Invertir una matriz en C#
-
Escribir un Algoritmo para Invertir un Array en
C#
-
Utilice el método
Array.Reverse()
para invertir una matriz enC#
-
Utilice el método
Enumerable.Reverse()
para invertir una matriz enC#
- Conclusión
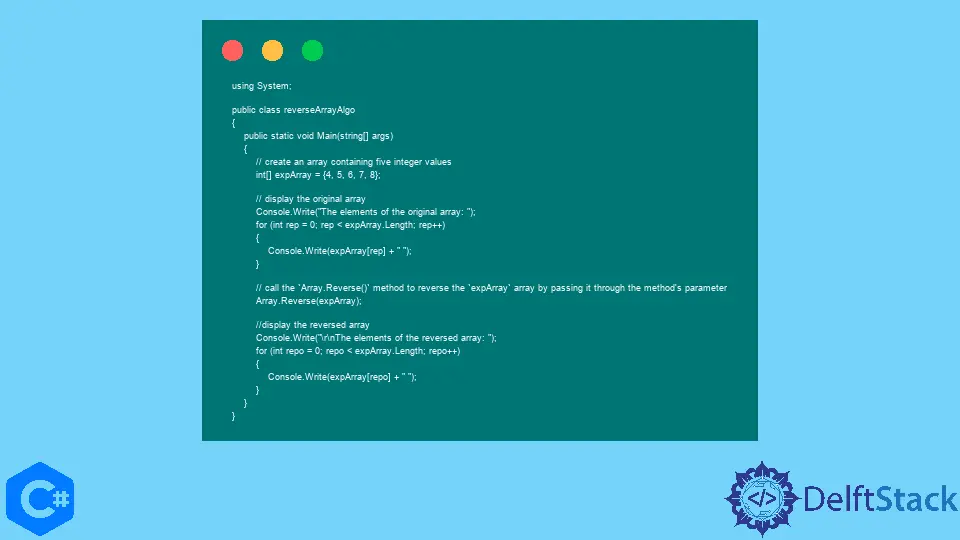
Este tutorial le enseñará diferentes formas de invertir una matriz en C#, incluidos los métodos predefinidos. Generalmente requiere la creación de una variable temp
para almacenar el primer valor de índice de una matriz temp = arr[0]
, almacenar el segundo valor de índice en arr[0]
como arr[0] = arr[1]
, y almacenar el valor temp
en arr[1]
.
Es un concepto general de invertir una matriz corta, y los bucles pueden ayudar a manejar las operaciones inversas en matrices grandes en C#.
Escribir un Algoritmo para Invertir un Array en C#
El arte del intercambio es extremadamente eficiente para invertir una matriz en C#. Es importante definir un par de variables llamadas startIndex
y endIndex
e inicializar sus valores con 0
y n-1
, respectivamente, donde n
representa la longitud de una matriz.
Puede implementar el concepto de intercambiar valores de ambas variables e incrementar el valor de startIndex
en un medio +1
y disminuir el endIndex
en uno (-1
) en un algoritmo en C# usando el for
bucle. Repita este paso hasta que el valor startIndex
sea menor que el valor endIndex
e imprima la matriz como una matriz invertida.
Es un enfoque simple para invertir una matriz sin usar algún espacio auxiliar solo intercambiando valores. Por lo tanto, la complejidad temporal del arreglo inverso es O(n)
, y su complejidad espacial es O(1)
.
Debe iterar solo a través de la primera mitad de una matriz arr.Length / 2
. Al iterar a través de toda la matriz, arr.Length
significa que la matriz se invertirá dos veces y generará el mismo resultado que la matriz original.
using System;
public class reverseArrayAlgo {
public static void Main(string[] args) {
// create an array containing five integer values
int[] expArray = { 4, 5, 6, 7, 8 };
// display the original array
Console.Write("The elements of the original array: ");
for (int rep = 0; rep < expArray.Length; rep++) {
Console.Write(expArray[rep] + " ");
}
// call a method that contains the algorithm to reverse the `expArray` array
arrayReverse(expArray, 0, (expArray.Length - 1));
// display the reversed array
Console.Write("\r\nThe elements of the reversed array: ");
for (int repo = 0; repo < expArray.Length; repo++) {
Console.Write(expArray[repo] + " ");
}
}
// create a method to reverse an array
public static void arrayReverse(int[] expArray, int startIndex, int endIndex) {
while (startIndex < endIndex) {
int objTemp = expArray[startIndex];
expArray[startIndex] = expArray[endIndex];
expArray[endIndex] = objTemp;
startIndex++;
endIndex--;
}
}
}
Producción :
The elements of the original array: 4 5 6 7 8
The elements of the reversed array: 8 7 6 5 4
Utilice el método Array.Reverse()
para invertir una matriz en C#
Pertenece a la clase Array
en el espacio de nombres System
y es una forma eficiente de invertir una matriz en C#. Procesa la matriz unidimensional tomándola como argumento para invertir su formato de modo que pueda recibir una matriz invertida como salida.
En lugar de crear una nueva matriz, modifica la matriz original. Puede usar el método Enumerable.Reverse()
si no está satisfecho con la modificación de la matriz original disponible en el espacio de nombres System.Linq
.
Como método estático en el tipo de matriz, funciona sobrescribiendo los elementos o valores existentes de la matriz especificada sin ningún espacio o matriz auxiliar.
Lanza múltiples tipos de excepciones cuando una matriz es nula o multidimensional; el índice es menor que el límite inferior de la matriz, la longitud es menor que cero o el índice y la longitud no especifican un rango válido en la matriz. Después de una llamada a este método, los elementos en expArray[repo]
(donde expArray
representa una matriz y repo
es un índice en la matriz) se mueven a expArray[i]
donde i
es igual a:
(myArray.Length + myArray.GetLowerBound(0)) - (i - myArray.GetLowerBound(0)) - 1
Este método es una operación O(n)
donde n
representa la longitud de una matriz. El tipo de valor del parámetro pasado a este método es System.Array
, que representa la matriz unidimensional a invertir.
Código de ejemplo:
using System;
public class reverseArrayAlgo {
public static void Main(string[] args) {
// create an array containing five integer values
int[] expArray = { 4, 5, 6, 7, 8 };
// display the original array
Console.Write("The elements of the original array: ");
for (int rep = 0; rep < expArray.Length; rep++) {
Console.Write(expArray[rep] + " ");
}
// call the `Array.Reverse()` method to reverse the `expArray` array by passing it through the
// method's parameter
Array.Reverse(expArray);
// display the reversed array
Console.Write("\r\nThe elements of the reversed array: ");
for (int repo = 0; repo < expArray.Length; repo++) {
Console.Write(expArray[repo] + " ");
}
}
}
Producción :
The elements of the original array: 4 5 6 7 8
The elements of the reversed array: 8 7 6 5 4
Utilice el método Enumerable.Reverse()
para invertir una matriz en C#
Es una forma útil de invertir el orden de los elementos en una matriz sin modificar la matriz original o subyacente mediante la creación de una nueva secuencia de elementos en el orden inverso. Invoque el método Enumerable.Reverse()
para invertir el orden de los elementos de una matriz, que es más conveniente y más fácil de leer que cualquier otro método o algoritmo inverso personalizado.
Pertenece al espacio de nombres System.Linq
y devuelve una secuencia cuyos elementos corresponden a la secuencia de entrada en orden inverso. Su valor, que almacena toda la información necesaria para realizar la operación inversa, es un objeto de retorno inmediato implementado por ejecución diferida.
Puedes usar el método foreach
o llamar directamente al método Getnumerator
para enumerar el objeto para la perfecta ejecución de este método. A diferencia de OrderBy
, Enumerable.Array()
como método de clasificación no tiene en cuenta los valores reales al determinar el orden.
Código de ejemplo:
using System;
using System.Linq;
public class reverseArrayAlgo {
public static void Main(string[] args) {
// create an array containing five integer values
int[] expArray = { 4, 5, 6, 7, 8 };
// display the original array
Console.Write("The elements of the original array: ");
for (int rep = 0; rep < expArray.Length; rep++) {
Console.Write(expArray[rep] + " ");
}
// call the `Enumerable.Reverse()` method to reverse the `expArray` array by passing it through
// the method's parameter
int[] reverse = Enumerable.Reverse(expArray).ToArray();
// another alternative to display the reverse array
// Console.WriteLine(String.Join(',', reverse));
// display the reversed array
Console.Write("\r\nThe elements of the reversed array: ");
for (int repo = 0; repo < reverse.Length; repo++) {
Console.Write(reverse[repo] + " ");
}
}
}
Producción :
The elements of the original array: 4 5 6 7 8
The elements of the reversed array: 8 7 6 5 4
Conclusión
Este tutorial analizó tres formas de invertir una matriz en C#. Este tutorial contiene ejemplos simplificados y optimizados del código C# ejecutable para una comprensión profunda.
Cada método inverso tiene sus propiedades y ventajas únicas sobre el otro. Elige el que mejor se adapte a tus necesidades.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub