Ruby での try...catch
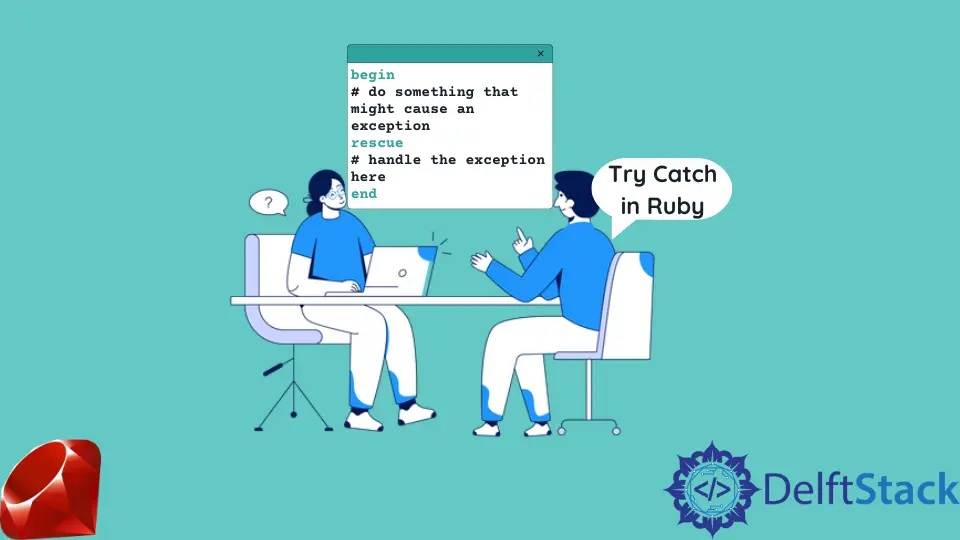
例外は、プログラムの実行中に発生する望ましくないイベントです。例として、プログラムが数値をゼロで除算しようとした場合の ZeroDivisionError
があります。例外をレスキューすることは、プログラムをクラッシュさせないように、例外を適切に処理する方法です。
Ruby の例外は通常、begin
と rescue
の 2つの句で構成されています。begin
句は Python または他の同様のプログラミング言語の try
と同等ですが、rescue
は catch
と同等です。以下は構文です。
begin
# do something that might cause an exception
rescue
# handle the exception here
end
商品の販売価格と原価が与えられた場合に利益率を計算する簡単なプログラムを書いてみましょう。
コード例:
def profit_percentage(selling_price, cost_price)
profit = selling_price - cost_price
"#{(profit.fdiv(cost_price) * 100)}%"
end
puts profit_percentage(30, 20)
puts profit_percentage(30, nil)
出力:
50.0%
nil can't be coerced into Integer (TypeError)
上記の出力でわかるように、profit_percentage
の 2 回目の呼び出しでは、cost_price
として nil
を渡したため、エラーが発生しました。次に、この例外を救済するためのさまざまな方法を説明しましょう。
Ruby で一般的なエラーメッセージを表示してレスキューする
一般的なエラーメッセージでレスキューすることは、例外の根本的な原因を明らかにしないため、必ずしも役立つとは限りません。
コード例:
def profit_percentage(selling_price, cost_price)
begin
profit = selling_price - cost_price
"#{(profit.fdiv(cost_price) * 100)}%"
rescue
"An unknown error occurred."
end
end
puts profit_percentage(30, nil)
出力:
An unknown error occurred.
Ruby の例外からのメッセージでレスキューする
このメソッドは、発生した例外のタイプではなく、エラーメッセージだけに関心がある場合に便利です。
コード例:
def profit_percentage(selling_price, cost_price)
begin
profit = selling_price - cost_price
"#{(profit.fdiv(cost_price) * 100)}%"
rescue => e
e
end
end
puts profit_percentage(30, nil)
出力:
nil can't be coerced into Integer
Ruby で特定の種類の例外をレスキューする
特定の種類の例外のみを処理することに関心がある場合に役立ちます。
コード例:
def profit_percentage(selling_price, cost_price)
begin
profit = selling_price - cost_price
"#{(profit.fdiv(cost_price) * 100)}%"
rescue TypeError
"An argument of invalid type was detected"
end
end
puts profit_percentage(30, nil)
出力:
An argument of invalid type was detected
また、ensure
と呼ばれる別のオプションの句があることにも言及する価値があります。これは、例外が発生したかどうかに関係なく、常に何らかのコードを実行する必要がある場合に役立ちます。
コード例:
def profit_percentage(selling_price, cost_price)
begin
profit = selling_price - cost_price
puts "#{(profit.fdiv(cost_price) * 100)}%"
rescue => e
puts e
ensure
puts "Done calculating profit %"
end
end
profit_percentage(30, 20)
profit_percentage(30, nil)
出力:
50.0%
Done calculating profit %
nil can't be coerced into Integer
Done calculating profit %
上記の出力に示されているように、ensure
句のコードは、profit_percentage
の両方の呼び出しでトリガーされました。この句は通常、例外が発生したかどうかに関係なく、いくつかのファイル操作を実行した後にファイルを閉じる必要がある場合に使用されます。