Eliminar palabras vacías en Python
-
Utilice el paquete
NLTK
para eliminar palabras vacías en Python -
Utilice el paquete
stop-words
para eliminar las palabras de parada en Python -
Utilice el método
remove_stpwrds
en la bibliotecatextcleaner
para eliminar palabras vacías en Python
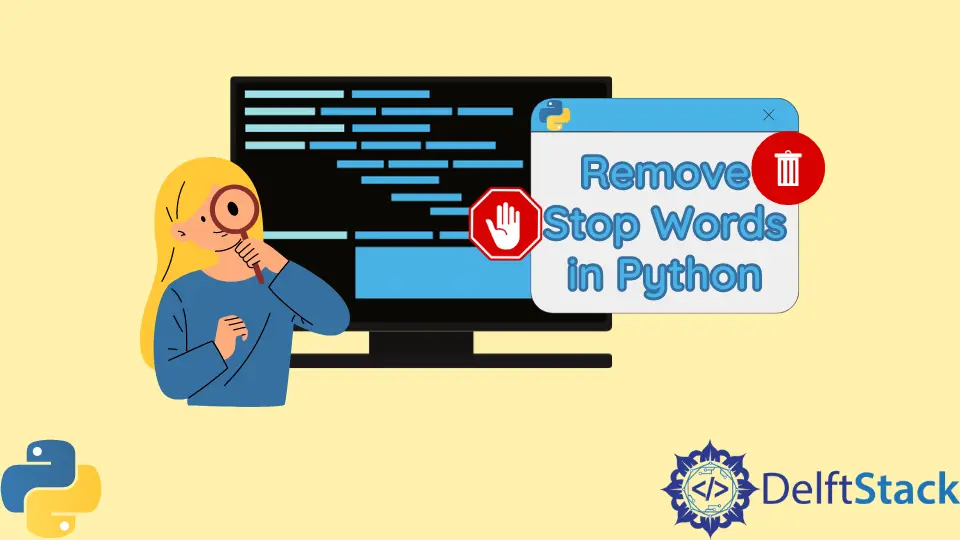
Las palabras vacías son las palabras de uso común que generalmente son ignoradas por el motor de búsqueda, como the
, a
, an
, y más. Estas palabras se eliminan para ahorrar espacio en la base de datos y el tiempo de procesamiento. La frase There is a snake in my boot
sin palabras vacías será simplemente snake boot
.
En este tutorial, discutiremos cómo eliminar palabras vacías en Python.
Utilice el paquete NLTK
para eliminar palabras vacías en Python
El paquete nlkt
(Natural Language Processing) se puede utilizar para eliminar palabras vacías del texto en Python. Este paquete contiene palabras vacías de muchos idiomas diferentes.
Podemos iterar a través de una lista y verificar si una palabra es una palabra de parada o no usando la lista de esta biblioteca.
Por ejemplo,
import nltk
from nltk.corpus import stopwords
dataset = ["This", "is", "just", "a", "snake"]
A = [word for word in dataset if word not in stopwords.words("english")]
print(A)
Producción :
['This', 'snake']
El siguiente código mostrará una lista de palabras vacías en Python:
import nltk
from nltk.corpus import stopwords
print(stopwords.words("english"))
Producción :
{'ourselves', 'hers', 'between', 'yourself', 'but', 'again', 'there', 'about', 'once', 'during', 'out', 'very', 'having', 'with', 'they', 'own', 'an', 'be', 'some', 'for', 'do', 'its', 'yours', 'such', 'into', 'of', 'most', 'itself', 'other', 'off', 'is', 's', 'am', 'or', 'who', 'as', 'from', 'him', 'each', 'the', 'themselves', 'until', 'below', 'are', 'we', 'these', 'your', 'his', 'through', 'don', 'nor', 'me', 'were', 'her', 'more', 'himself', 'this', 'down', 'should', 'our', 'their', 'while', 'above', 'both', 'up', 'to', 'ours', 'had', 'she', 'all', 'no', 'when', 'at', 'any', 'before', 'them', 'same', 'and', 'been', 'have', 'in', 'will', 'on', 'does', 'yourselves', 'then', 'that', 'because', 'what', 'over', 'why', 'so', 'can', 'did', 'not', 'now', 'under', 'he', 'you', 'herself', 'has', 'just', 'where', 'too', 'only', 'myself', 'which', 'those', 'i', 'after', 'few', 'whom', 't', 'being', 'if', 'theirs', 'my', 'against', 'a', 'by', 'doing', 'it', 'how', 'further', 'was', 'here', 'than'}
Utilice el paquete stop-words
para eliminar las palabras de parada en Python
El paquete stop-words
se utiliza para eliminar palabras vacías del texto en Python. Este paquete contiene palabras vacías de muchos idiomas como inglés, danés, francés, español y más.
Por ejemplo,
from stop_words import get_stop_words
dataset = ["This", "is", "just", "a", "snake"]
A = [word for word in dataset if word not in get_stop_words("english")]
print(A)
Producción :
['This', 'just', 'snake']
El código anterior filtrará el conjunto de datos eliminando todas las palabras vacías utilizadas en el idioma inglés.
Utilice el método remove_stpwrds
en la biblioteca textcleaner
para eliminar palabras vacías en Python
El método remove_stpwrds()
en la biblioteca textcleaner
se utiliza para eliminar palabras vacías del texto en Python.
Por ejemplo,
import textcleaner as tc
dataset = ["This", "is", "just", "a", "snake"]
data = tc.document(dataset)
print(data.remove_stpwrds())
Producción :
This
snake