在 C 語言中列印數字的二進位制
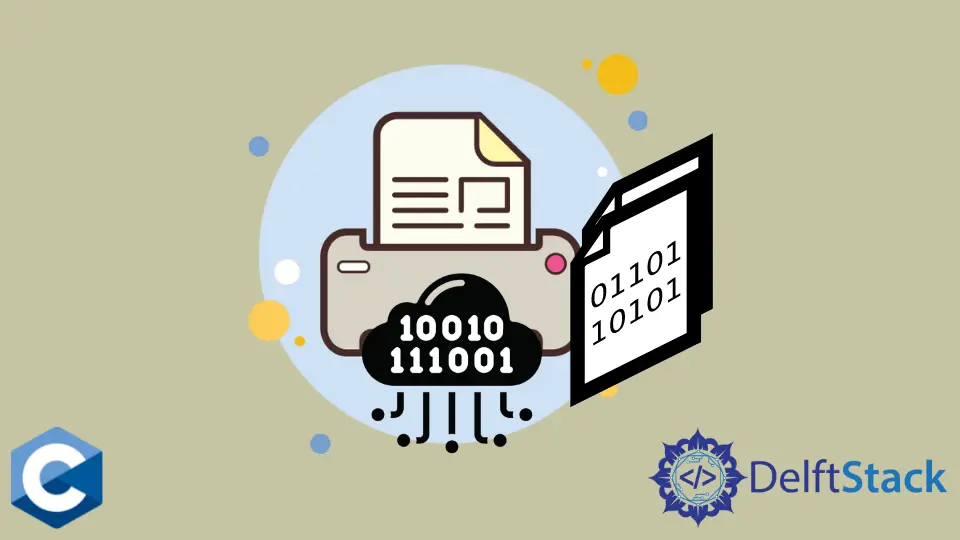
這個簡單的指南是關於使用 C 語言實現十進位制到二進位制數字系統轉換器的。在直接進入實現之前,我們將首先回顧一下二進位制數系統,然後討論多個 C 實現,以將十進位制表示轉換為二進位制等值。
二進位制數制
任何在兩個離散或分類狀態下執行的系統都稱為二進位制系統。同樣,二進位制數字系統僅使用兩個符號表示數字:1
(一)和 0
(零)。
因此,它也被稱為 base-2 系統。
目前,大多數基於電晶體的邏輯電路實現都使用離散的二進位制狀態。因此,所有現代數字計算機都使用二進位制系統來表示、儲存和處理資料。
例如,將 6
轉換為二進位制數系統。
$$
(6)_{10} = (110)_2
$$
這裡,6
是來自以 10
為底的十進位制數系統中的數,其對應的二進位制是 110
,在以 2
為底的二進位制數系統中。我們來看看這個轉換的過程。
轉換過程
第 1 步:將 6 除以 2 得到答案。使用在此階段獲得的整數商,你將獲得下一步的紅利。
以這種方式繼續,直到商達到零。
商 | 餘 |
---|---|
6/2 = 3 | 0 |
3/2 = 1 | 1 |
1/2 = 0 | 1 |
第 2 步:二進位制可以通過收集所有的餘數按照相反的時間順序(從下到上)形成。
1
是最高有效位 (MSB),0
是最低有效位 (LSB)。因此,6
的二進位制是 110
。
轉換的 C 實現
C 語言中有多種方法可以將數字轉換為二進位制數系統。它可以是迭代解決方案或遞迴解決方案。
這取決於你選擇的程式設計方式。本文將討論遞迴解決方案,因為它非常簡單。
解決方案 1:
如果 number > 1
:
- 將
number
放在堆疊上 - 遞迴呼叫
number/2
函式 - 從堆疊中取出一個
number
,將其除以二,然後輸出餘數。
#include <stdio.h>
void convertToBinary(unsigned a) {
/* step 1 */
if (a > 1) convertToBinary(a / 2);
/* step 2 */
printf("%d", a % 2);
}
int main() {
// Write C code here
printf("Binary of the number is: ");
convertToBinary(6);
printf("\n");
return 0;
}
此程式碼段釋出以下輸出。
Binary of the number is: 110
解決方案 2:
- 檢查是否
number > 0
- 將右移運算子應用 1 位,然後遞迴呼叫該函式。
- 輸出
number
的位
#include <stdio.h>
void convertToBinary(unsigned n) {
if (n > 1) convertToBinary(n >> 1);
printf("%d", n & 1);
}
int main() {
// Write C code here
printf("Binary of the number is: ");
convertToBinary(8);
printf("\n");
return 0;
}
給定程式碼片段的輸出是:
Binary of the number is: 1000
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn