색인에 Pandas DataFrames 병합
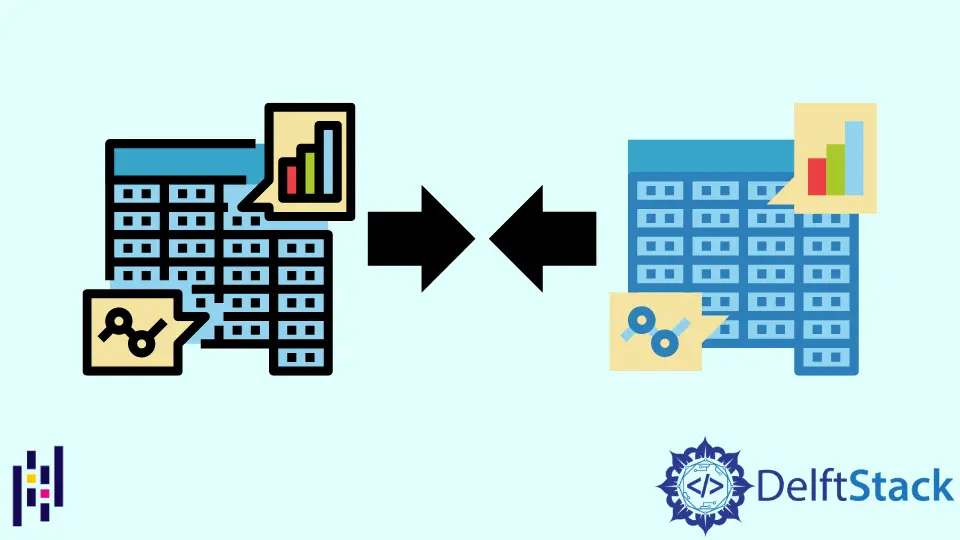
데이터 과학 및 기계 학습의 세계에서 추가 분석을 위해 데이터를 구성, 유지 관리 및 정리하는 작업에 능통해야합니다. 두 개의 DataFrame을 병합하는 것이 이러한 작업의 한 예입니다. Python에서 Pandas 라이브러리를 사용하여 두 개의 DataFrame을 결합하는 것이 쉽습니다.
Pandas는 결합 할 수있는 merge()
및join()
의 두 가지 유용한 함수를 제공합니다. 두 개의 DataFrame. 이 두 방법은 매우 비슷하지만merge()
는 더 다양하고 유연한 것으로 간주됩니다. 또한 최종 DataFrame의 동작을 변경하는 많은 매개 변수를 제공합니다. join()
은 인덱스에서 두 개의 DataFrame을 결합하는 반면merge()
는 두 개의 DataFrame을 병합하는 키 역할을 할 수있는 열을 지정할 수 있습니다.
이 두 함수의 공통 매개 변수 중 하나는 조인 유형을 정의하는 ‘방법’입니다. 기본적으로how
매개 변수는merge()
의 경우inner
이고join()
의 경우left
이지만 둘 다left
,right
,inner
및outer로 변경할 수 있습니다.
. 그들 모두의 차이점을 아는 것이 중요합니다.
두 개의 Pandas DataFrame을 결합하는 동안 하나는 Left DataFrame이고 다른 하나는 Right DataFrame이라고 가정합니다. merge()
와join()
은 모두 키 열의 레코드와 일치합니다. inner
조인은 두 DataFrame 모두에서 일치하는 레코드의 DataFrame을 반환합니다. outer
조인은 양쪽 DataFrames의 모든 요소가있는 병합 된 DataFrame을 생성하여 양쪽에서 누락 된 값에 대해 NaN을 채 웁니다. left
조인은 왼쪽 DataFrame의 모든 요소를 포함하지만 Right DataFrame의 일치하는 레코드 만 포함합니다. left
의 반대는 오른쪽 DataFrame의 모든 요소와 왼쪽 DataFrame의 일치하는 레코드 만있는 right
입니다. 이 모든 것은 아래 코드에서 DataFrames를 결합 할 다음 섹션의 예제 코드에서 더 명확해질 것입니다.
import pandas as pd
import numpy as np
df1 = pd.DataFrame(["a", "b", "d", "e", "h"], index=[1, 2, 4, 5, 7], columns=["C1"])
df2 = pd.DataFrame(
["AA", "BB", "CC", "EE", "FF"], index=[1, 2, 3, 5, 6], columns=["C2"]
)
print(df1)
print(df2)
출력:
C1
1 a
2 b
4 d
5 e
7 h
C2
1 AA
2 BB
3 CC
5 EE
6 FF
merge()
를 사용하여 인덱스에서 두 개의 Pandas DataFrame 결합
색인에서 두 개의 DataFrame을 병합 할 때 merge()
함수의 left_index
및 right_index
매개 변수 값은 True
여야합니다. 다음 코드 예제는 조인 유형으로inner
를 사용하여 두 개의 DataFrame을 결합합니다.
import pandas as pd
import numpy as np
df1 = pd.DataFrame(["a", "b", "d", "e", "h"], index=[1, 2, 4, 5, 7], columns=["C1"])
df2 = pd.DataFrame(
["AA", "BB", "CC", "EE", "FF"], index=[1, 2, 3, 5, 6], columns=["C2"]
)
df_inner = df1.merge(df2, how="inner", left_index=True, right_index=True)
print(df_inner)
출력:
C1 C2
1 a AA
2 b BB
5 e EE
다음 코드는 조인 유형이outer
인 DataFrame을 병합합니다.
import pandas as pd
import numpy as np
df1 = pd.DataFrame(["a", "b", "d", "e", "h"], index=[1, 2, 4, 5, 7], columns=["C1"])
df2 = pd.DataFrame(
["AA", "BB", "CC", "EE", "FF"], index=[1, 2, 3, 5, 6], columns=["C2"]
)
df_outer = df1.merge(df2, how="outer", left_index=True, right_index=True)
print(df_outer)
출력:
C1 C2
1 a AA
2 b BB
3 NaN CC
4 d NaN
5 e EE
6 NaN FF
7 h NaN
보시다시피 조인 유형이 inner
인 병합 된 DataFrame에는 두 DataFrames의 일치하는 레코드 만있는 반면 outer
조인이있는 병합 된 DataFrame에는 누락 된 레코드가 NaN
으로 채워집니다. 이제left
조인을 사용합니다.
import pandas as pd
import numpy as np
df1 = pd.DataFrame(["a", "b", "d", "e", "h"], index=[1, 2, 4, 5, 7], columns=["C1"])
df2 = pd.DataFrame(
["AA", "BB", "CC", "EE", "FF"], index=[1, 2, 3, 5, 6], columns=["C2"]
)
df_left = df1.merge(df2, how="left", left_index=True, right_index=True)
print(df_left)
출력:
C1 C2
1 a AA
2 b BB
4 d NaN
5 e EE
7 h NaN
위의 병합 된 DataFrame에는 왼쪽 DataFrame의 모든 요소와 오른쪽 DataFrame의 일치하는 레코드 만 있습니다. 정반대는 아래와 같이right
조인입니다.
import pandas as pd
import numpy as np
df1 = pd.DataFrame(["a", "b", "d", "e", "h"], index=[1, 2, 4, 5, 7], columns=["C1"])
df2 = pd.DataFrame(
["AA", "BB", "CC", "EE", "FF"], index=[1, 2, 3, 5, 6], columns=["C2"]
)
df_right = df1.merge(df2, how="right", left_index=True, right_index=True)
print(df_right)
출력:
C1 C2
1 a AA
2 b BB
3 NaN CC
5 e EE
6 NaN FF
join()
을 사용하여 인덱스에서 두 개의 Pandas DataFrame 결합
join()
메소드는 색인을 기반으로 두 DataFrame을 결합하며 기본적으로 결합 유형은left
입니다. 항상 올바른 DataFrame의 인덱스를 사용하지만 Left DataFrame의 키를 언급 할 수 있습니다. merge()
에서 언급 한 것과 동일한join()
함수에 대한 조인 유형을 지정할 수 있습니다.
다음 예제는outer
조인 유형이있는 병합 된 DataFrame을 보여줍니다.
import pandas as pd
import numpy as np
df1 = pd.DataFrame(["a", "b", "d", "e", "h"], index=[1, 2, 4, 5, 7], columns=["C1"])
df2 = pd.DataFrame(
["AA", "BB", "CC", "EE", "FF"], index=[1, 2, 3, 5, 6], columns=["C2"]
)
df_outer = df1.join(df2, how="outer")
print(df_outer)
출력:
C1 C2
1 a AA
2 b BB
3 NaN CC
4 d NaN
5 e EE
6 NaN FF
7 h NaN
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn