C++에서 콘솔 색상을 변경하는 방법
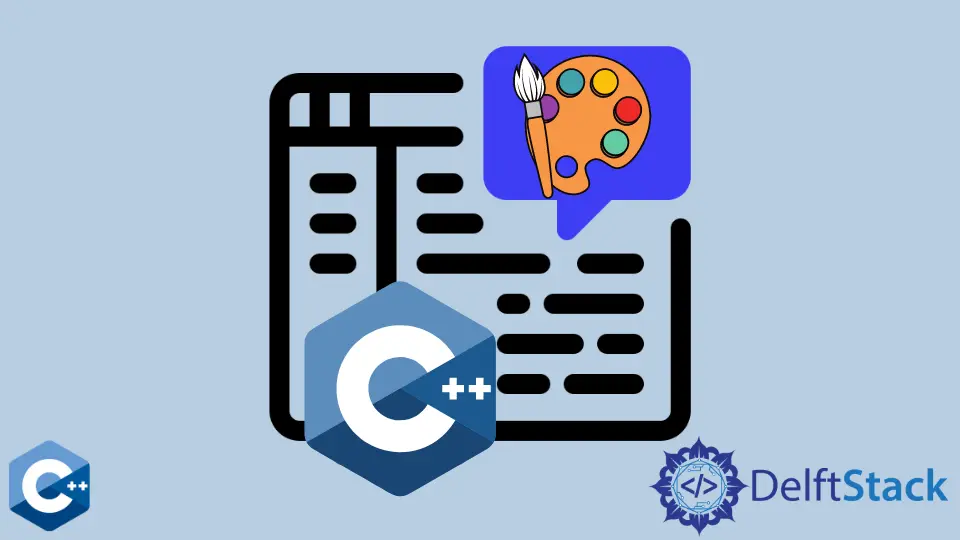
이 기사에서는 C++에서 콘솔 색상을 변경하는 방법에 대한 몇 가지 방법을 설명합니다.
ANSI 이스케이프 코드를 사용하여 콘솔 색상 변경
색상은 표준 C++ 라이브러리의 일부가 아니며 콘솔 관련 기능은 대부분 운영 체제에서 처리하므로 출력 스트림에 색상을 추가하는 기본 언어 기능이 없습니다. 그러나 우리는 텍스트 출력의 색상을 처리하는 몇 가지 플랫폼 별 방법에 대해 논의합니다.
ANSI 이스케이프 코드는이 문제를 해결하기위한 비교적 이식 가능한 방법입니다. 이스케이프 코드는 주로 ASCII 이스케이프 문자와 대괄호 문자와 매개 변수로 시작하는 바이트 시퀀스입니다. 이러한 문자는 출력 텍스트에 포함되며 콘솔은 시퀀스를 표시 할 텍스트가 아닌 명령으로 해석합니다. ANSI 코드에는 여러 색상 형식이 포함되어 있으며 자세한 내용은 이 페이지에서 확인할 수 있습니다. 다음 코드 예제에서는 여러 색상 문자를 매크로로 정의하고 나중에printf
문자열 인수에 이러한 기호를 포함하는 방법을 보여줍니다. printf
는 첫 번째 매개 변수 위치에 전달 된 여러 개의 큰 따옴표 문자열을 연결합니다.
#include <iostream>
#define NC "\e[0m"
#define RED "\e[0;31m"
#define GRN "\e[0;32m"
#define CYN "\e[0;36m"
#define REDB "\e[41m"
using std::cout;
using std::endl;
int main(int argc, char *argv[]) {
if (argc < 2) {
printf(RED "ERROR" NC
": provide argument as follows -> ./program argument\n");
exit(EXIT_FAILURE);
}
printf(GRN "SUCCESS!\n");
return EXIT_SUCCESS;
}
출력 (프로그램 인수 없음) :
ERROR: provide argument as follows -> ./program argument
출력 (프로그램 인수 포함) :
SUCCESS!
또는 cout
호출과 함께 동일한 이스케이프 코드를 사용할 수 있습니다. <<
스트림 삽입 연산자를 여러 번 사용할 필요는 없으며 자동으로 결합되므로 문자열 리터럴과 함께 매크로 기호를 결합하기 만하면됩니다.
#include <iostream>
#define NC "\e[0m"
#define RED "\e[0;31m"
#define GRN "\e[0;32m"
#define CYN "\e[0;36m"
#define REDB "\e[41m"
using std::cout;
using std::endl;
int main() {
cout << CYN "Some cyan colored text" << endl;
cout << REDB "Add red background" NC << endl;
cout << "reset to default colors with NC" << endl;
return EXIT_SUCCESS;
}
출력:
Some cyan colored text
Add red background
reset to default with NC
SetConsoleTextAttribute()
메서드를 사용하여 C++에서 콘솔 색상 변경
SetConsoleTextAttribute
는 다른 매개 변수를 사용하여 출력 텍스트 색상을 설정하는 Windows API 메서드입니다. 이 함수는WriteFile
또는WriteConsole
함수에 의해 콘솔 화면 버퍼에 기록되는 문자의 속성을 설정합니다. 캐릭터 속성에 대한 전체 설명은이 페이지에서 확인할 수 있습니다.
#include << windows.h>>
#include <iostream>
#include <string>
using std::cout;
using std::endl;
int main() {
std::string str("HeLLO tHERe\n");
DWORD bytesWritten = 0;
HANDLE cout_handle = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleTextAttribute(console_handle,
FOREGROUND_RED | BACKGROUND_INTENSITY);
WriteFile(cout_handle, str.c_str(), str.size(), &bytesWritten, NULL);
return EXIT_SUCCESS;
}
출력:
Some red colored text
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook