React Router を使用して props を Handler コンポーネントに渡す
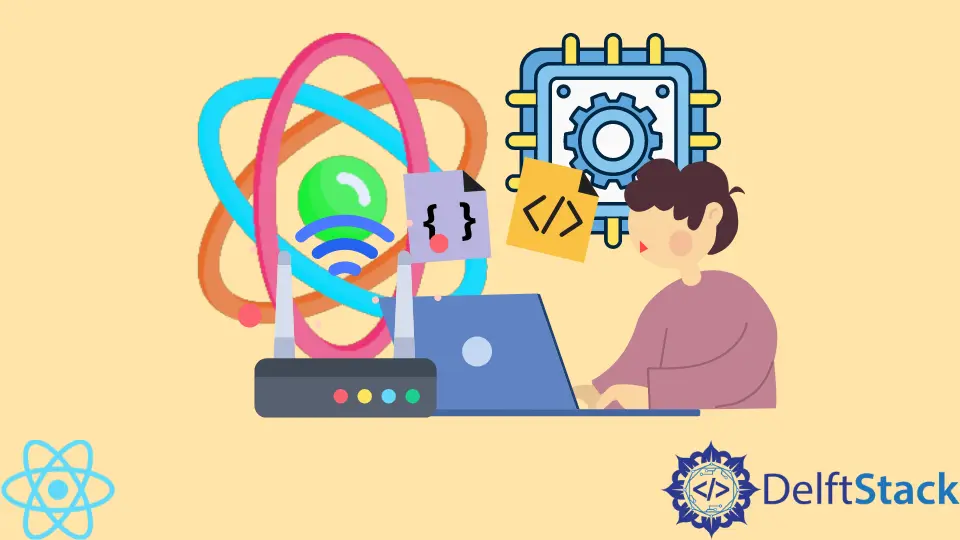
反応ルーターは、1つの Web ページを別の Web ページにリンクするという主要な機能を実行します。 マルチページ アプリを構築する場合、react-router を適用してページをリンクします。
ただし、React ルーターはもう 1つの機能を実行します。 また、あるリンクから別のリンクに状態または props{property}
を渡すこともできます。
このアプローチでは、1つのリンクを使用して別のリンクの状態を制御したり、前のページのコンテンツ全体をレンダリングすることなく、あるページの要素の一部を別のページと共有したりできます。
React Router を使用して props を別のコンポーネントに渡す
たとえば、React Router を使用して Page 4 コンポーネントから Page 5 コンポーネントの URL を調整する prop を渡したいとします。
新しいプロジェクト フォルダーを作成し、React Router を npm install react-router
でインストールし、ルーター DOM を npm install react-router-dom
でインストールします。 次に、フォルダーを作成してpages
という名前を付け、Page4.js
とPage5.js
という 2つのコンポーネントを作成します。
次に、次のように App.js
ファイルをコーディングすることから始めます。
コード スニペット - App.js
:
import React from 'react';
import { BrowserRouter, Route, Routes } from 'react-router-dom';
import Page4 from './pages/Page4';
import Page5 from './pages/Page5';
function App(props) {
return (
<BrowserRouter>
<Routes>
<Route exact path="/" element={<Page4/>} />
<Route exact path="/page4" element={<Page4 />} />
<Route path="/page5/:type" element={<Page5/>} />
</Routes>
</BrowserRouter>
);
}
export default App;
ここでは、両方のコンポーネントを App.js
にリンクし、Page5
ページが :type
属性を使用して任意のタイプの prop を受け入れるようにします。
次に、Page4.js
ファイルに、これらのコードを挿入します。
コード スニペット - Page4.js
:
import React from 'react';
import { Link } from 'react-router-dom';
function Page4(props) {
return (
<div>
<h1>Title of Page 4</h1>
<hr />
<Link to={{
pathname: "/page5/parameter-data",
state: { stateParam: true }
}}>Move to Next Page</Link>
</div>
);
}
export default Page4;
Page4
を Page5
にリンクすると同時に、URL を更新するステートも追加しました。 通常は http://localhost:3000/page5
だけが表示されますが、Page4
から追加したように http://localhost:3000/page5/parameter-data
が表示されるはずです。
次に、Page5
で、このように Page4
にリンクするコードを作成します。
コード スニペット - Page5.js
:
import React from 'react';
import { Link, useParams, useLocation } from 'react-router-dom';
function Page5(props) {
const { type } = useParams();
return (
<div>
<h1>Title of Page 5</h1>
<hr />
<Link to={"/page4"}>Move to Prev Page</Link>
</div>
);
}
export default Page5;
また、Page4
から props を受け取るために const {type}
を追加しました。
出力:
まとめ
このアプローチは、コードの干し草の山で失いたくない優れた機能や小道具を追加したい場合に効果的です。 したがって、それ用に別のコンポーネントを作成し、prop または state を渡すときに必要なページにリンクすることができます。
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn関連記事 - React Router
- React JS での条件付きルーティング
- React Router 4 正規表現パス
- React Router を使用して前のページに戻る
- React ルーターの動的ルート
- React の HashRouter コンポーネント