Kotlin の forEach ループの現在のインデックスを取得する
-
Kotlin の
forEachIndexed()
を使用して、forEach
ループ内のアイテムの現在のインデックスを取得する -
Kotlin の
withIndex()
を使用して、forEach
ループ内のアイテムの現在のインデックスを取得する -
Kotlin の
インデックス
を使用して、forEach
ループ内のアイテムの現在のインデックスを取得する -
Kotlin で
filterIndexed()
を使用して配列アイテムのインデックスを取得する
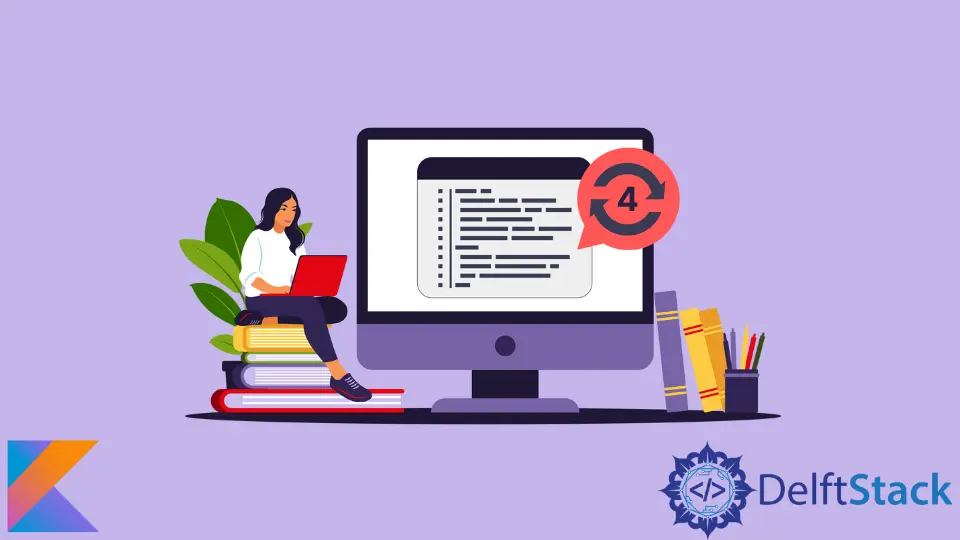
forEach
ループ内のアイテムの現在のインデックスを知ることは、見つけているアイテムの位置を見つけるのに役立ちます。この記事では、forEach
ループの現在のインデックスを見つけるためのさまざまな方法について説明します。
必要なものを取得するには、次の 3つの方法があります。
forEachIndexed()
を使用するwithIndex()
を使用するインデックス
の使用
Kotlin の forEachIndexed()
を使用して、forEach
ループ内のアイテムの現在のインデックスを取得する
forEachIndexed()
関数を使用して、現在のインデックスを取得できます。配列を入力として受け入れるインライン関数です。
forEachIndexed()
は、インデックス項目とその値を出力します。
forEachIndexed()
関数を使用するための構文は次のとおりです。
collection.forEachIndexed { index, element ->
// ...
}
それがどのように機能するかを理解するための例を見てみましょう。配列 Student
を作成し、forEachIndexed()
を使用してそれをトラバースし、以下の例の出力としてインデックスと値を取得します。
fun main() {
var Student = listOf("Virat", "David", "Steve", "Joe", "Chris")
Student.forEachIndexed {index, element ->
println("The index is $index and the item is $element ")
}
}
出力:
Kotlin の withIndex()
を使用して、forEach
ループ内のアイテムの現在のインデックスを取得する
forEachIndexed()
に加えて、withIndex()
関数を使用して、Kotlin の forEach
ループ内のアイテムの現在のインデックスを取得することもできます。
これは、ループを介してインデックスと値にアクセスできるようにするライブラリ関数です。
ここでも同じ配列を使用しますが、今回は withIndex()
関数を使用して、Student
配列のインデックスと値にアクセスします。
fun main() {
var Student = listOf("Virat", "David", "Steve", "Joe", "Chris")
for ((index, element) in Student.withIndex()) {
println("The index is $index and the item is $element ")
}
}
出力:
Kotlin のインデックス
を使用して、forEach
ループ内のアイテムの現在のインデックスを取得する
indices
キーワードを使用して、現在のインデックスを取得することもできます。インデックス
を使用するための構文は次のとおりです。
for (i in array.indices) {
print(array[i])
}
Student
配列でこの構文を使用して、インデックスと値にアクセスしてみましょう。
fun main(args : Array<String>){
val Student = arrayOf("Virat", "David", "Steve", "Joe", "Chris")
for (i in Student.indices){
println("Student[$i]: ${Student[i]}")
}
}
出力:
Kotlin で filterIndexed()
を使用して配列アイテムのインデックスを取得する
上記の関数を使用して、現在のインデックスを取得できます。しかし、すべてではなく特定のインデックスにのみアクセスしたい場合はどうでしょうか。
これは、filterIndexed()
関数を使用して行うことができます。
filterIndexed()
関数は、条件を引数として受け入れます。渡した条件に基づいて、関数は出力をフィルタリングして必要なインデックスを表示できます。
filterIndexed()
関数を使用して、Student
配列の偶数インデックスの値のみにアクセスしてみましょう。
fun main(args : Array<String>){
val Student = arrayOf("Virat", "David", "Steve", "Joe", "Chris")
.filterIndexed { index, _ -> index % 2 == 0 }
.forEach { println(it) }
}
出力:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn