 時被評估。 -
script
會將響應評估為 JavaScript 並將其作為純文字返回。我們需要使用帶有 URL 的查詢字串引數=[TIMESTAMP]
禁用快取,直到快取選項為真,並且此方法會將 POST 請求轉換為遠端域請求的 GET。 -
JSON
會將響應評估為 JSON 並返回一個 JavaScript 物件。跨域JSON
請求將被轉換為jsonp
,除非它在請求選項中包含jsonp : false
。JSON 資料將被嚴格解析;任何有故障的 JSON 都將被拒絕,並丟擲錯誤。在較新版本的 jQuery 中,空響應也會被拒絕。
-
jsonp
將使用 JSONP 載入到 JSON 塊中。我們可以在 URL 的末尾新增一個額外的callback
來指定它。我們還可以通過將
_=[TIMESTAMP]
附加到 URL 來禁用快取,直到快取選項為true
。 -
text
將返回純文字字串。
以下示例是使用上述資料型別的一些 ajax 請求。
將 XML 用於 Ajax 請求
用於在自定義 XML 模式中傳輸資料的 ajax 請求。
$.ajax({
url: 'http://demoxmlurl',
type: 'GET',
dataType: 'xml',
success: parseXml
});
});
將 HTML 用於 Ajax 請求
將 HTML 塊傳輸到頁面某處的 ajax 請求。
$.ajax({
type: 'POST',
url: 'post.php',
dataType: 'json',
data: {id: $('#id').val()},
});
為 Ajax 請求使用指令碼
向頁面新增新指令碼的 ajax 請求。
$.ajax({url: 'http://unknown.jquery.com/foo', dataType: 'script', cache: true})
.then(
function() {
console.log('Success');
},
function() {
console.log('Failed');
});
將 JSON 用於 Ajax 請求
用於傳輸 JSON 資料的 ajax 請求將包括任何型別的資料。
$.ajax({
url: 'delftstack.php',
type: 'POST',
data: {ID: ID, First_Name: First_Name, Last_Name: Last_Name, Salary: Salary},
dataType: 'JSON',
success: function(employee_data) {
console.log('Success');
$('#result').text(employee_data);
}
});
使用 JSONP 處理 Ajax 請求
從另一個域傳輸 JSON 資料的 ajax 請求。
$.ajax({
type: 'GET',
url: url,
async: false,
jsonpCallback: 'jsonCallback',
contentType: 'application/json',
dataType: 'jsonp',
success: function(json) {
console.dir(json.sites);
},
error: function(er) {
console.log(er.message);
}
});
將文字用於 Ajax 請求
傳輸純文字字串的 ajax 請求。
$.ajax({
type: 'POST',
url: 'delftstack.php',
data: '{}',
async: true,
dataType: 'text',
success: function(data) {
console.log(data);
}
});
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook