Python - ログ ファイルを追跡し、ブロッキングと非ブロッキングの Tail 関数を比較する
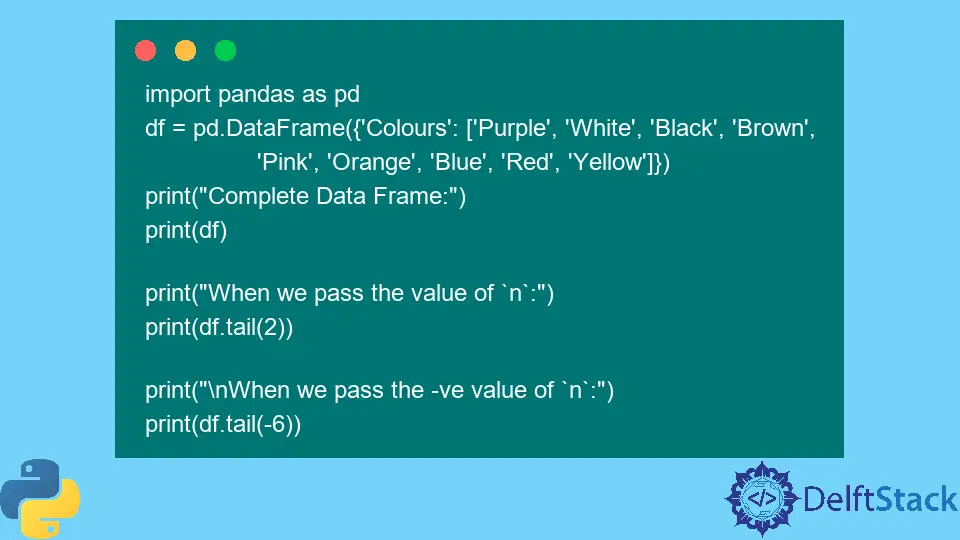
今日のチュートリアルでは、Python の tail()
関数の概要を説明し、ログ ファイルを追跡する方法の動作とデモンストレーションを行います。
また、Python のブロッキングおよび非ブロッキングの tail 関数を比較し、違いを強調しています。
Python tail()
関数の概要
Python では、tail()
関数を使用すると、データ フレームの最後の 5 行がデフォルトで表示されます。 入力パラメーターは 1つだけで、行数です。
このオプションを使用すると、特定の行数を表示できます。 さらに、次の例に示すように、tail()
関数は負の数も受け入れます。
このような状況では、すべての行が返されますが、最初の行は返されません。 head()
と tail()
の主な違いは、空のパラメーターが渡されると、head()
と tail()
は両方とも 5 行を返すことです。
head()
および tail()
関数は順序付けられたデータを生成するのに対し、sample()
は順序付けされていないデータを生成することに注意してください。
tail()
関数の構文:
dataframe.tail(n=5)
Python での Tail()
関数の動作
n
の負の値を tail()
関数に渡すと、最初の n
が除外されます (次の例を参照)。
print(df.tail(2))
を実行すると最後の 2 行が表示され、print(df.tail(-6))
を実行すると最初の 6 行を除くすべての行が表示されます。
コード例:
import pandas as pd
df = pd.DataFrame(
{
"Colours": [
"Purple",
"White",
"Black",
"Brown",
"Pink",
"Orange",
"Blue",
"Red",
"Yellow",
]
}
)
print("Complete Data Frame:")
print(df)
print("When we pass the value of `n`:")
print(df.tail(2))
print("\nWhen we pass the -ve value of `n`:")
print(df.tail(-6))
出力:
Complete Data Frame:
Colours
0 Purple
1 White
2 Black
3 Brown
4 Pink
5 Orange
6 Blue
7 Red
8 Yellow
When we pass the value of `n`:
Colours
7 Red
8 Yellow
When we pass the -ve value of `n`:
Colours
6 Blue
7 Red
8 Yellow
Python でログ ファイルを追跡する
std.log
という名前のログ ファイルを作成し、いくつかのデータを保存しました。これは、以下の出力に表示されます。 ログファイルを追跡するには、sh
モジュールから実行できます。
無限ループを実行して出力行を表示するには、ファイル名と _iter
を True
に設定して tail()
を呼び出します。
コード例:
from sh import tail
for line in tail("-f", "std.log", _iter=True):
print(line)
出力:
2022-08-25 21:44:10,045 This is just a reminder
2022-08-25 21:44:10,046 Meeting is at 2 pm
2022-08-25 21:44:10,046 After the lunch break
Python のブロッキングと非ブロッキングの Tail()
関数
ノンブロッキング tail()
関数から始めましょう。
ノンブロッキング Tail()
関数
機能がブロックされると、後続のアクティビティの完了が延期される可能性があります。 さらに、システム全体のパフォーマンスが低下する可能性があります。
言い換えれば、あなたのプログラムはブロックされ、他のものは実行できなくなります。 subprocess
を起動してその出力ストリームに接続するには、subprocess
モジュール (stdout
) を使用します。
コード例:
import subprocess
import select
import time
f = subprocess.Popen(
["tail", "-F", "log.txt"], stdout=subprocess.PIPE, stderr=subprocess.PIPE
)
p = select.poll()
p.register(f.stdout)
while True:
if p.poll(1):
print(f.stdout.readline())
time.sleep(1)
出力:
b'2022-08-25 21:44:10,045 This is just a reminder\n'
Tail()
関数のブロック
次のコードも新しい行が追加されると表示されますが、追加の select
モジュール呼び出しなしで subprocess
モジュールを利用できます。 tail プログラムが f.kill()
コマンドで終了されるまでブロックします。
コード例:
import subprocess
f = subprocess.Popen(
["tail", "-F", "log.txt"], stdout=subprocess.PIPE, stderr=subprocess.PIPE
)
while True:
line = f.stdout.readline()
print(line)
出力:
b'2022-08-25 21:44:10,045 This is just a reminder\\n'
b'2022-08-25 21:44:10,046 Meeting is at 2 pm\\n'
上記の例のログ ファイル
次のコードは log.txt
を作成するためのもので、ブロック コードと非ブロック コードで使用されます。
コード例:
import logging
logging.basicConfig(filename="log.txt", level=logging.DEBUG,
format="%(asctime)s %(message)s")
logging.debug("This is just a reminder")
logging.info("Meeting is at 2 pm")
logging.info("After the lunch break")
出力:
2022-08-25 21:44:10,045 This is just a reminder
2022-08-25 21:44:10,046 Meeting is at 2 pm
2022-08-25 21:44:10,046 After the lunch break
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn