Pandas Groupby 가중 평균
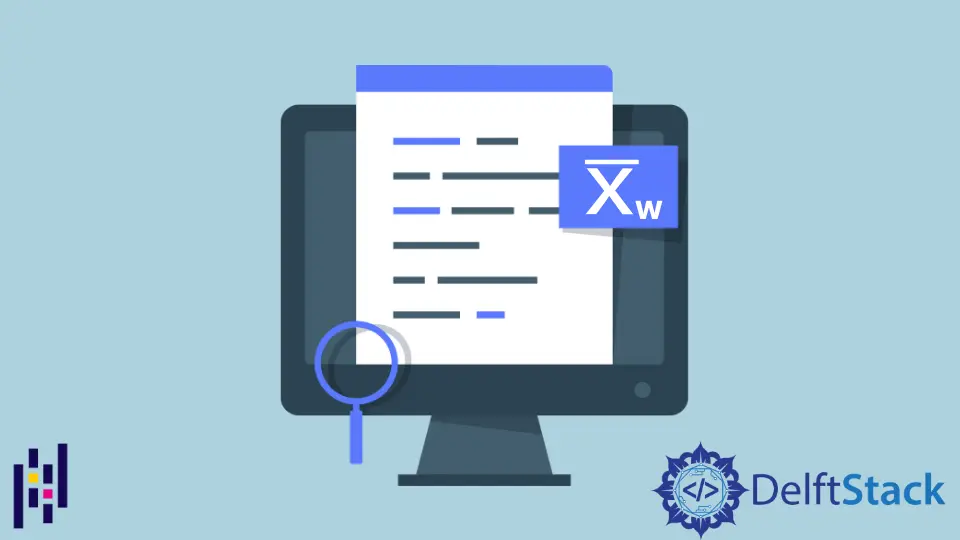
이 기사에서는 Pandas DataFrame의 가중 평균을 계산하는 방법을 배웁니다. 또한 Pandas DataFrame의 가중 평균을 그룹화하는 방법에 대해서도 설명합니다.
Pandas DataFrame의 가중 평균 계산
Pandas를 pd
로 가져온 후 간단한 DataFrame을 만듭니다. 당신이 선생님이고 학생들의 점수를 평가한다고 상상해 봅시다.
전반적으로 Quiz_1
, Quiz_2
및 Quiz_3
의 세 가지 다른 평가가 있습니다.
코드 예:
import pandas as pd
import numpy as np
Student_DF = pd.DataFrame(
{
"Student_Score": [30, 60, 90],
"Std_score_Weight": [1, 2, 3],
"Student_Assessment": ["Quiz_1", "Quiz_2", "Quiz_3"],
}
)
Student_DF
출력:
Student_Score Std_score_Weight Student_Assessment
0 30 1 Quiz_1
1 60 2 Quiz_2
2 90 3 Quiz_3
코드 예:
Student_Average = Student_DF["Student_Score"].mean()
Student_Average
출력:
60.0
이러한 평가는 가중치에 따라 전체 점수에 다르게 영향을 미쳐야 합니다. 따라서 표본 평균 대신 가중 평균을 계산하려고 합니다.
먼저 Student_Score
에 값을 곱한 다음 결과를 가중치의 총합으로 나누어야 하며 이것이 Pandas에서 구현할 수 있는 방법이기도 합니다.
Pandas 라이브러리를 사용하면 벡터화된 계산을 수행할 수 있으므로 Student_Score
에 가중치를 곱하고 합계를 계산할 수 있습니다. 그런 다음 결과를 가중치의 합으로 나누어야 합니다.
코드 예:
Std_weighted_avg = (
Student_DF["Student_Score"] * Student_DF["Std_score_Weight"]
).sum() / Student_DF["Std_score_Weight"].sum()
Std_weighted_avg
이 DataFrame의 경우 가중 평균은 다음과 같습니다.
70.0
Groupby
기능을 사용하여 Pandas에서 가중 평균 그룹화
다음 예에서는 다른 학생에 대해 다른 열을 추가했습니다. 그래서 우리는 여기에 John
과 Jack
학생들이 있습니다.
Student_DF = pd.DataFrame(
{
"Student_Score": [30, 50, 90, 40, 50, 20],
"Std_score_Weight": [1, 2, 3, 1, 2, 3],
"Two_Students": ["John", "John", "John", "Jack", "Jack", "Jack"],
"Students_Assessment": [
"Quiz_1",
"Quiz_2",
"Quiz_3",
"Quiz_1",
"Quiz_2",
"Quiz_3",
],
}
)
Student_DF
출력:
Student_Score Std_score_Weight Two_Students Students_Assessment
0 30 1 John Quiz_1
1 50 2 John Quiz_2
2 90 3 John Quiz_3
3 40 1 Jack Quiz_1
4 50 2 Jack Quiz_2
5 20 3 Jack Quiz_3
학생 Jack
에 대해서만 가중 평균을 계산한다고 가정해 봅시다. 이 경우 여기에서 query()
메서드를 사용하여 수행한 것처럼 데이터를 필터링할 수 있습니다.
필터링된 DataFrame은 다음과 같습니다.
Filtered_by_Jack = Student_DF.query("Two_Students == 'Jack'")
Filtered_by_Jack
출력:
Student_Score Std_score_Weight Two_Students Students_Assessment
3 40 1 Jack Quiz_1
4 50 2 Jack Quiz_2
5 20 3 Jack Quiz_3
이를 통해 이전과 동일한 계산을 적용할 수 있지만 이번에는 필터링된 DataFrame에 적용합니다.
