Pandas DataFrame DataFrame.replace()函式
Suraj Joshi
2023年1月30日
Pandas
Pandas DataFrame
-
pandas.DataFrame.replace()
語法 -
示例程式碼:使用
pandas.DataFrame.replace()
替換 DataFrame 中的值 -
示例程式碼:在 DataFrame 中使用
pandas.DataFrame.replace()
替換多個值
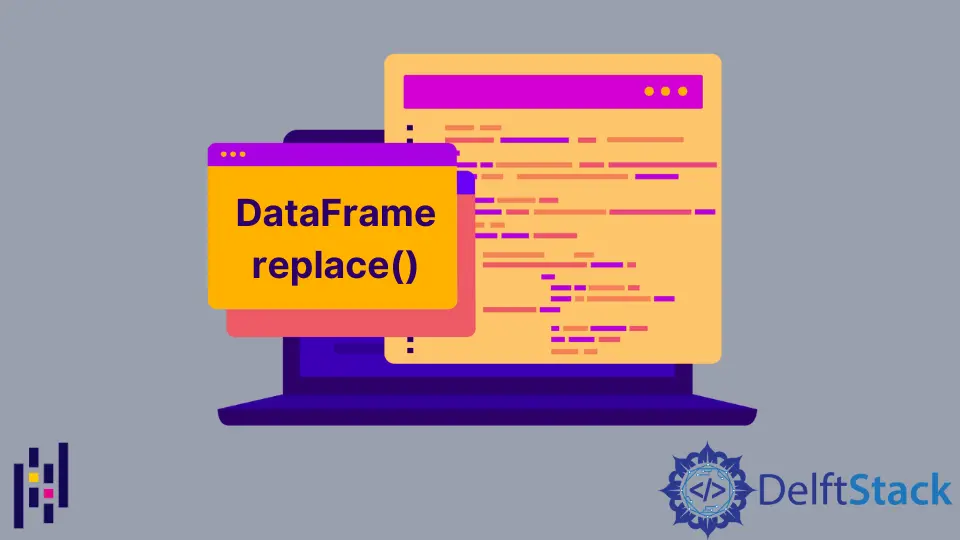
pandas.DataFrame.replace()
用其他值替換 DataFrame 中的值,這些值可以是字串、正規表示式、列表、字典、Series
或數字。
pandas.DataFrame.replace()
語法
DataFrame.replace(,
to_replace=None,
value=None,
inplace=False,
limit=None,
regex=False,
method='pad')
引數
to_replace |
字串,正規表示式,列表,字典,系列,數字或 None . 需要替換的 DataFrame 中的值。 |
value |
標量,字典,列表,字串,正規表示式或 None . 替換任何與 to_replace 匹配的值。 |
inplace |
布林值。如果為 True 修改呼叫者的 DataFrame |
limit |
整數。向前或向後填充的最大間隙大小 |
regex |
布林型。如果 to_replace 和/或 value 是一個正規表示式,則將 regex 設為 True 。 |
method |
用於替換的方法 |
返回值
它返回一個 DataFrame
,用給定的 value
替換所有指定的欄位。
示例程式碼:使用 pandas.DataFrame.replace()
替換 DataFrame 中的值
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3,],
'Y': [4, 1, 8]})
print("Before Replacement")
print(df)
replaced_df=df.replace(1, 5)
print("After Replacement")
print(replaced_df)
輸出:
Before Replacement
X Y
0 1 4
1 2 1
2 3 8
After Replacement
X Y
0 5 4
1 2 5
2 3 8
這裡,1
代表 to_replace
引數,5
代表 replace()
方法中的 value
引數。因此,在 df
中,所有值為 1
的元素都被 5
取代。
示例程式碼:在 DataFrame 中使用 pandas.DataFrame.replace()
替換多個值
使用列表替換
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3,],
'Y': [4, 1, 8]})
print("Before Replacement")
print(df)
replaced_df=df.replace([1,2,3],[1,4,9])
print("After Replacement")
print(replaced_df)
輸出:
Before Replacement
X Y
0 1 4
1 2 1
2 3 8
After Replacement
X Y
0 1 4
1 4 1
2 9 8
這裡,[1,2,3]
代表 to_replace
引數,[1,4,9]
代表 replace()
方法中的 value
引數。因此,在 df
中,列 [1,2,3]
被 [1,4,9]
替換。
使用字典替換
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3,],
'Y': [3, 1, 8]})
print("Before Replacement")
print(df)
replaced_df=df.replace({1:10,3:30})
print("After Replacement")
print(replaced_df)
輸出:
Before Replacement
X Y
0 1 3
1 2 1
2 3 8
After Replacement
X Y
0 10 30
1 2 10
2 30 8
它將所有值為 1
的元素替換為 10
,將所有值為 3
的元素替換為 30
。
使用 Regex
替換
import pandas as pd
df = pd.DataFrame({'X': ["zeppy", "amid", "amily"],
'Y': ["xar", "abc", "among"]})
print("Before Replacement")
print(df)
df.replace(to_replace=r'^ami.$', value='song', regex=True,inplace=True)
print("After Replacement")
print(df)
輸出:
Before Replacement
X Y
0 zeppy xar
1 amid abc
2 amily among
After Replacement
X Y
0 zeppy xar
1 song abc
2 amily among
它將所有前三個字元為 ami
然後後面跟任何一個字元的元素替換為 song
。這裡只有 amid
滿足給定的正規表示式,因此只有 amid
被 song
替換。雖然 amily
的前三個字元是 ami
,但 ami
後面還有兩個字元。所以,amily
不滿足給定的 regex,因此它保持一樣,沒被替換。如果你使用的是正規表示式,請確保 regex
設定為 True
,並且 inplace=True
在呼叫 replace()
方法後修改原來的 DataFrame
。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn