C++에서 디렉터리의 파일 목록을 가져 오는 방법
-
std::filesystem::directory_iterator
를 사용하여 디렉토리의 파일 목록 가져 오기 -
opendir/readdir
함수를 사용하여 디렉토리의 파일 목록 가져 오기 -
std::filesystem::recursive_directory_iterator
를 사용하여 모든 하위 디렉토리의 파일 목록을 가져옵니다
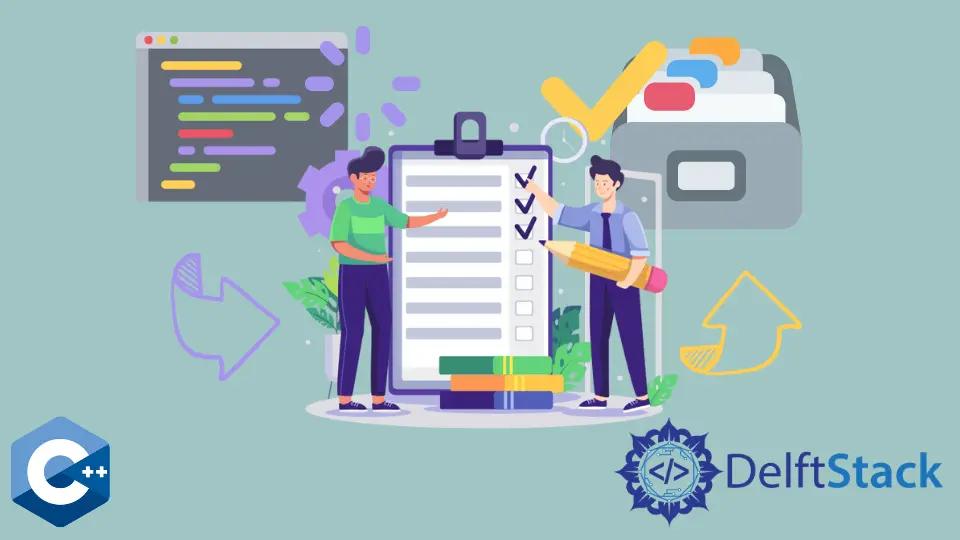
이 기사에서는 C++로 지정된 디렉토리에있는 파일 목록을 가져 오는 방법을 소개합니다.
std::filesystem::directory_iterator
를 사용하여 디렉토리의 파일 목록 가져 오기
이 메서드는 C++ 17에 추가 된<filesystem>
라이브러리의 일부입니다. 일부 오래된 컴파일러는이 메서드를 지원하지 않을 수 있지만 이는 디렉터리의 파일 목록을 가져 오는 네이티브 C++ 솔루션입니다. directory_iterator
는 범위 기반for
루프에서 사용되며 디렉토리 경로를 인수로 사용합니다. 이 예에서는 루트 디렉토리의 경로를 지정하지만 유효한 경로를 전달하려면 시스템에 따라이 변수를 수정해야합니다.
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::directory_iterator;
int main() {
string path = "/";
for (const auto& file : directory_iterator(path)) cout << file.path() << endl;
return EXIT_SUCCESS;
}
출력 (디렉토리에 따라 다름):
Archive2| lib| .| ..| lib64| Archive| dev| var| bin| usr| etc| tmp| proc|
opendir/readdir
함수를 사용하여 디렉토리의 파일 목록 가져 오기
이 방법은 훨씬 더 장황하지만 파일 시스템 조작을위한 신뢰할 수있는 대안입니다.
opendir
/readdir
함수를 사용하려면dirent.h
헤더 파일을 포함해야합니다.
opendir
은 이름으로 지정된 디렉토리를 열고DIR*
의 포인터를 반환합니다.
readdir
은DIR *
인수에서 작동하며dirent
라는 특수 구조체를 반환합니다 (전체 정보보기. ).
nullptr
과 비교하여while
루프에readdir
호출을 넣으면 디렉토리 반복자 역할을하며dirent
구조체를 반환하는 각각을 통해 파일 이름에 액세스 할 수 있습니다.
#include <dirent.h>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
int main() {
DIR *dir;
struct dirent *diread;
vector<char *> files;
if ((dir = opendir("/")) != nullptr) {
while ((diread = readdir(dir)) != nullptr) {
files.push_back(diread->d_name);
}
closedir(dir);
} else {
perror("opendir");
return EXIT_FAILURE;
}
for (auto file : files) cout << file << "| ";
cout << endl;
return EXIT_SUCCESS;
}
opendir
및readdir
함수는 디렉토리의 오류 / 끝에NULL
포인터를 반환하므로 대부분의 if
문은 예외 처리 메커니즘으로 작동합니다.
std::filesystem::recursive_directory_iterator
를 사용하여 모든 하위 디렉토리의 파일 목록을 가져옵니다
이 방법은 여러 하위 디렉터리에서 특정 파일 이름을 검색해야 할 때 유용합니다. 메서드는directory_iterator
와 동일하게 유지됩니다. 범위 기반 루프를 사용하여 루프를 반복하고 필요에 따라 파일을 조작하면됩니다.
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::recursive_directory_iterator;
int main() {
string path = "./";
for (const auto& file : recursive_directory_iterator(path))
cout << file.path() << endl;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook