Remover palavras de parada em Python
-
Use o pacote
NLTK
para remover palavras de parada em Python -
Use o pacote
stop-words
para remover palavras de parada em Python -
Use o método
remove_stpwrds
na bibliotecatextcleaner
para remover palavras de parada em Python
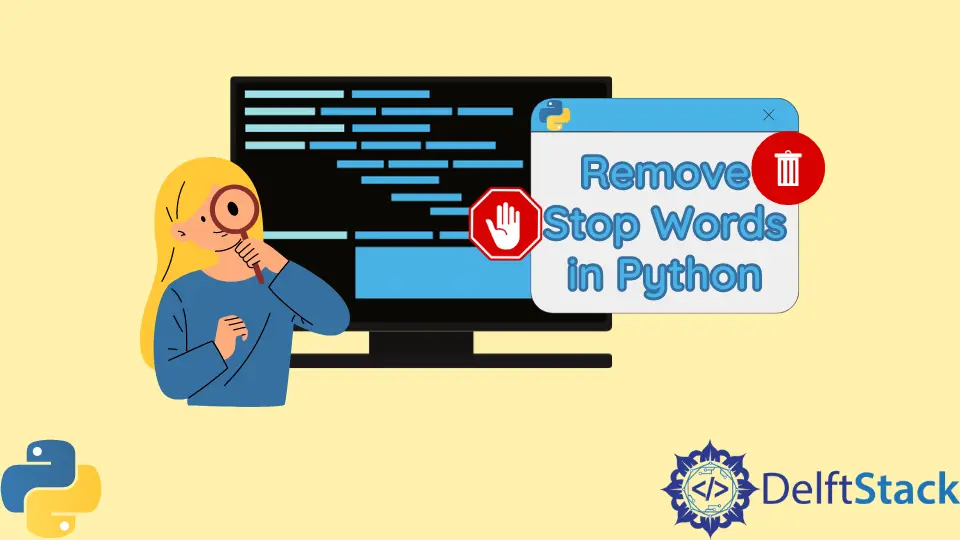
Palavras irrelevantes são as palavras comumente usadas que geralmente são ignoradas pelo mecanismo de pesquisa, como the
, a
, an
e muito mais. Essas palavras são removidas para economizar espaço no banco de dados e o tempo de processamento. A frase, There is a snake in my boot
sem palavras de interrupção será apenas snake boot
.
Neste tutorial, discutiremos como remover palavras de parada em Python.
Use o pacote NLTK
para remover palavras de parada em Python
O pacote nlkt
(Processamento de linguagem natural) pode ser usado para remover palavras de parada do texto em Python. Este pacote contém palavras de parada de muitos idiomas diferentes.
Podemos iterar por meio de uma lista e verificar se uma palavra é uma palavra de parada ou não usando a lista desta biblioteca.
Por exemplo,
import nltk
from nltk.corpus import stopwords
dataset = ["This", "is", "just", "a", "snake"]
A = [word for word in dataset if word not in stopwords.words("english")]
print(A)
Produção:
['This', 'snake']
O código a seguir mostrará uma lista de palavras de parada em Python:
import nltk
from nltk.corpus import stopwords
print(stopwords.words("english"))
Produção:
{'ourselves', 'hers', 'between', 'yourself', 'but', 'again', 'there', 'about', 'once', 'during', 'out', 'very', 'having', 'with', 'they', 'own', 'an', 'be', 'some', 'for', 'do', 'its', 'yours', 'such', 'into', 'of', 'most', 'itself', 'other', 'off', 'is', 's', 'am', 'or', 'who', 'as', 'from', 'him', 'each', 'the', 'themselves', 'until', 'below', 'are', 'we', 'these', 'your', 'his', 'through', 'don', 'nor', 'me', 'were', 'her', 'more', 'himself', 'this', 'down', 'should', 'our', 'their', 'while', 'above', 'both', 'up', 'to', 'ours', 'had', 'she', 'all', 'no', 'when', 'at', 'any', 'before', 'them', 'same', 'and', 'been', 'have', 'in', 'will', 'on', 'does', 'yourselves', 'then', 'that', 'because', 'what', 'over', 'why', 'so', 'can', 'did', 'not', 'now', 'under', 'he', 'you', 'herself', 'has', 'just', 'where', 'too', 'only', 'myself', 'which', 'those', 'i', 'after', 'few', 'whom', 't', 'being', 'if', 'theirs', 'my', 'against', 'a', 'by', 'doing', 'it', 'how', 'further', 'was', 'here', 'than'}
Use o pacote stop-words
para remover palavras de parada em Python
O pacote stop-words
é usado para remover stop words do texto em Python. Este pacote contém palavras de parada de muitos idiomas como inglês, dinamarquês, francês, espanhol e muito mais.
Por exemplo,
from stop_words import get_stop_words
dataset = ["This", "is", "just", "a", "snake"]
A = [word for word in dataset if word not in get_stop_words("english")]
print(A)
Produção:
["This", "just", "snake"]
O código acima filtrará o conjunto de dados removendo todas as palavras de parada usadas no idioma inglês.
Use o método remove_stpwrds
na biblioteca textcleaner
para remover palavras de parada em Python
O método remove_stpwrds()
na biblioteca textcleaner
é usado para remover palavras de parada do texto em Python.
Por exemplo,
import textcleaner as tc
dataset = ["This", "is", "just", "a", "snake"]
data = tc.document(dataset)
print(data.remove_stpwrds())
Produção:
This
snake