Trova l'indice degli elementi in Vector in C++
- Usa la funzione personalizzata per trovare l’indice degli elementi nel vettore in C++
-
Usa l’algoritmo
std::find
per trovare l’indice degli elementi in Vector in C++ -
Usa l’algoritmo
std::find_if
per trovare l’indice degli elementi in Vector in C++
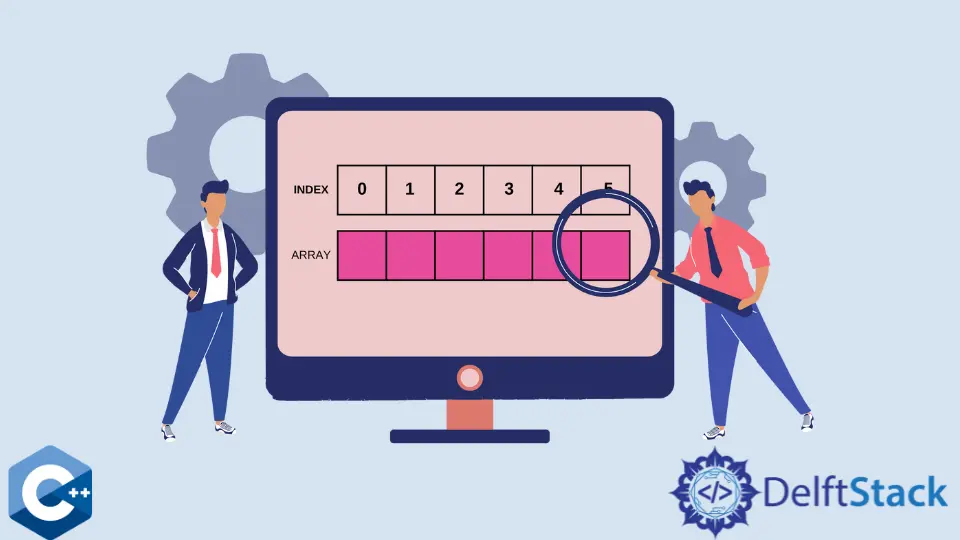
Questo articolo spiegherà diversi metodi su come trovare l’indice degli elementi nel vettore in C++.
Usa la funzione personalizzata per trovare l’indice degli elementi nel vettore in C++
Possiamo usare una funzione di ricerca lineare personalizzata per trovare la posizione di un dato elemento nel vettore
. Nota che questo è un metodo piuttosto inefficiente per risolvere questo problema. Nel seguente codice di esempio, dichiariamo un vettore
di numeri interi e cerchiamo una posizione arbitraria dell’elemento, che emettiamo se la chiamata ha successo.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int findIndex(const vector<int> &arr, int item) {
for (auto i = 0; i < arr.size(); ++i) {
if (arr[i] == item) return i;
}
return -1;
}
int main(int argc, char *argv[]) {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto pos = findIndex(arr, 32);
pos != -1
? cout << "Found the element " << 32 << " at position " << pos << endl
: cout << "Could not found the element " << 32 << " in vector" << endl;
exit(EXIT_SUCCESS);
}
Produzione:
Could not found the element 32 in vector
Usa l’algoritmo std::find
per trovare l’indice degli elementi in Vector in C++
In alternativa, possiamo usare l’algoritmo std::find
che fa parte della libreria STL. Questa funzione restituisce l’iteratore al primo elemento che soddisfa la condizione. D’altra parte, se non viene trovato alcun elemento, l’algoritmo restituisce l’ultimo elemento dell’intervallo. Attenzione però, l’iteratore restituito dovrebbe essere decrementato dall’iteratore begin
per calcolare la posizione.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int findIndex2(const vector<int> &arr, int item) {
auto ret = std::find(arr.begin(), arr.end(), item);
if (ret != arr.end()) return ret - arr.begin();
return -1;
}
int main(int argc, char *argv[]) {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto pos2 = findIndex2(arr, 10);
pos2 != -1
? cout << "Found the element " << 10 << " at position " << pos2 << endl
: cout << "Could not found the element " << 10 << " in vector" << endl;
exit(EXIT_SUCCESS);
}
Produzione:
Found the element 10 at position 9
Usa l’algoritmo std::find_if
per trovare l’indice degli elementi in Vector in C++
Un altro metodo per trovare l’indice dell’elemento è invocare l’algoritmo std::find_if
. È simile a std::find
tranne per il fatto che il terzo argomento può essere un’espressione di predicato per valutare ogni elemento iterato. Se l’espressione restituisce true, l’algoritmo tornerà. Si noti che passiamo l’espressione lambda come terzo argomento, che controlla se l’elemento è uguale a 10
.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main(int argc, char *argv[]) {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto ret =
std::find_if(arr.begin(), arr.end(), [](int x) { return x == 10; });
if (ret != arr.end())
cout << "Found the element " << 10 << " at position " << ret - arr.begin()
<< endl;
exit(EXIT_SUCCESS);
}
Produzione:
Found the element 10 at position 9
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook