C++의 벡터에서 요소 인덱스 찾기
- 사용자 지정 함수를 사용하여 C++의 벡터에서 요소 인덱스 찾기
-
std::find
알고리즘을 사용하여 C++의 벡터에서 요소 인덱스 찾기 -
std::find_if
알고리즘을 사용하여 C++의 벡터에서 요소 인덱스 찾기
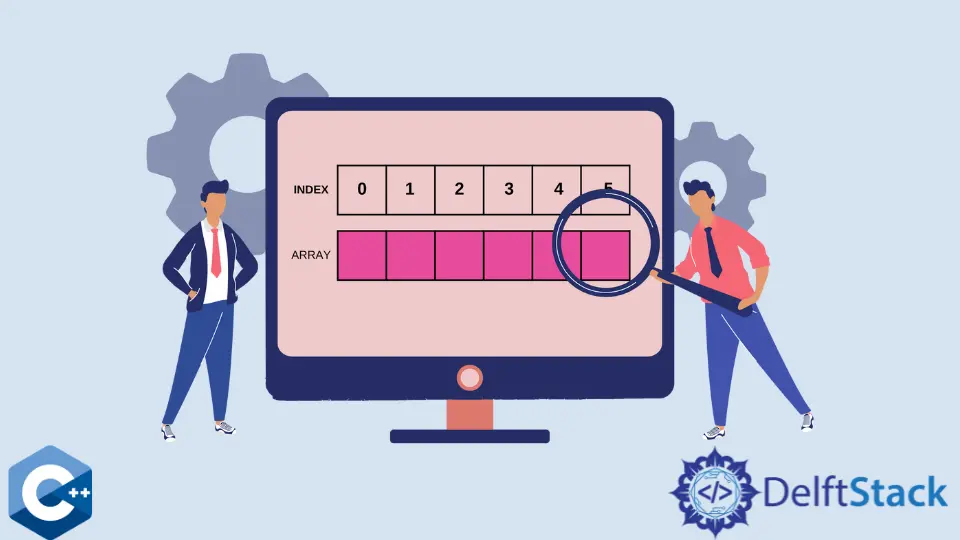
이 기사에서는 C++에서 벡터에서 요소 인덱스를 찾는 방법에 대한 몇 가지 방법을 설명합니다.
사용자 지정 함수를 사용하여 C++의 벡터에서 요소 인덱스 찾기
사용자 정의 선형 검색 기능을 사용하여벡터
에서 주어진 요소의 위치를 찾을 수 있습니다. 이것은이 문제를 해결하기위한 매우 비효율적 인 방법입니다. 다음 예제 코드에서 정수의벡터
를 선언하고 호출이 성공하면 출력하는 임의의 요소 위치를 찾습니다.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int findIndex(const vector<int> &arr, int item) {
for (auto i = 0; i < arr.size(); ++i) {
if (arr[i] == item) return i;
}
return -1;
}
int main(int argc, char *argv[]) {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto pos = findIndex(arr, 32);
pos != -1
? cout << "Found the element " << 32 << " at position " << pos << endl
: cout << "Could not found the element " << 32 << " in vector" << endl;
exit(EXIT_SUCCESS);
}
출력:
Could not found the element 32 in vector
std::find
알고리즘을 사용하여 C++의 벡터에서 요소 인덱스 찾기
또는 STL 라이브러리의 일부인std::find
알고리즘을 사용할 수 있습니다. 이 함수는 조건을 만족하는 첫 번째 요소에 대한 반복자를 반환합니다. 반면에 요소가 발견되지 않으면 알고리즘은 범위의 마지막 요소를 반환합니다. 하지만 반환 된 이터레이터는 위치를 계산하기 위해begin
이터레이터에 의해 감소되어야합니다.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int findIndex2(const vector<int> &arr, int item) {
auto ret = std::find(arr.begin(), arr.end(), item);
if (ret != arr.end()) return ret - arr.begin();
return -1;
}
int main(int argc, char *argv[]) {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto pos2 = findIndex2(arr, 10);
pos2 != -1
? cout << "Found the element " << 10 << " at position " << pos2 << endl
: cout << "Could not found the element " << 10 << " in vector" << endl;
exit(EXIT_SUCCESS);
}
출력:
Found the element 10 at position 9
std::find_if
알고리즘을 사용하여 C++의 벡터에서 요소 인덱스 찾기
요소의 색인을 찾는 또 다른 방법은std::find_if
알고리즘을 호출하는 것입니다. 세 번째 인수가 반복되는 각 요소를 평가하기위한 술어 표현식이 될 수 있다는 점을 제외하면std::find
와 유사합니다. 표현식이 true를 반환하면 알고리즘이 반환됩니다. 람다 식을 세 번째 인수로 전달하여 요소가10
과 같은지 확인합니다.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main(int argc, char *argv[]) {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto ret =
std::find_if(arr.begin(), arr.end(), [](int x) { return x == 10; });
if (ret != arr.end())
cout << "Found the element " << 10 << " at position " << ret - arr.begin()
<< endl;
exit(EXIT_SUCCESS);
}
출력:
Found the element 10 at position 9
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook