在 C++ 中使用 std::stod 系列函式
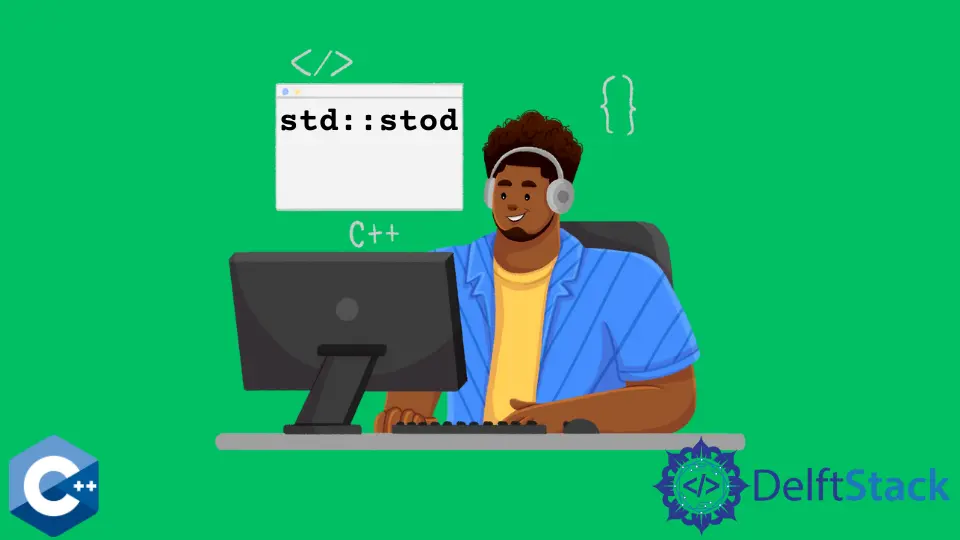
本文解釋並演示瞭如何在 C++ 中使用 std::stod
系列函式的幾種方法。
在 C++ 中使用 std::stod
將 string
轉換為浮點值
std::stod
函式連同 std::stof
和 std::stold
是由 STL 提供的,用於將 string
轉換為浮點數。請注意,我們說的是轉換,但它更像是將字串內容解釋為浮點值。也就是說,這些函式使用預定義的解析規則掃描給定的字串
引數,以識別有效的浮點數並將它們儲存在相應的型別對象中。
函式通過字尾來區分,字尾表示它們返回的型別。std::stod
物件返回 double
值,std::stof
返回一個浮點數,而 std::stold
返回 long double
。這些函式自 C++11 起就成為 STL 的一部分,幷包含在 <string>
標頭檔案中。
在下面的程式碼片段中,我們將探索不同的轉換場景並深入研究這些函式的解析規則。第一個示例是 string
物件僅包含數字和十進位制分隔符 (.
) 的最簡單情況。std::stod
函式將給定的字元序列解釋為有效的浮點數並將它們儲存在 double
型別中。請注意,十進位制分隔符不能是逗號(,
)字元,因為它不會被視為數字的一部分。
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
string str1 = "123.0";
string str2 = "0.123";
auto m1 = std::stod(str1);
auto m2 = std::stod(str2);
cout << "std::stod(\"" << str1 << "\") is " << m1 << endl;
cout << "std::stod(\"" << str2 << "\") is " << m2 << endl;
return EXIT_SUCCESS;
}
輸出:
std::stod("123.0") is 123
std::stod("0.123") is 0.123
或者,如果我們有 string
物件,其中數字與其他字元混合,則可以挑出兩種常見情況。第一種情況:string
物件以數字開頭,後跟其他字元; std::stod
函式在遇到第一個非數字字元(不包括十進位制分隔符)之前提取起始數字。
第二種情況:string
引數以非數字字元開頭,在這種情況下,函式會丟擲 std::invalid_argument
異常並且無法進行轉換。
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
string str3 = "123.4 with chars";
string str4 = "chars 1.2";
auto m3 = std::stod(str3);
// auto m4 = std::stod(str4);
cout << "std::stod(\"" << str3 << "\") is " << m3 << endl;
return EXIT_SUCCESS;
}
輸出:
std::stod("123.0") is 123
std::stod("0.123") is 0.123
通常,std::stod
及其函式系列會丟棄起始空白字元。因此,如果我們傳遞一個帶有多個前導空格字元後跟數字的字串,則轉換將成功執行,如下例所示。
此外,這些函式可以採用 size_t*
型別的可選引數,如果呼叫成功,它將儲存處理的字元數。請注意,如果將字串轉換為有效的浮點數,則前導空格字元也會計數。
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
string str3 = "123.4 with chars";
string str5 = " 123.4";
size_t ptr1 = -1;
size_t ptr2 = -1;
auto m4 = std::stod(str3, &ptr1);
auto m5 = std::stod(str5, &ptr2);
cout << m4 << " - characters processed: " << ptr1 << endl;
cout << "std::stod(\"" << str5 << "\") is " << m5 << " - " << ptr2
<< " characters processed" << endl;
cout << "length: " << str5.size() << endl;
return EXIT_SUCCESS;
}
輸出:
123.4 - characters processed: 5
std::stod(" 123.4") is 123.4 - 16 characters processed
length: 16