Std_weighted_avg = (
Filtered_by_Jack["Student_Score"] * Filtered_by_Jack["Std_score_Weight"]
).sum() / Filtered_by_Jack["Std_score_Weight"].sum()
Std_weighted_avg
출력:
33.333333333333336
그러나 이 방법은 특히 더 큰 데이터 세트를 처리할 때 지루할 수 있습니다. 예를 들어 100명의 학생이 있고 각 학생의 가중 평균을 계산하려고 합니다.
우리의 경우 데이터 세트에 학생을 한 명 더 추가했습니다.
Student_DF = pd.DataFrame(
{
"Student_Score": [20, 40, 90, 80, 60, 10, 5, 60, 90],
"Std_score_Weight": [1, 2, 3, 1, 2, 3, 1, 2, 3],
"Three_Student": [
"John",
"John",
"John",
"Jack",
"Jack",
"Jack",
"Harry",
"Harry",
"Harry",
],
"Students_Assessment": [
"Quiz_1",
"Quiz_2",
"Quiz_3",
"Quiz_1",
"Quiz_2",
"Quiz_3",
"Quiz_1",
"Quiz_2",
"Quiz_3",
],
}
)
Student_DF
출력:
Student_Score Std_score_Weight Three_Student Students_Assessment
0 20 1 John Quiz_1
1 40 2 John Quiz_2
2 90 3 John Quiz_3
3 80 1 Jack Quiz_1
4 60 2 Jack Quiz_2
5 10 3 Jack Quiz_3
6 5 1 Harry Quiz_1
7 60 2 Harry Quiz_2
8 90 3 Harry Quiz_3
그러나 다음 방법은 데이터 세트에 포함될 수 있는 많은 학생과 관계없이 작동합니다. 이번에는 Groupby_weighted_avg()
라는 작은 도우미 함수를 작성합니다.
이 함수는 가중 평균을 그룹화하려는 values
, weighted_value
및 Group_Cols
라는 열 이름의 세 가지 매개변수를 사용합니다. 계산 방법은 이전과 매우 유사합니다. 유일한 차이점은 groupby()
메서드와 결합한다는 것입니다.
def Groupby_weighted_avg(values, weighted_value, Group_Cols):
return (values * weighted_value).groupby(Group_Cols).sum() / weighted_value.groupby(
Group_Cols
).sum()
Groupby_weighted_avg()
함수를 사용하여 이제 값(예제에서는 Student_Score
및 Std_score_Weight
)을 전달할 수 있습니다. 결과를 Three_Student
로 그룹화하려고 합니다.
Groupby_weighted_avg(
Student_DF["Student_Score"],
Student_DF["Std_score_Weight"],
Student_DF["Three_Student"],
)
위의 줄을 실행하면 각 학생의 가중 평균을 포함하는 새로운 DataFrame이 생성됩니다.
Three_Student
Harry 65.833333
Jack 38.333333
John 61.666667
dtype: float64
완전한 소스 코드:
# In[1]:
import pandas as pd
import numpy as np
Student_DF = pd.DataFrame(
{
"Student_Score": [30, 60, 90],
"Std_score_Weight": [1, 2, 3],
"Student_Assessment": ["Quiz_1", "Quiz_2", "Quiz_3"],
}
)
Student_DF
# In[2]:
Student_Average = Student_DF["Student_Score"].mean()
Student_Average
# In[3]:
Std_weighted_avg = (
Student_DF["Student_Score"] * Student_DF["Std_score_Weight"]
).sum() / Student_DF["Std_score_Weight"].sum()
Std_weighted_avg
# In[4]:
# groupby
Student_DF = pd.DataFrame(
{
"Student_Score": [30, 50, 90, 40, 50, 20],
"Std_score_Weight": [1, 2, 3, 1, 2, 3],
"Two_Students": ["John", "John", "John", "Jack", "Jack", "Jack"],
"Students_Assessment": [
"Quiz_1",
"Quiz_2",
"Quiz_3",
"Quiz_1",
"Quiz_2",
"Quiz_3",
],
}
)
Student_DF
# In[5]:
Filtered_by_Jack = Student_DF.query("Two_Students == 'Jack'")
Filtered_by_Jack
# In[6]:
Std_weighted_avg = (
Filtered_by_Jack["Student_Score"] * Filtered_by_Jack["Std_score_Weight"]
).sum() / Filtered_by_Jack["Std_score_Weight"].sum()
Std_weighted_avg
# In[7]:
Student_DF = pd.DataFrame(
{
"Student_Score": [20, 40, 90, 80, 60, 10, 5, 60, 90],
"Std_score_Weight": [1, 2, 3, 1, 2, 3, 1, 2, 3],
"Three_Student": [
"John",
"John",
"John",
"Jack",
"Jack",
"Jack",
"Harry",
"Harry",
"Harry",
],
"Students_Assessment": [
"Quiz_1",
"Quiz_2",
"Quiz_3",
"Quiz_1",
"Quiz_2",
"Quiz_3",
"Quiz_1",
"Quiz_2",
"Quiz_3",
],
}
)
Student_DF
# In[8]:
def Groupby_weighted_avg(values, weighted_value, Group_Cols):
return (values * weighted_value).groupby(Group_Cols).sum() / weighted_value.groupby(
Group_Cols
).sum()
# In[9]:
Groupby_weighted_avg(
Student_DF["Student_Score"],
Student_DF["Std_score_Weight"],
Student_DF["Three_Student"],
)
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn