Kotlin에서 문자열을 Int로 변환하는 다양한 방법
-
toInt()
메서드를 사용하여 KotlinString
을Int
로 변환 -
toIntOrNull()
메서드를 사용하여 KotlinString
을Int
로 변환 -
parseInt()
메서드를 사용하여 KotlinString
을Int
로 변환
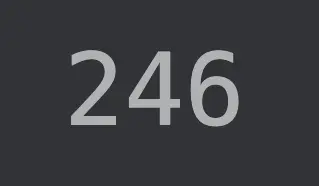
응용 프로그램이나 간단한 프로그램을 만들고 있다고 가정합니다. 프로그램의 함수가 정수 값만 허용하는 경우 NumberFormatException
또는 기타 이러한 예외를 방지하기 위해 String
을 Int
로 변환해야 합니다.
하지만 Kotlin String
을 Int
로 어떻게 변환합니까? 여러 가지 방법이 있습니다. 이 기사에서는 Kotlin에서 String
을 Int
로 변환하는 몇 가지 방법을 설명합니다.
toInt()
메서드를 사용하여 Kotlin String
을 Int
로 변환
String
을 Int
로 변환하기 위해 toInt()
함수를 사용할 수 있습니다. 이를 수행하기 위한 표준 구문은 다음과 같습니다.
String.toInt()
즉, 변환을 위해 String
인스턴스에서 toInt()
메서드를 호출합니다. 아래 예에서는 String
"246"
을 Int
246
으로 변환하기 위해 이 구문을 사용합니다.
fun main() {
val strVal = "246"
val intVal = strVal.toInt()
println(intVal)
}
보시다시피 위의 프로그램은 String
을 Int
로 성공적으로 변환합니다. 그러나 변환할 수 없는 String
이 있는 경우 toInt()
함수는 InvalidFormatException
예외를 throw합니다.
데모를 위해 위의 코드에서 String
"246"
을 String
"246b"
로 변경하고 변환을 시도합니다.
fun main() {
val strVal = "246b"
val intVal = strVal.toInt()
println(intVal)
}
이 프로그램은 "b"
문자를 정수로 변환할 수 없기 때문에 java.lang.NumberFormatException
을 발생시킵니다.
따라서 try-catch
블록 내에서 toInt()
함수를 사용하여 예외를 처리해야 합니다. try-catch
블록을 사용하면 예외가 발생하면 기본 인쇄 값을 할당할 수 있습니다.
다음은 try-catch
블록에 toInt()
함수를 통합하는 방법입니다.
fun main(){
try {
val strVal = "246"
val intVal = strVal.toInt()
println(intVal)
} catch (e: NumberFormatException) {
println("This format is not convertible.")
}
try {
val strVal1 = "246b"
val intVal1 = strVal1.toInt()
println(intVal1)
} catch (e: NumberFormatException) {
println("This format is not convertible.")
}
}
첫 번째 toInt()
메서드는 위 프로그램에서 String
"246"
을 Int
로 변환합니다. 그러나 두 번째 toInt()
함수가 실행되면 try-catch
블록에 의해 처리되는 NumberFormatException
이 발생합니다.
toIntOrNull()
메서드를 사용하여 Kotlin String
을 Int
로 변환
try-catch
블록을 추가하지 않으려면 toIntOrNull()
함수를 대신 사용할 수 있습니다. 이름에서 알 수 있듯이 이 메서드는 변환에 실패하면 null
값을 반환합니다.
따라서 정수 값만 원하고 변환 불가능한 값에 대해 걱정하지 않으려면 toIntOrNull
함수를 사용할 수 있습니다.
fun main(){
val strVal = "246"
val intVal = strVal.toIntOrNull()
println (intVal)
}
변환이 성공하면 Int
값이 표시됩니다. 그러나 변환에 실패하면 String
을 null
로 변환합니다.
fun main(){
val strVal = "246b"
val intVal = strVal.toIntOrNull()
println (intVal)
}
parseInt()
메서드를 사용하여 Kotlin String
을 Int
로 변환
Kotlin String
을 Int
로 변환하는 또 다른 방법은 parseInt()
함수를 사용하는 것입니다. 이는 parseInt()
가 매개변수를 받는다는 주요 차이점을 제외하고 toInt()
메서드와 유사하게 작동합니다.
이 둘의 또 다른 차이점은 toInt
는 String
클래스에 속하고 parseInt()
는 Kotlin Int
클래스의 함수라는 것입니다.
다음은 parseInt()
메서드를 사용하여 Kotlin String
을 Int
로 변환하는 방법입니다.
fun main(){
val strVal = "246"
val intVal = Integer.parseInt(strVal)
println(intVal)
}
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn