C#에서 배열 반전
-
C#
에서 배열을 뒤집는 알고리즘 작성 -
Array.Reverse()
메서드를 사용하여C#
에서 배열 반전 -
Enumerable.Reverse()
메서드를 사용하여C#
에서 배열 반전 - 결론
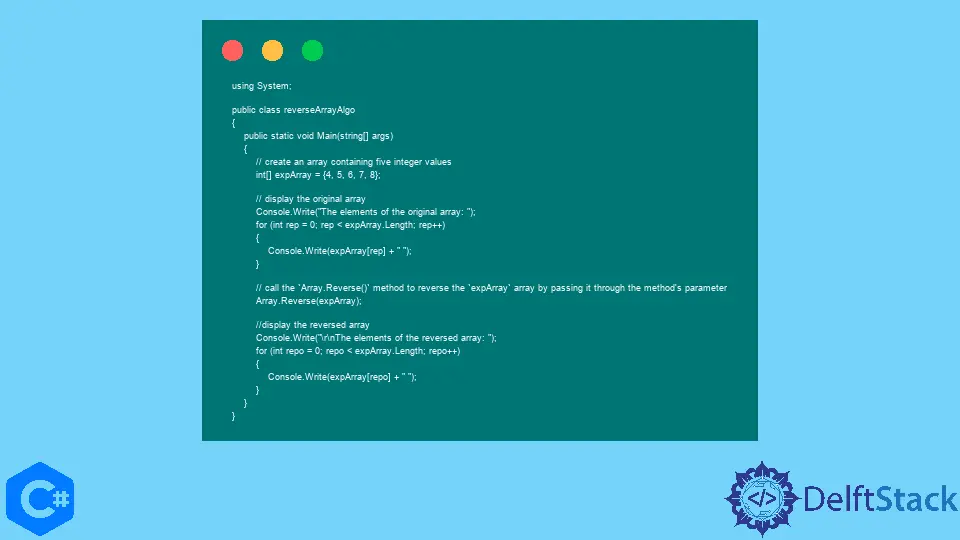
이 자습서에서는 미리 정의된 메서드를 포함하여 C#에서 배열을 반전하는 다양한 방법을 알려줍니다. 일반적으로 temp = arr[0]
배열의 첫 번째 인덱스 값을 저장하려면 temp
변수를 생성하고 arr[0] = arr[와 같이
arr[0]에 두 번째 인덱스 값을 저장해야 합니다. 1]
, temp
값을 arr[1]
에 저장합니다.
이것은 짧은 배열을 뒤집는 일반적인 개념이며 루프는 C#에서 큰 배열에 대한 역방향 작업을 처리하는 데 도움이 될 수 있습니다.
C#
에서 배열을 뒤집는 알고리즘 작성
스와핑 기술은 C#에서 배열을 뒤집을 때 매우 효율적입니다. startIndex
및 endIndex
라는 변수 쌍을 정의하고 해당 값을 각각 0
및 n-1
로 초기화하는 것이 중요합니다. 여기서 n
은 배열의 길이를 나타냅니다.
for
루프. startIndex
값이 endIndex
값보다 작을 때까지 이 단계를 반복하고 배열을 반전된 배열로 인쇄합니다.
일부 보조 공간을 사용하지 않고 값을 교환하는 것만으로 배열을 뒤집는 간단한 방법입니다. 따라서 역배열의 시간 복잡도는 O(n)
이고 공간 복잡도는 O(1)
입니다.
배열 arr.Length / 2
의 전반부를 통해서만 반복해야 합니다. 전체 배열을 반복하는 arr.Length
는 배열이 두 번 반전되어 원래 배열과 동일한 결과를 출력함을 의미합니다.
using System;
public class reverseArrayAlgo {
public static void Main(string[] args) {
// create an array containing five integer values
int[] expArray = { 4, 5, 6, 7, 8 };
// display the original array
Console.Write("The elements of the original array: ");
for (int rep = 0; rep < expArray.Length; rep++) {
Console.Write(expArray[rep] + " ");
}
// call a method that contains the algorithm to reverse the `expArray` array
arrayReverse(expArray, 0, (expArray.Length - 1));
// display the reversed array
Console.Write("\r\nThe elements of the reversed array: ");
for (int repo = 0; repo < expArray.Length; repo++) {
Console.Write(expArray[repo] + " ");
}
}
// create a method to reverse an array
public static void arrayReverse(int[] expArray, int startIndex, int endIndex) {
while (startIndex < endIndex) {
int objTemp = expArray[startIndex];
expArray[startIndex] = expArray[endIndex];
expArray[endIndex] = objTemp;
startIndex++;
endIndex--;
}
}
}
출력:
The elements of the original array: 4 5 6 7 8
The elements of the reversed array: 8 7 6 5 4
Array.Reverse()
메서드를 사용하여 C#
에서 배열 반전
이는 System
네임스페이스의 Array
클래스에 속하며 C#에서 배열을 뒤집는 효율적인 방법입니다. 반전된 배열을 출력으로 받을 수 있도록 형식을 반전시키는 인수로 취하여 1차원 배열을 처리합니다.
