React 요소의 너비 가져오기
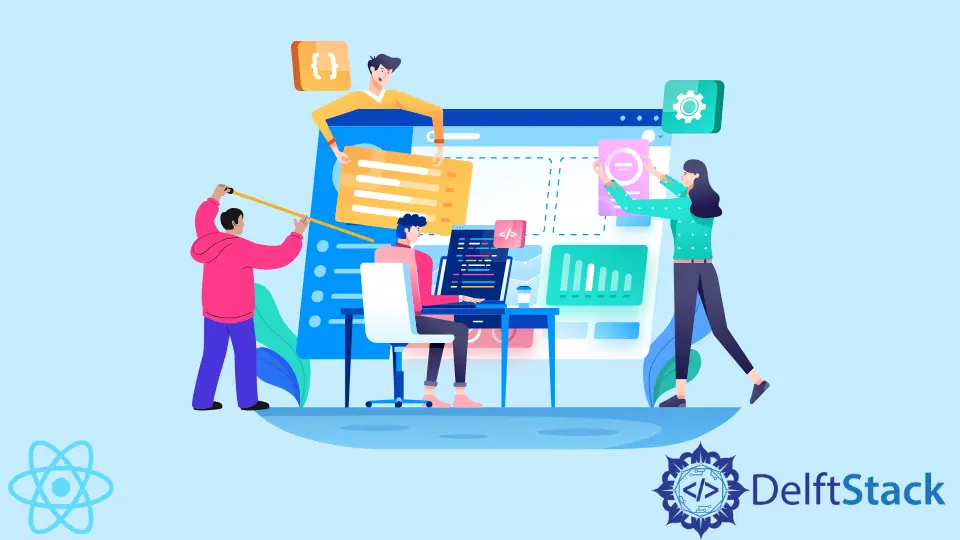
이 기사에서는 React에서 요소의 너비를 얻는 방법을 배웁니다. 너비 또는 기타 치수에 따라 일부 작업을 수행하려면 요소의 너비를 가져와야 하는 경우가 많습니다. 따라서 여기에서는 useRef()
React 후크를 사용하여 특정 요소의 크기를 가져옵니다.
useRef()
반응 후크를 사용하여 요소의 너비 가져오기
React에서 useRef()
의 작업을 처음부터 이해해 봅시다.
먼저 바닐라 JavaScript에서 요소의 너비를 가져와야 하는지 고려하십시오. 너 뭐하니? 대답은 먼저 DOM 요소에 액세스하고 해당 DOM 요소의 width
속성을 가져오는 것입니다.
여기에서 useRef()
를 사용하여 React DOM 요소에 대한 참조를 생성합니다. 사용자는 useRef()
후크를 사용하여 아래와 같은 DOM 요소의 참조를 가져올 수 있습니다.
const ref = useRef(Initial DOM element);
return (
<div ref={ref} >
</div>
);
useRef()
후크에는 current
속성이 포함되어 있으며 이를 사용하여 참조된 요소에 액세스할 수 있습니다. 참조 요소를 얻은 후 offsetWidth
를 사용하여 특정 참조 요소의 너비를 얻을 수 있습니다.
let width = ref.current.offsetWidth;
아래 예에서는 HTML <div>
요소를 생성하고 ref
변수를 사용하여 참조했습니다. 처음에는 div의 height
및 width
를 200px로 설정했습니다.
또한 Change Div Size
버튼을 추가했으며 사용자가 이를 클릭하면 changeDivSize()
기능이 실행됩니다.
changeDivSize()
함수는 100에서 300 사이의 임의 값을 생성하고 이에 따라 div
요소 크기를 변경합니다. 그런 다음 ref.current.offsetWidth
를 사용하여 div
요소의 새 너비를 가져왔습니다.
여기에서는 div
요소의 새 너비를 가져오기 위해 약간의 지연을 추가하기 위해 setTimeout()
함수를 사용했습니다.
예제 코드:
import { useState, useRef } from "react";
function App() {
// creating the variables for the div width and height
let [divWidth, setDivWidth] = useState("200px");
let [divheight, setDivheight] = useState("200px");
// variable to store the element's width from `ref`.
let [widthByRef, setWidthByRef] = useState(200);
// It is used to get the reference of any component or element
const ref = useRef(null);
// whenever user clicks on Change Div size button, ChangeDivSize() function will be called.
function changeDivSize() {
// get a random number between 100 and 300;
let random = 100 + Math.random() * (300 - 100);
// convert the random number to a string with pixels
random = random + "px";
// change the height and width of the div element.
setDivWidth(random);
setDivheight(random);
// get the width of the div element using ref.
// reason to add the setTimeout() function is that we want to get the width of the Div once it is updated in the DOM;
// Otherwise, it returns the old width.
setTimeout(() => {
setWidthByRef(ref.current ? ref.current.offsetWidth : 0);
}, 100);
}
return (
<div>
{/* showing width which we got using ref */}
<h3>Width of Element is : {widthByRef}</h3>
{/* resizable div element */}
<div
ref={ref}
style={{ backgroundColor: "red", height: divheight, width: divWidth }}
>
Click on the below buttons to resize the div.
</div>
{/* button to resize the div element */}
<button style={{ marginTop: "20px" }} onClick={changeDivSize}>
Change Div Size
</button>
</div>
);
}
export default App;
출력:
위의 출력에서 사용자는 버튼을 클릭하면 div
의 크기가 변경되고 웹 페이지에 새 너비가 표시되는 것을 볼 수 있습니다.