Python でステップ関数をあてはめる
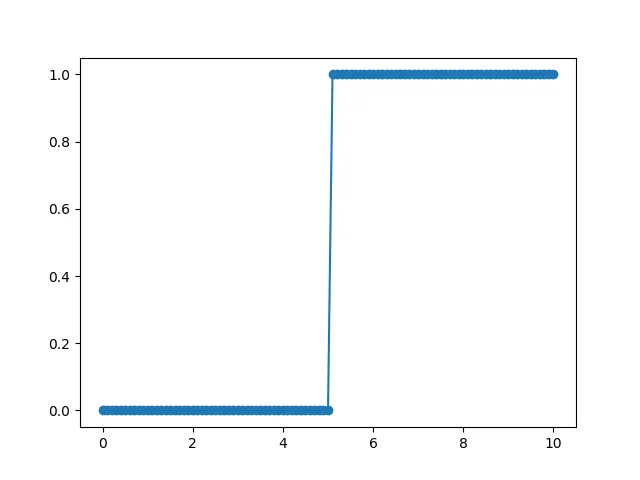
ステップ関数は、一連のステップのように見えるグラフを持つメソッドです。 それらは、間隔のある一連の水平線分で構成され、階段関数とも呼ばれます。
任意の間隔で、ステップ関数は一定の値を持ち、グラフに水平線を作成します。 間隔は、各線分の間でジャンプします。
ステップ関数は、離散プロットを生成するときに役立ち、Python でのベクトル化されたプロットで広く使用されています。 numpy
を使用して Python で実装できます。
この記事では、ステップ関数の簡単なデモを示します。
Python でステップ関数をあてはめる
この例では、Python バージョン 3.10.6 を使用します。 さらに、必要なライブラリをインストールする必要がありますが、この場合は numpy です。
Numpy は、次のコマンドを実行してインストールできます。
pip install numpy
次に、データを最適化してグラフに適合させるために scipy
が必要です。 以下のコマンドでインストールできます。
pip install scipy
この例では、データセットを使用して単純なステップ関数を生成します。 まず、次のステートメントを使用して numpy
と scipy
を環境にインポートします。
import numpy as np
import scipy
この例では、小さなデータセットを生成するために numpy linspace
メソッドを使用します。 次のコード スニペットは、このデータセットを生成できます。
x = np.linspace(0, 10, 101)
階段状のプロットを表示するには、heaviside
関数を生成する必要があります。 ユース ケースの要件に応じて、numpy またはカスタム メソッドを使用して生成できます。
関数を作成するには、この例では numpy メソッド heaviside
を使用します。
y = np.heaviside((x - 5), 0.0)
このメソッドは、次のプロットに従って heaviside
値を生成します。
0 if x1 < 0
heaviside(x1, x2) = x2 if x1 == 0
1 if x1 > 0
scipy ライブラリの curve_fit
を使用して、最適なデータ ポイントで args
を生成します。
curve_fit(sigmoid, x, y)
フローを整理して理解したので、最終的なスクリプトは次のようになります。
import numpy as np
from scipy.special import expit
from scipy.optimize import curve_fit
x = np.linspace(0, 10, 101)
y = np.heaviside((x - 5), 0.0)
def sigmoid(x, x0, b):
return expit((x - x0) * b)
args, cov = curve_fit(sigmoid, x, y)
print(args)
matplotlib
を使用して、このプロットの結果を監視できます。 プロット スニペットと最終的なコードを追加すると、次のようになります。
import matplotlib.pyplot as plt
import numpy as np
from scipy.special import expit
from scipy.optimize import curve_fit
x = np.linspace(0, 10, 101)
y = np.heaviside((x - 5), 0.0)
def sigmoid(x, x0, b):
return expit((x - x0) * b)
args, cov = curve_fit(sigmoid, x, y)
plt.scatter(x, y)
plt.plot(x, sigmoid(x, *args))
plt.show()
print(args)
出力:
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn