在 Java 中獲取 SQL ResultSet 的大小
Sheeraz Gul
2023年10月12日
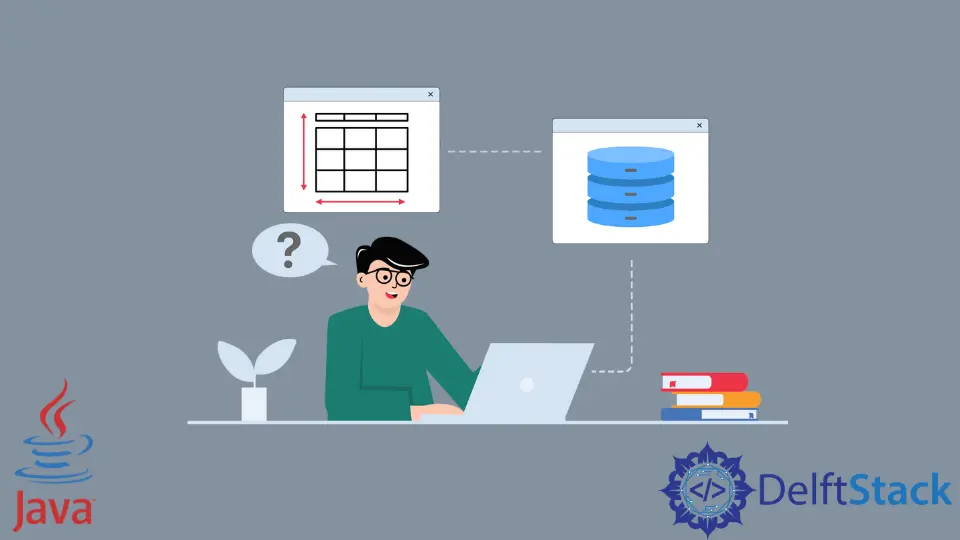
在 Java 中查詢 SQL ResultSet
的大小可能很棘手,因為 SQL 不提供諸如 length()
或 size()
之類的任何方法;它只提供了滾動資料庫的方法,這也是基於資料庫型別的。
本教程演示了一種使用任何資料庫查詢 SQL ResultSet
大小的通用方法。
在 Java 中查詢 SQL ResultSet
的大小
首先,確定要查詢 ResultSet
大小的資料庫表並確定你的查詢。我們建立了一個示例資料庫,我們將通過選擇查詢中的所有專案來獲得 ResultSet
的大小。
我們建立的資料庫如下。
現在讓我們嘗試使用 Java 獲取 ResultSet
的大小。我們使用 SqlLite
資料庫,但該程式將適用於其他資料庫,如 Oracle
和 MySQL
並正確建立連線。
請參閱 Java 示例:
package Delfstack;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
class Get_Result_Set {
public static void main(String[] args) {
Connection db_con = null;
PreparedStatement db_Statement = null;
ResultSet result_set = null;
try {
String db_path = "jdbc:sqlite:path-to-db/sample.db";
db_con = DriverManager.getConnection(db_path);
System.out.println("The Data Based Connection is established.");
db_Statement = db_con.prepareStatement(
"select * from Products", ResultSet.TYPE_FORWARD_ONLY, ResultSet.CONCUR_READ_ONLY);
result_set = db_Statement.executeQuery();
int Row_Count = 0;
System.out.println("Display all the records in ResultSet object");
System.out.println("Product_Id\tProduct_Name");
while (result_set.next()) {
System.out.println(
result_set.getString("Product_Id") + "\t\t" + result_set.getString("Product_Name"));
// Row count will get the length of result set
Row_Count++;
}
System.out.println("Total number of rows in ResultSet object = " + Row_Count);
} catch (SQLException e) {
throw new Error("Problem", e);
} finally {
try {
if (db_con != null) {
db_con.close();
}
} catch (SQLException ex) {
System.out.println(ex.getMessage());
}
}
}
}
上面的程式碼計算了行的迭代次數,這將得到 ResultSet
的大小。檢視程式的輸出:
The Data Based Connection is established.
Display all the records in ResultSet object
Product_Id Product_Name
1 Delfstack1
2 Delftstack2
3 Delftstack3
4 Delftstack4
5 Delftstack5
6 Delftstack6
7 Delftstack7
8 Delftstack8
9 Delftstack9
10 Delftstack10
The Size of the ResultSet object according to rows = 10
查詢 select * from Products
的 ResultSet
的大小為 10。
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook