기본 프로그래밍 개념을 사용하여 Python에서 곱셈 테이블 인쇄
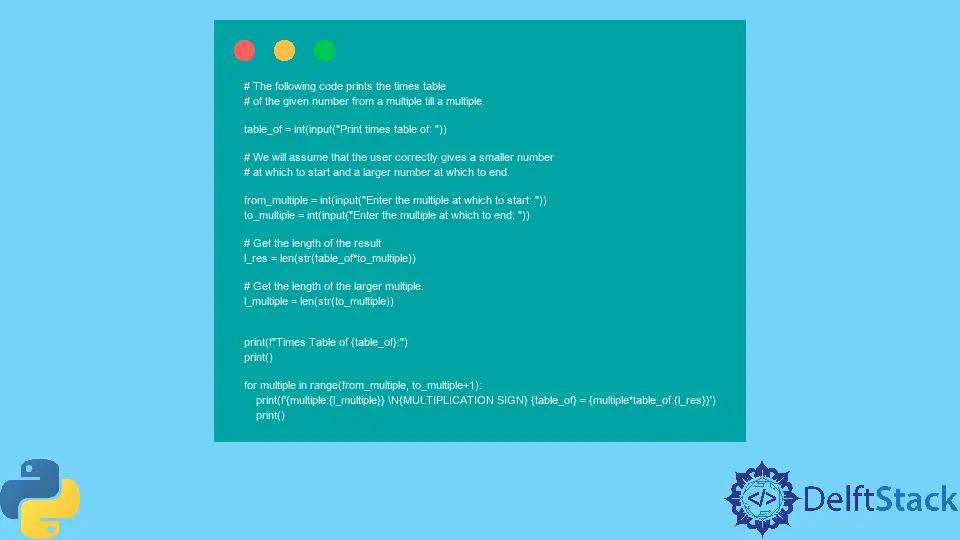
우리는 파이썬에서 시간표를 인쇄하는 법을 배움으로써 몇 가지 기본 프로그래밍 개념을 연습할 수 있습니다. 여기에는 다음이 포함됩니다.
- 변수 사용
- 사용자 입력 받기
- 내장 기능 사용하기
- 유형 캐스팅 변수
- 반복(루프)
- 문자열 형식화
- 유니코드 기호 사용
Python 3.6 이상에서 사용할 수 있는 Python의 f
문자열 형식 지정 기능을 사용합니다.
기본 프로그래밍 개념
다음과 같이 변수를 선언하고 값을 할당할 수 있습니다.
table_of = 5
아래와 같이 input()
함수를 사용하여 사용자 입력을 받습니다.
table_of = input("Print times table of: ")
프로그램은 Print times table of:
문자열을 표시하고 사용자 입력을 기다립니다. 사용자는 무엇이든 입력할 수 있습니다. Python은 입력을 문자열로 해석합니다.
정수로 변환하기 위해 input()
함수 주위에 int()
함수를 사용합니다.
table_of = int(input("Print times table of: "))
print("Times")
는 디스플레이에 Times
라는 단어를 인쇄합니다. 빈 print()
함수는 빈 줄을 인쇄합니다.
range()
함수는 start_int
에서 end_int
까지의 시퀀스를 생성하지만 제외합니다. 기본적으로 1씩 증가합니다.
range(start_int, end_int, step_int)
코드에서 for
루프를 사용할 것입니다. 변수가 지정된 범위에 있는 만큼 루프에서 코드를 반복합니다.
for variable in range(start, end):
code to repeat
Python의 f
문자열 형식 지정 기능을 사용하면 자리 표시자 {}
를 사용하여 문자열에 변수를 포함할 수 있습니다. table_of
변수의 값을 사용하려면 다음을 사용합니다.
print(f"Times table of {table_of}")
정수를 사용하여 자리 표시자의 길이를 지정할 수 있습니다. 코드에서 다른 변수를 사용하여 이를 지정합니다: 결과 table_of * 9
의 길이.
길이를 얻기 위해 str()
을 사용하여 정수를 문자열로 변환합니다.
곱셈 기호는 유니코드 이름을 사용하여 지정됩니다.
\N{MULTIPLICATION SIGN}
파이썬에서 주어진 숫자의 타임즈 테이블 출력하기
이제 위의 모든 개념을 다음 코드에 넣을 것입니다. 두 가지 방법으로 사용자가 지정한 숫자의 곱셈표를 인쇄합니다.
예제 코드:
# The following code prints the times table
# of the given number till 'number x 9'.
# It prints the times table in two different ways.
table_of = int(input("Print times table of: "))
# Get the length of the result
l_res = len(str(table_of * 9))
print(f"Times Table of {table_of}:")
print()
for multiple in range(1, 10):
print(
f"{multiple} \N{MULTIPLICATION SIGN} {table_of} = {table_of*multiple:{l_res}}"
)
print()
print("-------------")
print()
for multiple in range(1, 10):
print(
f"{table_of} \N{MULTIPLICATION SIGN} {multiple} = {table_of*multiple:{l_res}}"
)
print()
샘플 출력:
Print times table of: 1717
Times Table of 1717:
1 × 1717 = 1717
2 × 1717 = 3434
3 × 1717 = 5151
4 × 1717 = 6868
5 × 1717 = 8585
6 × 1717 = 10302
7 × 1717 = 12019
8 × 1717 = 13736
9 × 1717 = 15453
-------------
1717 × 1 = 1717
1717 × 2 = 3434
1717 × 3 = 5151
1717 × 4 = 6868
1717 × 5 = 8585
1717 × 6 = 10302
1717 × 7 = 12019
1717 × 8 = 13736
1717 × 9 = 15453
변형으로 주어진 숫자의 원하는 배수에서 곱셈표를 인쇄할 수 있습니다.
예제 코드:
# The following code prints the times table
# of the given number from a multiple till a multiple.
table_of = int(input("Print times table of: "))
# We will assume that the user correctly gives a smaller number
# at which to start and a larger number at which to end.
from_multiple = int(input("Enter the multiple at which to start: "))
to_multiple = int(input("Enter the multiple at which to end: "))
# Get the length of the result
l_res = len(str(table_of * to_multiple))
# Get the length of the larger multiple.
l_multiple = len(str(to_multiple))
print(f"Times Table of {table_of}:")
print()
for multiple in range(from_multiple, to_multiple + 1):
print(
f"{multiple:{l_multiple}} \N{MULTIPLICATION SIGN} {table_of} = {multiple*table_of:{l_res}}"
)
print()
샘플 출력:
Print times table of: 16
Enter the multiple at which to start: 5
Enter the multiple at which to end: 15
Times Table of 16:
5 × 16 = 80
6 × 16 = 96
7 × 16 = 112
8 × 16 = 128
9 × 16 = 144
10 × 16 = 160
11 × 16 = 176
12 × 16 = 192
13 × 16 = 208
14 × 16 = 224
15 × 16 = 240