새 배열을 만드는 대신 원래 배열을 수정합니다. System.Linq
네임스페이스에서 사용할 수 있는 원래 배열의 수정 사항이 만족스럽지 않은 경우 Enumerable.Reverse()
메서드를 사용할 수 있습니다.
배열 유형의 정적 메서드로 보조 공간이나 배열 없이 지정된 배열의 기존 요소 또는 값을 덮어쓰는 방식으로 작동합니다.
배열이 null이거나 다차원인 경우 여러 유형의 예외가 발생합니다. 인덱스가 배열의 하한보다 작거나, 길이가 0보다 작거나, 인덱스와 길이가 배열에서 유효한 범위를 지정하지 않습니다. 이 메서드를 호출한 후 expArray[repo]
의 요소(여기서 expArray
는 배열을 나타내고 repo
는 배열의 인덱스임)는 expArray[i]
로 이동합니다. 여기서 i
는 다음과 같습니다.
(myArray.Length + myArray.GetLowerBound(0)) - (i - myArray.GetLowerBound(0)) - 1
이 방법은 O(n)
작업이며 n
은 배열의 길이를 나타냅니다. 이 메서드에 전달되는 매개 변수 값 유형은 System.Array
이며 반전할 1차원 배열을 나타냅니다.
예제 코드:
using System;
public class reverseArrayAlgo {
public static void Main(string[] args) {
// create an array containing five integer values
int[] expArray = { 4, 5, 6, 7, 8 };
// display the original array
Console.Write("The elements of the original array: ");
for (int rep = 0; rep < expArray.Length; rep++) {
Console.Write(expArray[rep] + " ");
}
// call the `Array.Reverse()` method to reverse the `expArray` array by passing it through the
// method's parameter
Array.Reverse(expArray);
// display the reversed array
Console.Write("\r\nThe elements of the reversed array: ");
for (int repo = 0; repo < expArray.Length; repo++) {
Console.Write(expArray[repo] + " ");
}
}
}
출력:
The elements of the original array: 4 5 6 7 8
The elements of the reversed array: 8 7 6 5 4
Enumerable.Reverse()
메서드를 사용하여 C#
에서 배열 반전
역순으로 요소의 새 시퀀스를 생성하여 원본 또는 기본 배열을 수정하지 않고 배열의 요소 순서를 역순으로 만드는 유용한 방법입니다. Enumerable.Reverse()
메서드를 호출하여 배열 요소의 순서를 반전합니다. 다른 메서드나 사용자 지정 역방향 알고리즘보다 읽기 쉽고 편리합니다.
이는 System.Linq
네임스페이스에 속하며 입력 시퀀스에 해당하는 요소가 역순으로 있는 시퀀스를 반환합니다. 역방향 연산을 수행하는 데 필요한 모든 정보를 저장하는 값은 지연 실행으로 구현된 즉시 반환 개체입니다.
foreach
를 사용하거나 Getnumerator
메소드를 직접 호출하여 이 메소드의 완벽한 실행을 위해 개체를 열거할 수 있습니다. OrderBy
와 달리 정렬 방법인 Enumerable.Array()
는 순서를 결정할 때 실제 값을 고려하지 않습니다.
예제 코드:
using System;
using System.Linq;
public class reverseArrayAlgo {
public static void Main(string[] args) {
// create an array containing five integer values
int[] expArray = { 4, 5, 6, 7, 8 };
// display the original array
Console.Write("The elements of the original array: ");
for (int rep = 0; rep < expArray.Length; rep++) {
Console.Write(expArray[rep] + " ");
}
// call the `Enumerable.Reverse()` method to reverse the `expArray` array by passing it through
// the method's parameter
int[] reverse = Enumerable.Reverse(expArray).ToArray();
// another alternative to display the reverse array
// Console.WriteLine(String.Join(',', reverse));
// display the reversed array
Console.Write("\r\nThe elements of the reversed array: ");
for (int repo = 0; repo < reverse.Length; repo++) {
Console.Write(reverse[repo] + " ");
}
}
}
출력:
The elements of the original array: 4 5 6 7 8
The elements of the reversed array: 8 7 6 5 4
결론
이 자습서에서는 C#에서 배열을 뒤집는 세 가지 방법에 대해 설명했습니다. 이 자습서에는 깊은 이해를 위해 실행 가능한 C# 코드의 단순화되고 최적화된 예제가 포함되어 있습니다.
모든 리버스 방법에는 고유한 특성과 이점이 있습니다. 귀하의 필요에 가장 적합한 것을 선택하십시오.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub