Reaccionar Obtener el ancho de un elemento
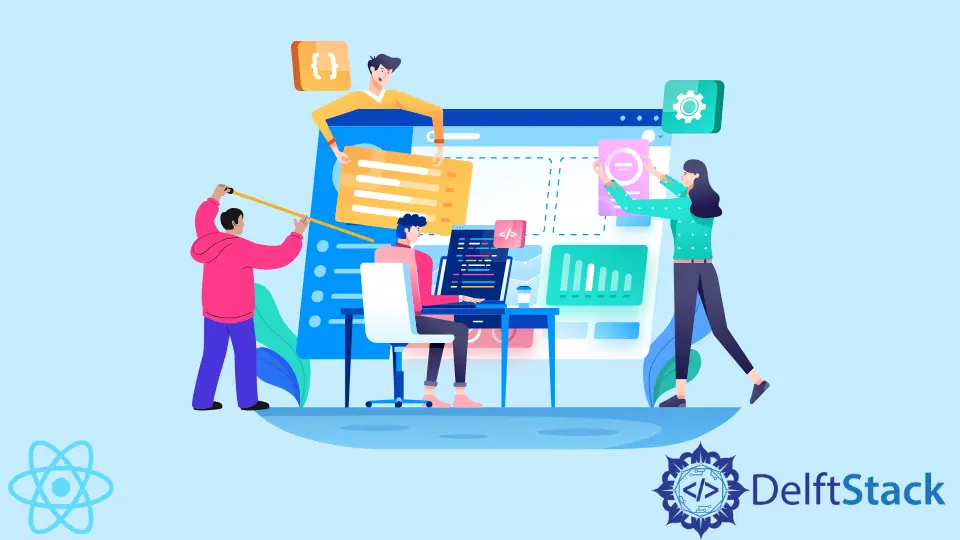
En este artículo, aprenderemos a obtener el ancho de un elemento en React. A menudo requiere obtener el ancho del elemento para realizar alguna operación de acuerdo con el ancho u otras dimensiones. Entonces, aquí usaremos el gancho de React useRef()
para obtener las dimensiones de un elemento en particular.
Utilice el gancho de reacción useRef()
para obtener el ancho de un elemento
Intentemos entender el funcionamiento de useRef()
desde cero en React.
Primero, considere si necesita obtener el ancho de un elemento en JavaScript estándar; ¿a qué te dedicas? La respuesta es que primero acceda al elemento DOM y obtenga la propiedad de ancho
de ese elemento DOM.
Aquí, usaremos useRef()
para crear una referencia al elemento React DOM. Los usuarios pueden usar el gancho useRef()
para obtener la referencia de cualquier elemento DOM como el que se muestra a continuación.
const ref = useRef(Initial DOM element);
return (
<div ref={ref} >
</div>
);
El gancho useRef()
contiene una propiedad actual
, y podemos acceder al elemento al que se hace referencia usándolo. Después de obtener el elemento de referencia, podemos usar offsetWidth
para obtener el ancho de un elemento al que se hace referencia en particular.
let width = ref.current.offsetWidth;
En el siguiente ejemplo, hemos creado el elemento HTML <div>
y lo hemos referenciado usando la variable ref
. Inicialmente, establecemos la “altura” y el “ancho” del div en 200px.
Además, hemos agregado el botón Cambiar tamaño de div
, y cuando el usuario haga clic en él, ejecutará una función cambiar tamaño de div ()
.
La función changeDivSize()
genera un valor aleatorio entre 100 y 300 y cambia el tamaño del elemento div
de acuerdo con eso. Después de eso, usamos ref.current.offsetWidth
para obtener el nuevo ancho del elemento div
.
Aquí, hemos usado la función setTimeout()
para agregar algo de retraso para obtener el nuevo ancho del elemento div
.
Código de ejemplo:
import { useState, useRef } from "react";
function App() {
// creating the variables for the div width and height
let [divWidth, setDivWidth] = useState("200px");
let [divheight, setDivheight] = useState("200px");
// variable to store the element's width from `ref`.
let [widthByRef, setWidthByRef] = useState(200);
// It is used to get the reference of any component or element
const ref = useRef(null);
// whenever user clicks on Change Div size button, ChangeDivSize() function will be called.
function changeDivSize() {
// get a random number between 100 and 300;
let random = 100 + Math.random() * (300 - 100);
// convert the random number to a string with pixels
random = random + "px";
// change the height and width of the div element.
setDivWidth(random);
setDivheight(random);
// get the width of the div element using ref.
// reason to add the setTimeout() function is that we want to get the width of the Div once it is updated in the DOM;
// Otherwise, it returns the old width.
setTimeout(() => {
setWidthByRef(ref.current ? ref.current.offsetWidth : 0);
}, 100);
}
return (
<div>
{/* showing width which we got using ref */}
<h3>Width of Element is : {widthByRef}</h3>
{/* resizable div element */}
<div
ref={ref}
style={{ backgroundColor: "red", height: divheight, width: divWidth }}
>
Click on the below buttons to resize the div.
</div>
{/* button to resize the div element */}
<button style={{ marginTop: "20px" }} onClick={changeDivSize}>
Change Div Size
</button>
</div>
);
}
export default App;
Producción:
En el resultado anterior, los usuarios pueden ver que cuando hacemos clic en el botón, cambia el tamaño del div
y muestra el nuevo ancho en la página web.